3-D triangle mesh geometry. More...
#include <Core/Geometry/TriangleMesh3.h>
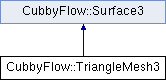
Classes | |
class | Builder |
Front-end to create TriangleMesh3 objects step by step. More... | |
Public Types | |
using | Vector2DArray = Array1< Vector2D > |
using | Vector3DArray = Array1< Vector3D > |
using | IndexArray = Array1< Point3UI > |
using | PointArray = Vector3DArray |
using | NormalArray = Vector3DArray |
using | UVArray = Vector2DArray |
Public Member Functions | |
TriangleMesh3 (const Transform3 &transform=Transform3(), bool isNormalFlipped=false) | |
Default constructor. More... | |
TriangleMesh3 (const PointArray &points, const NormalArray &normals, const UVArray &uvs, const IndexArray &pointIndices, const IndexArray &normalIndices, const IndexArray &uvIndices, const Transform3 &transform_=Transform3(), bool isNormalFlipped_=false) | |
Constructs mesh with points, normals, uvs, and their indices. More... | |
TriangleMesh3 (const TriangleMesh3 &other) | |
Copy constructor. More... | |
void | UpdateQueryEngine () override |
Updates internal spatial query engine. More... | |
void | Clear () |
Clears all content. More... | |
void | Set (const TriangleMesh3 &other) |
Copies the contents from other mesh. More... | |
void | Swap (TriangleMesh3 &other) |
Swaps the contents with other mesh. More... | |
double | Area () const |
Returns area of this mesh. More... | |
double | Volume () const |
Returns volume of this mesh. More... | |
const Vector3D & | Point (size_t i) const |
Returns constant reference to the i-th point. More... | |
Vector3D & | Point (size_t i) |
Returns reference to the i-th point. More... | |
const Vector3D & | Normal (size_t i) const |
Returns constant reference to the i-th normal. More... | |
Vector3D & | Normal (size_t i) |
Returns reference to the i-th normal. More... | |
const Vector2D & | UV (size_t i) const |
Returns constant reference to the i-th UV coordinates. More... | |
Vector2D & | UV (size_t i) |
Returns reference to the i-th UV coordinates. More... | |
const Point3UI & | PointIndex (size_t i) const |
Returns constant reference to the point indices of i-th triangle. More... | |
Point3UI & | PointIndex (size_t i) |
Returns reference to the point indices of i-th triangle. More... | |
const Point3UI & | NormalIndex (size_t i) const |
Returns constant reference to the normal indices of i-th triangle. More... | |
Point3UI & | NormalIndex (size_t i) |
Returns reference to the normal indices of i-th triangle. More... | |
const Point3UI & | UVIndex (size_t i) const |
Returns constant reference to the UV indices of i-th triangle. More... | |
Point3UI & | UVIndex (size_t i) |
Returns reference to the UV indices of i-th triangle. More... | |
Triangle3 | Triangle (size_t i) const |
Returns i-th triangle. More... | |
size_t | NumberOfPoints () const |
Returns number of points. More... | |
size_t | NumberOfNormals () const |
Returns number of normals. More... | |
size_t | NumberOfUVs () const |
Returns number of UV coordinates. More... | |
size_t | NumberOfTriangles () const |
Returns number of triangles. More... | |
bool | HasNormals () const |
Returns true if the mesh has normals. More... | |
bool | HasUVs () const |
Returns true if the mesh has UV coordinates. More... | |
void | AddPoint (const Vector3D &pt) |
Adds a point. More... | |
void | AddNormal (const Vector3D &n) |
Adds a normal. More... | |
void | AddUV (const Vector2D &t) |
Adds a UV. More... | |
void | AddPointTriangle (const Point3UI &newPointIndices) |
Adds a triangle with points. More... | |
void | AddPointNormalTriangle (const Point3UI &newPointIndices, const Point3UI &newNormalIndices) |
Adds a triangle with point and normal. More... | |
void | AddPointUVNormalTriangle (const Point3UI &newPointIndices, const Point3UI &newUVIndices, const Point3UI &newNormalIndices) |
Adds a triangle with point, normal, and UV. More... | |
void | AddPointUVTriangle (const Point3UI &newPointIndices, const Point3UI &newUVIndices) |
Adds a triangle with point and UV. More... | |
void | AddTriangle (const Triangle3 &tri) |
Add a triangle. More... | |
void | SetFaceNormal () |
Sets entire normals to the face normals. More... | |
void | SetAngleWeightedVertexNormal () |
Sets angle weighted vertex normal. More... | |
void | Scale (double factor) |
Scales the mesh by given factor. More... | |
void | Translate (const Vector3D &t) |
Translates the mesh. More... | |
void | Rotate (const QuaternionD &q) |
Rotates the mesh. More... | |
void | WriteObj (std::ostream *stream) const |
Writes the mesh in obj format to the output stream. More... | |
bool | WriteObj (const std::string &fileName) const |
Writes the mesh in obj format to the file. More... | |
bool | ReadObj (std::istream *stream) |
Reads the mesh in obj format from the input stream. More... | |
bool | ReadObj (const std::string &fileName) |
Reads the mesh in obj format from the file. More... | |
TriangleMesh3 & | operator= (const TriangleMesh3 &other) |
Copies other mesh. More... | |
![]() | |
Surface3 (const Transform3 &transform=Transform3(), bool isNormalFlipped=false) | |
Constructs a surface with normal direction. More... | |
Surface3 (const Surface3 &other) | |
Copy constructor. More... | |
virtual | ~Surface3 () |
Default destructor. More... | |
Vector3D | ClosestPoint (const Vector3D &otherPoint) const |
Returns the closest point from the given point otherPoint to the surface. More... | |
BoundingBox3D | BoundingBox () const |
Returns the bounding box of this surface object. More... | |
bool | Intersects (const Ray3D &ray) const |
Returns true if the given ray intersects with this surface object. More... | |
double | ClosestDistance (const Vector3D &otherPoint) const |
SurfaceRayIntersection3 | ClosestIntersection (const Ray3D &ray) const |
Returns the closest intersection point for given ray . More... | |
Vector3D | ClosestNormal (const Vector3D &otherPoint) const |
Static Public Member Functions | |
static Builder | GetBuilder () |
Returns builder fox TriangleMesh3. More... | |
Protected Member Functions | |
Vector3D | ClosestPointLocal (const Vector3D &otherPoint) const override |
double | ClosestDistanceLocal (const Vector3D &otherPoint) const override |
bool | IntersectsLocal (const Ray3D &ray) const override |
BoundingBox3D | BoundingBoxLocal () const override |
Returns the bounding box of this surface object in local frame. More... | |
Vector3D | ClosestNormalLocal (const Vector3D &otherPoint) const override |
SurfaceRayIntersection3 | ClosestIntersectionLocal (const Ray3D &ray) const override |
Returns the closest intersection point for given ray in local frame. More... | |
Additional Inherited Members | |
![]() | |
Transform3 | transform |
Local-to-world transform. More... | |
bool | isNormalFlipped = false |
Flips normal when calling Surface3::closestNormal(...). More... | |
Detailed Description
3-D triangle mesh geometry.
This class represents 3-D triangle mesh geometry which extends Surface3 by overriding surface-related queries. The mesh structure stores point, normals, and UV coordinates.
Member Typedef Documentation
◆ IndexArray
◆ NormalArray
◆ PointArray
◆ UVArray
◆ Vector2DArray
◆ Vector3DArray
Constructor & Destructor Documentation
◆ TriangleMesh3() [1/3]
CubbyFlow::TriangleMesh3::TriangleMesh3 | ( | const Transform3 & | transform = Transform3() , |
bool | isNormalFlipped = false |
||
) |
Default constructor.
◆ TriangleMesh3() [2/3]
CubbyFlow::TriangleMesh3::TriangleMesh3 | ( | const PointArray & | points, |
const NormalArray & | normals, | ||
const UVArray & | uvs, | ||
const IndexArray & | pointIndices, | ||
const IndexArray & | normalIndices, | ||
const IndexArray & | uvIndices, | ||
const Transform3 & | transform_ = Transform3() , |
||
bool | isNormalFlipped_ = false |
||
) |
Constructs mesh with points, normals, uvs, and their indices.
◆ TriangleMesh3() [3/3]
CubbyFlow::TriangleMesh3::TriangleMesh3 | ( | const TriangleMesh3 & | other | ) |
Copy constructor.
Member Function Documentation
◆ AddNormal()
void CubbyFlow::TriangleMesh3::AddNormal | ( | const Vector3D & | n | ) |
Adds a normal.
◆ AddPoint()
void CubbyFlow::TriangleMesh3::AddPoint | ( | const Vector3D & | pt | ) |
Adds a point.
◆ AddPointNormalTriangle()
void CubbyFlow::TriangleMesh3::AddPointNormalTriangle | ( | const Point3UI & | newPointIndices, |
const Point3UI & | newNormalIndices | ||
) |
Adds a triangle with point and normal.
◆ AddPointTriangle()
void CubbyFlow::TriangleMesh3::AddPointTriangle | ( | const Point3UI & | newPointIndices | ) |
Adds a triangle with points.
◆ AddPointUVNormalTriangle()
void CubbyFlow::TriangleMesh3::AddPointUVNormalTriangle | ( | const Point3UI & | newPointIndices, |
const Point3UI & | newUVIndices, | ||
const Point3UI & | newNormalIndices | ||
) |
Adds a triangle with point, normal, and UV.
◆ AddPointUVTriangle()
void CubbyFlow::TriangleMesh3::AddPointUVTriangle | ( | const Point3UI & | newPointIndices, |
const Point3UI & | newUVIndices | ||
) |
Adds a triangle with point and UV.
◆ AddTriangle()
void CubbyFlow::TriangleMesh3::AddTriangle | ( | const Triangle3 & | tri | ) |
Add a triangle.
◆ AddUV()
void CubbyFlow::TriangleMesh3::AddUV | ( | const Vector2D & | t | ) |
Adds a UV.
◆ Area()
double CubbyFlow::TriangleMesh3::Area | ( | ) | const |
Returns area of this mesh.
◆ BoundingBoxLocal()
|
overrideprotectedvirtual |
Returns the bounding box of this surface object in local frame.
Implements CubbyFlow::Surface3.
◆ Clear()
void CubbyFlow::TriangleMesh3::Clear | ( | ) |
Clears all content.
◆ ClosestDistanceLocal()
|
overrideprotectedvirtual |
Returns the closest distance from the given point otherPoint
to the point on the surface in local frame.
Reimplemented from CubbyFlow::Surface3.
◆ ClosestIntersectionLocal()
|
overrideprotectedvirtual |
Returns the closest intersection point for given ray
in local frame.
Implements CubbyFlow::Surface3.
◆ ClosestNormalLocal()
|
overrideprotectedvirtual |
Returns the normal to the closest point on the surface from the given point otherPoint
in local frame.
Implements CubbyFlow::Surface3.
◆ ClosestPointLocal()
|
overrideprotectedvirtual |
Returns the closest point from the given point otherPoint
to the surface in local frame.
Implements CubbyFlow::Surface3.
◆ GetBuilder()
|
static |
Returns builder fox TriangleMesh3.
◆ HasNormals()
bool CubbyFlow::TriangleMesh3::HasNormals | ( | ) | const |
Returns true if the mesh has normals.
◆ HasUVs()
bool CubbyFlow::TriangleMesh3::HasUVs | ( | ) | const |
Returns true if the mesh has UV coordinates.
◆ IntersectsLocal()
|
overrideprotectedvirtual |
Returns true if the given ray
intersects with this surface object in local frame.
Reimplemented from CubbyFlow::Surface3.
◆ Normal() [1/2]
const Vector3D& CubbyFlow::TriangleMesh3::Normal | ( | size_t | i | ) | const |
Returns constant reference to the i-th normal.
◆ Normal() [2/2]
Vector3D& CubbyFlow::TriangleMesh3::Normal | ( | size_t | i | ) |
Returns reference to the i-th normal.
◆ NormalIndex() [1/2]
const Point3UI& CubbyFlow::TriangleMesh3::NormalIndex | ( | size_t | i | ) | const |
Returns constant reference to the normal indices of i-th triangle.
◆ NormalIndex() [2/2]
Point3UI& CubbyFlow::TriangleMesh3::NormalIndex | ( | size_t | i | ) |
Returns reference to the normal indices of i-th triangle.
◆ NumberOfNormals()
size_t CubbyFlow::TriangleMesh3::NumberOfNormals | ( | ) | const |
Returns number of normals.
◆ NumberOfPoints()
size_t CubbyFlow::TriangleMesh3::NumberOfPoints | ( | ) | const |
Returns number of points.
◆ NumberOfTriangles()
size_t CubbyFlow::TriangleMesh3::NumberOfTriangles | ( | ) | const |
Returns number of triangles.
◆ NumberOfUVs()
size_t CubbyFlow::TriangleMesh3::NumberOfUVs | ( | ) | const |
Returns number of UV coordinates.
◆ operator=()
TriangleMesh3& CubbyFlow::TriangleMesh3::operator= | ( | const TriangleMesh3 & | other | ) |
Copies other
mesh.
◆ Point() [1/2]
const Vector3D& CubbyFlow::TriangleMesh3::Point | ( | size_t | i | ) | const |
Returns constant reference to the i-th point.
◆ Point() [2/2]
Vector3D& CubbyFlow::TriangleMesh3::Point | ( | size_t | i | ) |
Returns reference to the i-th point.
◆ PointIndex() [1/2]
const Point3UI& CubbyFlow::TriangleMesh3::PointIndex | ( | size_t | i | ) | const |
Returns constant reference to the point indices of i-th triangle.
◆ PointIndex() [2/2]
Point3UI& CubbyFlow::TriangleMesh3::PointIndex | ( | size_t | i | ) |
Returns reference to the point indices of i-th triangle.
◆ ReadObj() [1/2]
bool CubbyFlow::TriangleMesh3::ReadObj | ( | std::istream * | stream | ) |
Reads the mesh in obj format from the input stream.
◆ ReadObj() [2/2]
bool CubbyFlow::TriangleMesh3::ReadObj | ( | const std::string & | fileName | ) |
Reads the mesh in obj format from the file.
◆ Rotate()
void CubbyFlow::TriangleMesh3::Rotate | ( | const QuaternionD & | q | ) |
Rotates the mesh.
◆ Scale()
void CubbyFlow::TriangleMesh3::Scale | ( | double | factor | ) |
Scales the mesh by given factor.
◆ Set()
void CubbyFlow::TriangleMesh3::Set | ( | const TriangleMesh3 & | other | ) |
Copies the contents from other
mesh.
◆ SetAngleWeightedVertexNormal()
void CubbyFlow::TriangleMesh3::SetAngleWeightedVertexNormal | ( | ) |
Sets angle weighted vertex normal.
◆ SetFaceNormal()
void CubbyFlow::TriangleMesh3::SetFaceNormal | ( | ) |
Sets entire normals to the face normals.
◆ Swap()
void CubbyFlow::TriangleMesh3::Swap | ( | TriangleMesh3 & | other | ) |
Swaps the contents with other
mesh.
◆ Translate()
void CubbyFlow::TriangleMesh3::Translate | ( | const Vector3D & | t | ) |
Translates the mesh.
◆ Triangle()
Triangle3 CubbyFlow::TriangleMesh3::Triangle | ( | size_t | i | ) | const |
Returns i-th triangle.
◆ UpdateQueryEngine()
|
overridevirtual |
Updates internal spatial query engine.
Reimplemented from CubbyFlow::Surface3.
◆ UV() [1/2]
const Vector2D& CubbyFlow::TriangleMesh3::UV | ( | size_t | i | ) | const |
Returns constant reference to the i-th UV coordinates.
◆ UV() [2/2]
Vector2D& CubbyFlow::TriangleMesh3::UV | ( | size_t | i | ) |
Returns reference to the i-th UV coordinates.
◆ UVIndex() [1/2]
const Point3UI& CubbyFlow::TriangleMesh3::UVIndex | ( | size_t | i | ) | const |
Returns constant reference to the UV indices of i-th triangle.
◆ UVIndex() [2/2]
Point3UI& CubbyFlow::TriangleMesh3::UVIndex | ( | size_t | i | ) |
Returns reference to the UV indices of i-th triangle.
◆ Volume()
double CubbyFlow::TriangleMesh3::Volume | ( | ) | const |
Returns volume of this mesh.
◆ WriteObj() [1/2]
void CubbyFlow::TriangleMesh3::WriteObj | ( | std::ostream * | stream | ) | const |
Writes the mesh in obj format to the output stream.
◆ WriteObj() [2/2]
bool CubbyFlow::TriangleMesh3::WriteObj | ( | const std::string & | fileName | ) | const |
Writes the mesh in obj format to the file.
The documentation for this class was generated from the following file:
- Core/Geometry/TriangleMesh3.h