Abstract base class for 3-D collocated vector grid structure. More...
#include <Core/Grid/CollocatedVectorGrid3.h>
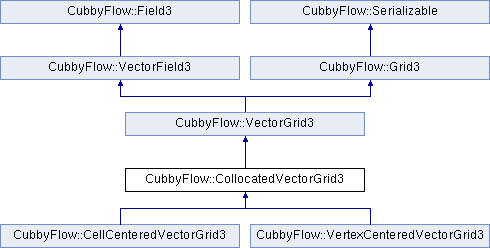
Public Member Functions | |
CollocatedVectorGrid3 () | |
Constructs an empty grid. More... | |
virtual | ~CollocatedVectorGrid3 () |
Default destructor. More... | |
virtual Size3 | GetDataSize () const =0 |
Returns the actual data point size. More... | |
virtual Vector3D | GetDataOrigin () const =0 |
Returns data position for the grid point at (0, 0, 0). More... | |
const Vector3D & | operator() (size_t i, size_t j, size_t k) const |
Returns the grid data at given data point. More... | |
Vector3D & | operator() (size_t i, size_t j, size_t k) |
Returns the grid data at given data point. More... | |
double | DivergenceAtDataPoint (size_t i, size_t j, size_t k) const |
Returns divergence at data point location. More... | |
Vector3D | CurlAtDataPoint (size_t i, size_t j, size_t k) const |
Returns curl at data point location. More... | |
VectorDataAccessor | GetDataAccessor () |
Returns the read-write data array accessor. More... | |
ConstVectorDataAccessor | GetConstDataAccessor () const |
Returns the read-only data array accessor. More... | |
DataPositionFunc | GetDataPosition () const |
Returns the function that maps data point to its position. More... | |
void | ForEachDataPointIndex (const std::function< void(size_t, size_t, size_t)> &func) const |
Invokes the given function func for each data point. More... | |
void | ParallelForEachDataPointIndex (const std::function< void(size_t, size_t, size_t)> &func) const |
Invokes the given function func for each data point in parallel. More... | |
Vector3D | Sample (const Vector3D &x) const override |
Returns sampled value at given position x . More... | |
double | Divergence (const Vector3D &x) const override |
Returns divergence at given position x . More... | |
Vector3D | Curl (const Vector3D &x) const override |
Returns curl at given position x . More... | |
std::function< Vector3D(const Vector3D &)> | Sampler () const override |
Returns the sampler function. More... | |
![]() | |
VectorGrid3 () | |
Constructs an empty grid. More... | |
virtual | ~VectorGrid3 () |
Default destructor. More... | |
void | Clear () |
Clears the contents of the grid. More... | |
void | Resize (size_t resolutionX, size_t resolutionY, size_t resolutionZ, double gridSpacingX=1.0, double gridSpacingY=1.0, double gridSpacingZ=1.0, double originX=0.0, double originY=0.0, double originZ=0.0, double initialValueX=0.0, double initialValueY=0.0, double initialValueZ=0.0) |
Resizes the grid using given parameters. More... | |
void | Resize (const Size3 &resolution, const Vector3D &gridSpacing=Vector3D(1, 1, 1), const Vector3D &origin=Vector3D(), const Vector3D &initialValue=Vector3D()) |
Resizes the grid using given parameters. More... | |
void | Resize (double gridSpacingX, double gridSpacingY, double gridSpacingZ, double originX, double originY, double originZ) |
Resizes the grid using given parameters. More... | |
void | Resize (const Vector3D &gridSpacing, const Vector3D &origin) |
Resizes the grid using given parameters. More... | |
virtual void | Fill (const Vector3D &value, ExecutionPolicy policy=ExecutionPolicy::Parallel)=0 |
Fills the grid with given value. More... | |
virtual void | Fill (const std::function< Vector3D(const Vector3D &)> &func, ExecutionPolicy policy=ExecutionPolicy::Parallel)=0 |
Fills the grid with given position-to-value mapping function. More... | |
virtual std::shared_ptr< VectorGrid3 > | Clone () const =0 |
Returns the copy of the grid instance. More... | |
void | Serialize (std::vector< uint8_t > *buffer) const override |
Serializes the grid instance to the output buffer. More... | |
void | Deserialize (const std::vector< uint8_t > &buffer) override |
Deserializes the input buffer to the grid instance. More... | |
![]() | |
VectorField3 () | |
Default constructor. More... | |
virtual | ~VectorField3 () |
Default destructor. More... | |
![]() | |
Field3 () | |
virtual | ~Field3 () |
![]() | |
Grid3 () | |
Constructs an empty grid. More... | |
virtual | ~Grid3 () |
Default destructor. More... | |
virtual std::string | TypeName () const =0 |
Returns the type name of derived grid. More... | |
const Size3 & | Resolution () const |
Returns the grid resolution. More... | |
const Vector3D & | Origin () const |
Returns the grid origin. More... | |
const Vector3D & | GridSpacing () const |
Returns the grid spacing. More... | |
const BoundingBox3D & | BoundingBox () const |
Returns the bounding box of the grid. More... | |
DataPositionFunc | CellCenterPosition () const |
Returns the function that maps grid index to the cell-center position. More... | |
void | ForEachCellIndex (const std::function< void(size_t, size_t, size_t)> &func) const |
Invokes the given function func for each grid cell. More... | |
void | ParallelForEachCellIndex (const std::function< void(size_t, size_t, size_t)> &func) const |
Invokes the given function func for each grid cell in parallel. More... | |
bool | HasSameShape (const Grid3 &other) const |
Returns true if resolution, grid-spacing and origin are same. More... | |
virtual void | Swap (Grid3 *other)=0 |
Swaps the data with other grid. More... | |
Protected Member Functions | |
void | SwapCollocatedVectorGrid (CollocatedVectorGrid3 *other) |
Swaps the data storage and predefined samplers with given grid. More... | |
void | SetCollocatedVectorGrid (const CollocatedVectorGrid3 &other) |
Sets the data storage and predefined samplers with given grid. More... | |
void | GetData (std::vector< double > *data) const override |
Fetches the data into a continuous linear array. More... | |
void | SetData (const std::vector< double > &data) override |
Sets the data from a continuous linear array. More... | |
![]() | |
void | SetSizeParameters (const Size3 &resolution, const Vector3D &gridSpacing, const Vector3D &origin) |
Sets the size parameters including the resolution, grid spacing, and origin. More... | |
void | SwapGrid (Grid3 *other) |
Swaps the size parameters with given grid other . More... | |
void | SetGrid (const Grid3 &other) |
Sets the size parameters with given grid other . More... | |
Additional Inherited Members | |
![]() | |
using | VectorDataAccessor = ArrayAccessor3< Vector3D > |
Read-write array accessor type. More... | |
using | ConstVectorDataAccessor = ConstArrayAccessor3< Vector3D > |
Read-only array accessor type. More... | |
![]() | |
using | DataPositionFunc = std::function< Vector3D(size_t, size_t, size_t)> |
Function type for mapping data index to actual position. More... | |
Detailed Description
Abstract base class for 3-D collocated vector grid structure.
Constructor & Destructor Documentation
◆ CollocatedVectorGrid3()
CubbyFlow::CollocatedVectorGrid3::CollocatedVectorGrid3 | ( | ) |
Constructs an empty grid.
◆ ~CollocatedVectorGrid3()
|
virtual |
Default destructor.
Member Function Documentation
◆ Curl()
Returns curl at given position x
.
Reimplemented from CubbyFlow::VectorField3.
◆ CurlAtDataPoint()
Vector3D CubbyFlow::CollocatedVectorGrid3::CurlAtDataPoint | ( | size_t | i, |
size_t | j, | ||
size_t | k | ||
) | const |
Returns curl at data point location.
◆ Divergence()
|
overridevirtual |
Returns divergence at given position x
.
Reimplemented from CubbyFlow::VectorField3.
◆ DivergenceAtDataPoint()
double CubbyFlow::CollocatedVectorGrid3::DivergenceAtDataPoint | ( | size_t | i, |
size_t | j, | ||
size_t | k | ||
) | const |
Returns divergence at data point location.
◆ ForEachDataPointIndex()
void CubbyFlow::CollocatedVectorGrid3::ForEachDataPointIndex | ( | const std::function< void(size_t, size_t, size_t)> & | func | ) | const |
Invokes the given function func
for each data point.
This function invokes the given function object func
for each data point in serial manner. The input parameters are i and j indices of a data point. The order of execution is i-first, j-last.
◆ GetConstDataAccessor()
ConstVectorDataAccessor CubbyFlow::CollocatedVectorGrid3::GetConstDataAccessor | ( | ) | const |
Returns the read-only data array accessor.
◆ GetData()
|
overrideprotectedvirtual |
Fetches the data into a continuous linear array.
Implements CubbyFlow::Grid3.
◆ GetDataAccessor()
VectorDataAccessor CubbyFlow::CollocatedVectorGrid3::GetDataAccessor | ( | ) |
Returns the read-write data array accessor.
◆ GetDataOrigin()
|
pure virtual |
Returns data position for the grid point at (0, 0, 0).
Note that this is different from origin() since origin() returns the lower corner point of the bounding box.
Implemented in CubbyFlow::CellCenteredVectorGrid3, and CubbyFlow::VertexCenteredVectorGrid3.
◆ GetDataPosition()
DataPositionFunc CubbyFlow::CollocatedVectorGrid3::GetDataPosition | ( | ) | const |
Returns the function that maps data point to its position.
◆ GetDataSize()
|
pure virtual |
Returns the actual data point size.
Implemented in CubbyFlow::CellCenteredVectorGrid3, and CubbyFlow::VertexCenteredVectorGrid3.
◆ operator()() [1/2]
const Vector3D& CubbyFlow::CollocatedVectorGrid3::operator() | ( | size_t | i, |
size_t | j, | ||
size_t | k | ||
) | const |
Returns the grid data at given data point.
◆ operator()() [2/2]
Vector3D& CubbyFlow::CollocatedVectorGrid3::operator() | ( | size_t | i, |
size_t | j, | ||
size_t | k | ||
) |
Returns the grid data at given data point.
◆ ParallelForEachDataPointIndex()
void CubbyFlow::CollocatedVectorGrid3::ParallelForEachDataPointIndex | ( | const std::function< void(size_t, size_t, size_t)> & | func | ) | const |
Invokes the given function func
for each data point in parallel.
This function invokes the given function object func
for each data point in parallel manner. The input parameters are i and j indices of a data point. The order of execution can be arbitrary since it's multi-threaded.
◆ Sample()
Returns sampled value at given position x
.
Implements CubbyFlow::VectorField3.
◆ Sampler()
|
overridevirtual |
Returns the sampler function.
This function returns the data sampler function object. The sampling function is linear.
Reimplemented from CubbyFlow::VectorField3.
◆ SetCollocatedVectorGrid()
|
protected |
Sets the data storage and predefined samplers with given grid.
◆ SetData()
|
overrideprotectedvirtual |
Sets the data from a continuous linear array.
Implements CubbyFlow::Grid3.
◆ SwapCollocatedVectorGrid()
|
protected |
Swaps the data storage and predefined samplers with given grid.
The documentation for this class was generated from the following file:
- Core/Grid/CollocatedVectorGrid3.h