3-D triangle geometry. More...
#include <Core/Geometry/Triangle3.h>
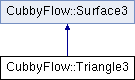
Classes | |
class | Builder |
Front-end to create Triangle3 objects step by step. More... | |
Public Member Functions | |
Triangle3 (const Transform3 &transform=Transform3(), bool isNormalFlipped=false) | |
Constructs an empty triangle. More... | |
Triangle3 (const std::array< Vector3D, 3 > &points, const std::array< Vector3D, 3 > &normals, const std::array< Vector2D, 3 > &uvs, const Transform3 &transform=Transform3(), bool isNormalFlipped=false) | |
Constructs a triangle with given points , normals , and uvs . More... | |
Triangle3 (const Triangle3 &other) | |
Copy constructor. More... | |
double | Area () const |
Returns the area of this triangle. More... | |
void | GetBarycentricCoords (const Vector3D &pt, double *b0, double *b1, double *b2) const |
Returns barycentric coordinates for the given point pt . More... | |
Vector3D | FaceNormal () const |
Returns the face normal of the triangle. More... | |
void | SetNormalsToFaceNormal () |
Set Triangle3::normals to the face normal. More... | |
![]() | |
Surface3 (const Transform3 &transform=Transform3(), bool isNormalFlipped=false) | |
Constructs a surface with normal direction. More... | |
Surface3 (const Surface3 &other) | |
Copy constructor. More... | |
virtual | ~Surface3 () |
Default destructor. More... | |
Vector3D | ClosestPoint (const Vector3D &otherPoint) const |
Returns the closest point from the given point otherPoint to the surface. More... | |
BoundingBox3D | BoundingBox () const |
Returns the bounding box of this surface object. More... | |
bool | Intersects (const Ray3D &ray) const |
Returns true if the given ray intersects with this surface object. More... | |
double | ClosestDistance (const Vector3D &otherPoint) const |
SurfaceRayIntersection3 | ClosestIntersection (const Ray3D &ray) const |
Returns the closest intersection point for given ray . More... | |
Vector3D | ClosestNormal (const Vector3D &otherPoint) const |
virtual void | UpdateQueryEngine () |
Updates internal spatial query engine. More... | |
Static Public Member Functions | |
static Builder | GetBuilder () |
Returns builder fox Triangle3. More... | |
Public Attributes | |
std::array< Vector3D, 3 > | points |
Three points. More... | |
std::array< Vector3D, 3 > | normals |
Three normals. More... | |
std::array< Vector2D, 3 > | uvs |
Three UV coordinates. More... | |
![]() | |
Transform3 | transform |
Local-to-world transform. More... | |
bool | isNormalFlipped = false |
Flips normal when calling Surface3::closestNormal(...). More... | |
Protected Member Functions | |
Vector3D | ClosestPointLocal (const Vector3D &otherPoint) const override |
bool | IntersectsLocal (const Ray3D &ray) const override |
BoundingBox3D | BoundingBoxLocal () const override |
Returns the bounding box of this surface object in local frame. More... | |
Vector3D | ClosestNormalLocal (const Vector3D &otherPoint) const override |
SurfaceRayIntersection3 | ClosestIntersectionLocal (const Ray3D &ray) const override |
Returns the closest intersection point for given ray in local frame. More... | |
![]() | |
virtual double | ClosestDistanceLocal (const Vector3D &otherPoint) const |
Detailed Description
3-D triangle geometry.
This class represents 3-D triangle geometry which extends Surface3 by overriding surface-related queries.
Constructor & Destructor Documentation
◆ Triangle3() [1/3]
CubbyFlow::Triangle3::Triangle3 | ( | const Transform3 & | transform = Transform3() , |
bool | isNormalFlipped = false |
||
) |
Constructs an empty triangle.
◆ Triangle3() [2/3]
CubbyFlow::Triangle3::Triangle3 | ( | const std::array< Vector3D, 3 > & | points, |
const std::array< Vector3D, 3 > & | normals, | ||
const std::array< Vector2D, 3 > & | uvs, | ||
const Transform3 & | transform = Transform3() , |
||
bool | isNormalFlipped = false |
||
) |
Constructs a triangle with given points
, normals
, and uvs
.
◆ Triangle3() [3/3]
CubbyFlow::Triangle3::Triangle3 | ( | const Triangle3 & | other | ) |
Copy constructor.
Member Function Documentation
◆ Area()
double CubbyFlow::Triangle3::Area | ( | ) | const |
Returns the area of this triangle.
◆ BoundingBoxLocal()
|
overrideprotectedvirtual |
Returns the bounding box of this surface object in local frame.
Implements CubbyFlow::Surface3.
◆ ClosestIntersectionLocal()
|
overrideprotectedvirtual |
Returns the closest intersection point for given ray
in local frame.
Implements CubbyFlow::Surface3.
◆ ClosestNormalLocal()
|
overrideprotectedvirtual |
Returns the normal to the closest point on the surface from the given point otherPoint
in local frame.
Implements CubbyFlow::Surface3.
◆ ClosestPointLocal()
|
overrideprotectedvirtual |
Returns the closest point from the given point otherPoint
to the surface in local frame.
Implements CubbyFlow::Surface3.
◆ FaceNormal()
Vector3D CubbyFlow::Triangle3::FaceNormal | ( | ) | const |
Returns the face normal of the triangle.
◆ GetBarycentricCoords()
void CubbyFlow::Triangle3::GetBarycentricCoords | ( | const Vector3D & | pt, |
double * | b0, | ||
double * | b1, | ||
double * | b2 | ||
) | const |
Returns barycentric coordinates for the given point pt
.
◆ GetBuilder()
◆ IntersectsLocal()
|
overrideprotectedvirtual |
Returns true if the given ray
intersects with this surface object in local frame.
Reimplemented from CubbyFlow::Surface3.
◆ SetNormalsToFaceNormal()
void CubbyFlow::Triangle3::SetNormalsToFaceNormal | ( | ) |
Set Triangle3::normals to the face normal.
Member Data Documentation
◆ normals
std::array<Vector3D, 3> CubbyFlow::Triangle3::normals |
Three normals.
◆ points
std::array<Vector3D, 3> CubbyFlow::Triangle3::points |
Three points.
◆ uvs
std::array<Vector2D, 3> CubbyFlow::Triangle3::uvs |
Three UV coordinates.
The documentation for this class was generated from the following file:
- Core/Geometry/Triangle3.h