Abstract base class for 3-D scalar grid structure. More...
#include <Core/Grid/ScalarGrid3.h>
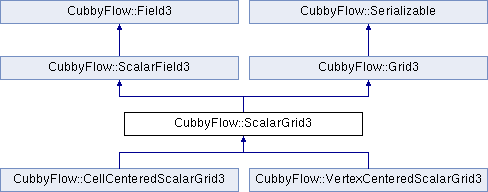
Public Types | |
using | ScalarDataAccessor = ArrayAccessor3< double > |
Read-write array accessor type. More... | |
using | ConstScalarDataAccessor = ConstArrayAccessor3< double > |
Read-only array accessor type. More... | |
![]() | |
using | DataPositionFunc = std::function< Vector3D(size_t, size_t, size_t)> |
Function type for mapping data index to actual position. More... | |
Public Member Functions | |
ScalarGrid3 () | |
Constructs an empty grid. More... | |
virtual | ~ScalarGrid3 () |
Default destructor. More... | |
virtual Size3 | GetDataSize () const =0 |
Returns the size of the grid data. More... | |
virtual Vector3D | GetDataOrigin () const =0 |
Returns the origin of the grid data. More... | |
virtual std::shared_ptr< ScalarGrid3 > | Clone () const =0 |
Returns the copy of the grid instance. More... | |
void | Clear () |
Clears the contents of the grid. More... | |
void | Resize (size_t resolutionX, size_t resolutionY, size_t resolutionZ, double gridSpacingX=1.0, double gridSpacingY=1.0, double gridSpacingZ=1.0, double originX=0.0, double originY=0.0, double originZ=0.0, double initialValue=0.0) |
Resizes the grid using given parameters. More... | |
void | Resize (const Size3 &resolution, const Vector3D &gridSpacing=Vector3D(1, 1, 1), const Vector3D &origin=Vector3D(), double initialValue=0.0) |
Resizes the grid using given parameters. More... | |
void | Resize (double gridSpacingX, double gridSpacingY, double gridSpacingZ, double originX, double originY, double originZ) |
Resizes the grid using given parameters. More... | |
void | Resize (const Vector3D &gridSpacing, const Vector3D &origin) |
Resizes the grid using given parameters. More... | |
const double & | operator() (size_t i, size_t j, size_t k) const |
Returns the grid data at given data point. More... | |
double & | operator() (size_t i, size_t j, size_t k) |
Returns the grid data at given data point. More... | |
Vector3D | GradientAtDataPoint (size_t i, size_t j, size_t k) const |
Returns the gradient vector at given data point. More... | |
double | LaplacianAtDataPoint (size_t i, size_t j, size_t k) const |
Returns the Laplacian at given data point. More... | |
ScalarDataAccessor | GetDataAccessor () |
Returns the read-write data array accessor. More... | |
ConstScalarDataAccessor | GetConstDataAccessor () const |
Returns the read-only data array accessor. More... | |
DataPositionFunc | GetDataPosition () const |
Returns the function that maps data point to its position. More... | |
void | Fill (double value, ExecutionPolicy policy=ExecutionPolicy::Parallel) |
Fills the grid with given value. More... | |
void | Fill (const std::function< double(const Vector3D &)> &func, ExecutionPolicy policy=ExecutionPolicy::Serial) |
Fills the grid with given position-to-value mapping function. More... | |
void | ForEachDataPointIndex (const std::function< void(size_t, size_t, size_t)> &func) const |
Invokes the given function func for each data point. More... | |
void | ParallelForEachDataPointIndex (const std::function< void(size_t, size_t, size_t)> &func) const |
Invokes the given function func for each data point in parallel. More... | |
double | Sample (const Vector3D &x) const override |
Returns the sampled value at given position x . More... | |
std::function< double(const Vector3D &)> | Sampler () const override |
Returns the sampler function. More... | |
Vector3D | Gradient (const Vector3D &x) const override |
Returns the gradient vector at given position x . More... | |
double | Laplacian (const Vector3D &x) const override |
Returns the Laplacian at given position x . More... | |
void | Serialize (std::vector< uint8_t > *buffer) const override |
Serializes the grid instance to the output buffer. More... | |
void | Deserialize (const std::vector< uint8_t > &buffer) override |
Deserializes the input buffer to the grid instance. More... | |
![]() | |
ScalarField3 () | |
Default constructor. More... | |
virtual | ~ScalarField3 () |
Default destructor. More... | |
![]() | |
Field3 () | |
virtual | ~Field3 () |
![]() | |
Grid3 () | |
Constructs an empty grid. More... | |
virtual | ~Grid3 () |
Default destructor. More... | |
virtual std::string | TypeName () const =0 |
Returns the type name of derived grid. More... | |
const Size3 & | Resolution () const |
Returns the grid resolution. More... | |
const Vector3D & | Origin () const |
Returns the grid origin. More... | |
const Vector3D & | GridSpacing () const |
Returns the grid spacing. More... | |
const BoundingBox3D & | BoundingBox () const |
Returns the bounding box of the grid. More... | |
DataPositionFunc | CellCenterPosition () const |
Returns the function that maps grid index to the cell-center position. More... | |
void | ForEachCellIndex (const std::function< void(size_t, size_t, size_t)> &func) const |
Invokes the given function func for each grid cell. More... | |
void | ParallelForEachCellIndex (const std::function< void(size_t, size_t, size_t)> &func) const |
Invokes the given function func for each grid cell in parallel. More... | |
bool | HasSameShape (const Grid3 &other) const |
Returns true if resolution, grid-spacing and origin are same. More... | |
virtual void | Swap (Grid3 *other)=0 |
Swaps the data with other grid. More... | |
Protected Member Functions | |
void | SwapScalarGrid (ScalarGrid3 *other) |
Swaps the data storage and predefined samplers with given grid. More... | |
void | SetScalarGrid (const ScalarGrid3 &other) |
Sets the data storage and predefined samplers with given grid. More... | |
void | GetData (std::vector< double > *data) const override |
Fetches the data into a continuous linear array. More... | |
void | SetData (const std::vector< double > &data) override |
Sets the data from a continuous linear array. More... | |
![]() | |
void | SetSizeParameters (const Size3 &resolution, const Vector3D &gridSpacing, const Vector3D &origin) |
Sets the size parameters including the resolution, grid spacing, and origin. More... | |
void | SwapGrid (Grid3 *other) |
Swaps the size parameters with given grid other . More... | |
void | SetGrid (const Grid3 &other) |
Sets the size parameters with given grid other . More... | |
Detailed Description
Abstract base class for 3-D scalar grid structure.
Member Typedef Documentation
◆ ConstScalarDataAccessor
using CubbyFlow::ScalarGrid3::ConstScalarDataAccessor = ConstArrayAccessor3<double> |
Read-only array accessor type.
◆ ScalarDataAccessor
using CubbyFlow::ScalarGrid3::ScalarDataAccessor = ArrayAccessor3<double> |
Read-write array accessor type.
Constructor & Destructor Documentation
◆ ScalarGrid3()
CubbyFlow::ScalarGrid3::ScalarGrid3 | ( | ) |
Constructs an empty grid.
◆ ~ScalarGrid3()
|
virtual |
Default destructor.
Member Function Documentation
◆ Clear()
void CubbyFlow::ScalarGrid3::Clear | ( | ) |
Clears the contents of the grid.
◆ Clone()
|
pure virtual |
Returns the copy of the grid instance.
Implemented in CubbyFlow::CellCenteredScalarGrid3, and CubbyFlow::VertexCenteredScalarGrid3.
◆ Deserialize()
|
overridevirtual |
Deserializes the input buffer to the grid instance.
Implements CubbyFlow::Grid3.
◆ Fill() [1/2]
void CubbyFlow::ScalarGrid3::Fill | ( | double | value, |
ExecutionPolicy | policy = ExecutionPolicy::Parallel |
||
) |
Fills the grid with given value.
◆ Fill() [2/2]
void CubbyFlow::ScalarGrid3::Fill | ( | const std::function< double(const Vector3D &)> & | func, |
ExecutionPolicy | policy = ExecutionPolicy::Serial |
||
) |
Fills the grid with given position-to-value mapping function.
◆ ForEachDataPointIndex()
void CubbyFlow::ScalarGrid3::ForEachDataPointIndex | ( | const std::function< void(size_t, size_t, size_t)> & | func | ) | const |
Invokes the given function func
for each data point.
This function invokes the given function object func
for each data point in serial manner. The input parameters are i and j indices of a data point. The order of execution is i-first, j-last.
◆ GetConstDataAccessor()
ConstScalarDataAccessor CubbyFlow::ScalarGrid3::GetConstDataAccessor | ( | ) | const |
Returns the read-only data array accessor.
◆ GetData()
|
overrideprotectedvirtual |
Fetches the data into a continuous linear array.
Implements CubbyFlow::Grid3.
◆ GetDataAccessor()
ScalarDataAccessor CubbyFlow::ScalarGrid3::GetDataAccessor | ( | ) |
Returns the read-write data array accessor.
◆ GetDataOrigin()
|
pure virtual |
Returns the origin of the grid data.
This function returns data position for the grid point at (0, 0, 0). Note that this is different from origin() since origin() returns the lower corner point of the bounding box.
Implemented in CubbyFlow::CellCenteredScalarGrid3, and CubbyFlow::VertexCenteredScalarGrid3.
◆ GetDataPosition()
DataPositionFunc CubbyFlow::ScalarGrid3::GetDataPosition | ( | ) | const |
Returns the function that maps data point to its position.
◆ GetDataSize()
|
pure virtual |
Returns the size of the grid data.
This function returns the size of the grid data which is not necessarily equal to the grid resolution if the data is not stored at cell-center.
Implemented in CubbyFlow::CellCenteredScalarGrid3, and CubbyFlow::VertexCenteredScalarGrid3.
◆ Gradient()
Returns the gradient vector at given position x
.
Reimplemented from CubbyFlow::ScalarField3.
◆ GradientAtDataPoint()
Vector3D CubbyFlow::ScalarGrid3::GradientAtDataPoint | ( | size_t | i, |
size_t | j, | ||
size_t | k | ||
) | const |
Returns the gradient vector at given data point.
◆ Laplacian()
|
overridevirtual |
Returns the Laplacian at given position x
.
Reimplemented from CubbyFlow::ScalarField3.
◆ LaplacianAtDataPoint()
double CubbyFlow::ScalarGrid3::LaplacianAtDataPoint | ( | size_t | i, |
size_t | j, | ||
size_t | k | ||
) | const |
Returns the Laplacian at given data point.
◆ operator()() [1/2]
const double& CubbyFlow::ScalarGrid3::operator() | ( | size_t | i, |
size_t | j, | ||
size_t | k | ||
) | const |
Returns the grid data at given data point.
◆ operator()() [2/2]
double& CubbyFlow::ScalarGrid3::operator() | ( | size_t | i, |
size_t | j, | ||
size_t | k | ||
) |
Returns the grid data at given data point.
◆ ParallelForEachDataPointIndex()
void CubbyFlow::ScalarGrid3::ParallelForEachDataPointIndex | ( | const std::function< void(size_t, size_t, size_t)> & | func | ) | const |
Invokes the given function func
for each data point in parallel.
This function invokes the given function object func
for each data point in parallel manner. The input parameters are i and j indices of a data point. The order of execution can be arbitrary since it's multi-threaded.
◆ Resize() [1/4]
void CubbyFlow::ScalarGrid3::Resize | ( | size_t | resolutionX, |
size_t | resolutionY, | ||
size_t | resolutionZ, | ||
double | gridSpacingX = 1.0 , |
||
double | gridSpacingY = 1.0 , |
||
double | gridSpacingZ = 1.0 , |
||
double | originX = 0.0 , |
||
double | originY = 0.0 , |
||
double | originZ = 0.0 , |
||
double | initialValue = 0.0 |
||
) |
Resizes the grid using given parameters.
◆ Resize() [2/4]
void CubbyFlow::ScalarGrid3::Resize | ( | const Size3 & | resolution, |
const Vector3D & | gridSpacing = Vector3D(1, 1, 1) , |
||
const Vector3D & | origin = Vector3D() , |
||
double | initialValue = 0.0 |
||
) |
Resizes the grid using given parameters.
◆ Resize() [3/4]
void CubbyFlow::ScalarGrid3::Resize | ( | double | gridSpacingX, |
double | gridSpacingY, | ||
double | gridSpacingZ, | ||
double | originX, | ||
double | originY, | ||
double | originZ | ||
) |
Resizes the grid using given parameters.
◆ Resize() [4/4]
Resizes the grid using given parameters.
◆ Sample()
|
overridevirtual |
Returns the sampled value at given position x
.
This function returns the data sampled at arbitrary position x
. The sampling function is linear.
Implements CubbyFlow::ScalarField3.
◆ Sampler()
|
overridevirtual |
Returns the sampler function.
This function returns the data sampler function object. The sampling function is linear.
Reimplemented from CubbyFlow::ScalarField3.
◆ Serialize()
|
overridevirtual |
Serializes the grid instance to the output buffer.
Implements CubbyFlow::Grid3.
◆ SetData()
|
overrideprotectedvirtual |
Sets the data from a continuous linear array.
Implements CubbyFlow::Grid3.
◆ SetScalarGrid()
|
protected |
Sets the data storage and predefined samplers with given grid.
◆ SwapScalarGrid()
|
protected |
Swaps the data storage and predefined samplers with given grid.
The documentation for this class was generated from the following file:
- Core/Grid/ScalarGrid3.h