Implementation of 3-D semi-Lagrangian advection solver. More...
#include <Core/SemiLagrangian/SemiLagrangian3.h>
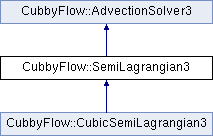
Public Member Functions | |
SemiLagrangian3 () | |
virtual | ~SemiLagrangian3 () |
void | Advect (const ScalarGrid3 &input, const VectorField3 &flow, double dt, ScalarGrid3 *output, const ScalarField3 &boundarySDF=ConstantScalarField3(std::numeric_limits< double >::max())) final |
Computes semi-Lagrangian for given scalar grid. More... | |
void | Advect (const CollocatedVectorGrid3 &input, const VectorField3 &flow, double dt, CollocatedVectorGrid3 *output, const ScalarField3 &boundarySDF=ConstantScalarField3(std::numeric_limits< double >::max())) final |
Computes semi-Lagrangian for given collocated vector grid. More... | |
void | Advect (const FaceCenteredGrid3 &input, const VectorField3 &flow, double dt, FaceCenteredGrid3 *output, const ScalarField3 &boundarySDF=ConstantScalarField3(std::numeric_limits< double >::max())) final |
Computes semi-Lagrangian for given face-centered vector grid. More... | |
![]() | |
AdvectionSolver3 () | |
virtual | ~AdvectionSolver3 () |
Protected Member Functions | |
virtual std::function< double(const Vector3D &)> | GetScalarSamplerFunc (const ScalarGrid3 &input) const |
Returns spatial interpolation function object for given scalar grid. More... | |
virtual std::function< Vector3D(const Vector3D &)> | GetVectorSamplerFunc (const CollocatedVectorGrid3 &input) const |
Returns spatial interpolation function object for given collocated vector grid. More... | |
virtual std::function< Vector3D(const Vector3D &)> | GetVectorSamplerFunc (const FaceCenteredGrid3 &input) const |
Returns spatial interpolation function object for given face-centered vector grid. More... | |
Detailed Description
Implementation of 3-D semi-Lagrangian advection solver.
This class implements 3-D semi-Lagrangian advection solver. By default, the class implements 1st-order (linear) algorithm for the spatial interpolation. For the back-tracing, this class uses 2nd-order mid-point rule with adaptive time-stepping (CFL <= 1). To extend the class using higher-order spatial interpolation, the inheriting classes can override SemiLagrangian2::getScalarSamplerFunc and SemiLagrangian2::getVectorSamplerFunc. See CubicSemiLagrangian2 for example.
Constructor & Destructor Documentation
◆ SemiLagrangian3()
CubbyFlow::SemiLagrangian3::SemiLagrangian3 | ( | ) |
◆ ~SemiLagrangian3()
|
virtual |
Member Function Documentation
◆ Advect() [1/3]
|
finalvirtual |
Computes semi-Lagrangian for given scalar grid.
This function computes semi-Lagrangian method to solve advection equation for given scalar field input
and underlying vector field flow
that carries the input field. The solution after solving the equation for given time-step dt
should be stored in scalar field output
. The boundary interface is given by a signed-distance field. The field is negative inside the boundary. By default, a constant field with max double value (std::numeric_limits<double>::max()) is used, meaning no boundary.
- Parameters
-
input Input scalar grid. flow Vector field that advects the input field. dt Time-step for the advection. output Output scalar grid. boundarySDF Boundary interface defined by signed-distance field.
Implements CubbyFlow::AdvectionSolver3.
◆ Advect() [2/3]
|
finalvirtual |
Computes semi-Lagrangian for given collocated vector grid.
This function computes semi-Lagrangian method to solve advection equation for given collocated vector grid input
and underlying vector field flow
that carries the input field. The solution after solving the equation for given time-step dt
should be stored in scalar field output
. The boundary interface is given by a signed-distance field. The field is negative inside the boundary. By default, a constant field with max double value (std::numeric_limits<double>::max()) is used, meaning no boundary.
- Parameters
-
input Input vector grid. flow Vector field that advects the input field. dt Time-step for the advection. output Output vector grid. boundarySDF Boundary interface defined by signed-distance field.
Reimplemented from CubbyFlow::AdvectionSolver3.
◆ Advect() [3/3]
|
finalvirtual |
Computes semi-Lagrangian for given face-centered vector grid.
This function computes semi-Lagrangian method to solve advection equation for given face-centered vector grid input
and underlying vector field flow
that carries the input field. The solution after solving the equation for given time-step dt
should be stored in vector field output
. The boundary interface is given by a signed-distance field. The field is negative inside the boundary. By default, a constant field with max double value (std::numeric_limits<double>::max()) is used, meaning no boundary.
- Parameters
-
input Input vector grid. flow Vector field that advects the input field. dt Time-step for the advection. output Output vector grid. boundarySDF Boundary interface defined by signed-distance field.
Reimplemented from CubbyFlow::AdvectionSolver3.
◆ GetScalarSamplerFunc()
|
protectedvirtual |
Returns spatial interpolation function object for given scalar grid.
This function returns spatial interpolation function (sampler) for given scalar grid input
. By default, this function returns linear interpolation function. Override this function to have custom interpolation for semi-Lagrangian process.
Reimplemented in CubbyFlow::CubicSemiLagrangian3.
◆ GetVectorSamplerFunc() [1/2]
|
protectedvirtual |
Returns spatial interpolation function object for given collocated vector grid.
This function returns spatial interpolation function (sampler) for given collocated vector grid input
. By default, this function returns linear interpolation function. Override this function to have custom interpolation for semi-Lagrangian process.
Reimplemented in CubbyFlow::CubicSemiLagrangian3.
◆ GetVectorSamplerFunc() [2/2]
|
protectedvirtual |
Returns spatial interpolation function object for given face-centered vector grid.
This function returns spatial interpolation function (sampler) for given face-centered vector grid input
. By default, this function returns linear interpolation function. Override this function to have custom interpolation for semi-Lagrangian process.
Reimplemented in CubbyFlow::CubicSemiLagrangian3.
The documentation for this class was generated from the following file:
- Core/SemiLagrangian/SemiLagrangian3.h