Abstract base class for grid-based 3-D fluid solver. More...
#include <Core/Solver/Grid/GridFluidSolver3.h>
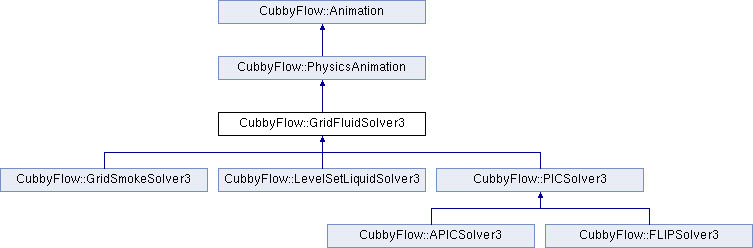
Classes | |
class | Builder |
Front-end to create GridFluidSolver3 objects step by step. More... | |
Public Member Functions | |
GridFluidSolver3 () | |
Default constructor. More... | |
GridFluidSolver3 (const Size3 &resolution, const Vector3D &gridSpacing, const Vector3D &gridOrigin) | |
Constructs solver with initial grid size. More... | |
virtual | ~GridFluidSolver3 () |
Default destructor. More... | |
const Vector3D & | GetGravity () const |
Returns the gravity vector of the system. More... | |
void | SetGravity (const Vector3D &newGravity) |
Sets the gravity of the system. More... | |
double | GetViscosityCoefficient () const |
Returns the viscosity coefficient. More... | |
void | SetViscosityCoefficient (double newValue) |
Sets the viscosity coefficient. More... | |
double | GetCFL (double timeIntervalInSeconds) const |
Returns the CFL number from the current velocity field for given time interval. More... | |
double | GetMaxCFL () const |
Returns the max allowed CFL number. More... | |
void | SetMaxCFL (double newCFL) |
Sets the max allowed CFL number. More... | |
bool | GetUseCompressedLinearSystem () const |
Returns true if the solver is using compressed linear system. More... | |
void | SetUseCompressedLinearSystem (bool onoff) |
Sets whether the solver should use compressed linear system. More... | |
const AdvectionSolver3Ptr & | GetAdvectionSolver () const |
Returns the advection solver instance. More... | |
void | SetAdvectionSolver (const AdvectionSolver3Ptr &newSolver) |
Sets the advection solver. More... | |
const GridDiffusionSolver3Ptr & | GetDiffusionSolver () const |
Returns the diffusion solver instance. More... | |
void | SetDiffusionSolver (const GridDiffusionSolver3Ptr &newSolver) |
Sets the diffusion solver. More... | |
const GridPressureSolver3Ptr & | GetPressureSolver () const |
Returns the pressure solver instance. More... | |
void | SetPressureSolver (const GridPressureSolver3Ptr &newSolver) |
Sets the pressure solver. More... | |
int | GetClosedDomainBoundaryFlag () const |
Returns the closed domain boundary flag. More... | |
void | SetClosedDomainBoundaryFlag (int flag) |
Sets the closed domain boundary flag. More... | |
const GridSystemData3Ptr & | GetGridSystemData () const |
Returns the grid system data. More... | |
void | ResizeGrid (const Size3 &newSize, const Vector3D &newGridSpacing, const Vector3D &newGridOrigin) const |
Resizes grid system data. More... | |
Size3 | GetResolution () const |
Returns the resolution of the grid system data. More... | |
Vector3D | GetGridSpacing () const |
Returns the grid spacing of the grid system data. More... | |
Vector3D | GetGridOrigin () const |
Returns the origin of the grid system data. More... | |
const FaceCenteredGrid3Ptr & | GetVelocity () const |
Returns the velocity field. More... | |
const Collider3Ptr & | GetCollider () const |
Returns the collider. More... | |
void | SetCollider (const Collider3Ptr &newCollider) |
Sets the collider. More... | |
const GridEmitter3Ptr & | GetEmitter () const |
Returns the emitter. More... | |
void | SetEmitter (const GridEmitter3Ptr &newEmitter) |
Sets the emitter. More... | |
![]() | |
PhysicsAnimation () | |
Default constructor. More... | |
virtual | ~PhysicsAnimation () |
Destructor. More... | |
bool | GetIsUsingFixedSubTimeSteps () const |
Returns true if fixed sub-timestepping is used. More... | |
void | SetIsUsingFixedSubTimeSteps (bool isUsing) |
Sets true if fixed sub-timestepping is used. More... | |
unsigned int | GetNumberOfFixedSubTimeSteps () const |
Returns the number of fixed sub-timesteps. More... | |
void | SetNumberOfFixedSubTimeSteps (unsigned int numberOfSteps) |
Sets the number of fixed sub-timesteps. More... | |
void | AdvanceSingleFrame () |
Advances a single frame. More... | |
Frame | GetCurrentFrame () const |
Returns current frame. More... | |
void | SetCurrentFrame (const Frame &frame) |
Sets current frame cursor (but do not invoke update()). More... | |
double | GetCurrentTimeInSeconds () const |
Returns current time in seconds. More... | |
![]() | |
Animation () | |
virtual | ~Animation () |
void | Update (const Frame &frame) |
Updates animation state for given frame . More... | |
Static Public Member Functions | |
static Builder | GetBuilder () |
Returns builder fox GridFluidSolver3. More... | |
Protected Member Functions | |
void | OnInitialize () override |
Called when it needs to setup initial condition. More... | |
void | OnAdvanceTimeStep (double timeIntervalInSeconds) override |
Called when advancing a single time-step. More... | |
unsigned int | GetNumberOfSubTimeSteps (double timeIntervalInSeconds) const override |
Returns the required sub-time-steps for given time interval. More... | |
virtual void | OnBeginAdvanceTimeStep (double timeIntervalInSeconds) |
Called at the beginning of a time-step. More... | |
virtual void | OnEndAdvanceTimeStep (double timeIntervalInSeconds) |
Called at the end of a time-step. More... | |
virtual void | ComputeExternalForces (double timeIntervalInSeconds) |
Computes the external force terms. More... | |
virtual void | ComputeViscosity (double timeIntervalInSeconds) |
Computes the viscosity term using the diffusion solver. More... | |
virtual void | ComputePressure (double timeIntervalInSeconds) |
Computes the pressure term using the pressure solver. More... | |
virtual void | ComputeAdvection (double timeIntervalInSeconds) |
Computes the advection term using the advection solver. More... | |
virtual ScalarField3Ptr | GetFluidSDF () const |
Returns the signed-distance representation of the fluid. More... | |
void | ComputeGravity (double timeIntervalInSeconds) |
Computes the gravity term. More... | |
void | ApplyBoundaryCondition () const |
Applies the boundary condition to the velocity field. More... | |
void | ExtrapolateIntoCollider (ScalarGrid3 *grid) |
Extrapolates given field into the collider-occupied region. More... | |
void | ExtrapolateIntoCollider (CollocatedVectorGrid3 *grid) |
Extrapolates given field into the collider-occupied region. More... | |
void | ExtrapolateIntoCollider (FaceCenteredGrid3 *grid) |
Extrapolates given field into the collider-occupied region. More... | |
ScalarField3Ptr | GetColliderSDF () const |
Returns the signed-distance field representation of the collider. More... | |
VectorField3Ptr | GetColliderVelocityField () const |
Returns the velocity field of the collider. More... | |
Detailed Description
Abstract base class for grid-based 3-D fluid solver.
This is an abstract base class for grid-based 3-D fluid solver based on Jos Stam's famous 1999 paper - "Stable Fluids". This solver takes fractional step method as its foundation which is consisted of independent advection, diffusion, external forces, and pressure projection steps. Each step is configurable so that a custom step can be implemented. For example, if a user wants to change the advection solver to her/his own implementation, simply call GridFluidSolver3::setAdvectionSolver(newSolver).
Constructor & Destructor Documentation
◆ GridFluidSolver3() [1/2]
CubbyFlow::GridFluidSolver3::GridFluidSolver3 | ( | ) |
Default constructor.
◆ GridFluidSolver3() [2/2]
CubbyFlow::GridFluidSolver3::GridFluidSolver3 | ( | const Size3 & | resolution, |
const Vector3D & | gridSpacing, | ||
const Vector3D & | gridOrigin | ||
) |
Constructs solver with initial grid size.
◆ ~GridFluidSolver3()
|
virtual |
Default destructor.
Member Function Documentation
◆ ApplyBoundaryCondition()
|
protected |
Applies the boundary condition to the velocity field.
This function applies the boundary condition to the velocity field by constraining the flow based on the boundary condition solver.
◆ ComputeAdvection()
|
protectedvirtual |
Computes the advection term using the advection solver.
Reimplemented in CubbyFlow::LevelSetLiquidSolver3, and CubbyFlow::PICSolver3.
◆ ComputeExternalForces()
|
protectedvirtual |
Computes the external force terms.
This function computes the external force applied for given time interval. By default, it only computes the gravity.
- See also
- GridFluidSolver3::ComputeGravity
Reimplemented in CubbyFlow::GridSmokeSolver3.
◆ ComputeGravity()
|
protected |
Computes the gravity term.
◆ ComputePressure()
|
protectedvirtual |
Computes the pressure term using the pressure solver.
◆ ComputeViscosity()
|
protectedvirtual |
Computes the viscosity term using the diffusion solver.
◆ ExtrapolateIntoCollider() [1/3]
|
protected |
Extrapolates given field into the collider-occupied region.
◆ ExtrapolateIntoCollider() [2/3]
|
protected |
Extrapolates given field into the collider-occupied region.
◆ ExtrapolateIntoCollider() [3/3]
|
protected |
Extrapolates given field into the collider-occupied region.
◆ GetAdvectionSolver()
const AdvectionSolver3Ptr& CubbyFlow::GridFluidSolver3::GetAdvectionSolver | ( | ) | const |
Returns the advection solver instance.
◆ GetBuilder()
|
static |
Returns builder fox GridFluidSolver3.
◆ GetCFL()
double CubbyFlow::GridFluidSolver3::GetCFL | ( | double | timeIntervalInSeconds | ) | const |
Returns the CFL number from the current velocity field for given time interval.
- Parameters
-
[in] timeIntervalInSeconds The time interval in seconds.
◆ GetClosedDomainBoundaryFlag()
int CubbyFlow::GridFluidSolver3::GetClosedDomainBoundaryFlag | ( | ) | const |
Returns the closed domain boundary flag.
◆ GetCollider()
const Collider3Ptr& CubbyFlow::GridFluidSolver3::GetCollider | ( | ) | const |
Returns the collider.
◆ GetColliderSDF()
|
protected |
Returns the signed-distance field representation of the collider.
◆ GetColliderVelocityField()
|
protected |
Returns the velocity field of the collider.
◆ GetDiffusionSolver()
const GridDiffusionSolver3Ptr& CubbyFlow::GridFluidSolver3::GetDiffusionSolver | ( | ) | const |
Returns the diffusion solver instance.
◆ GetEmitter()
const GridEmitter3Ptr& CubbyFlow::GridFluidSolver3::GetEmitter | ( | ) | const |
Returns the emitter.
◆ GetFluidSDF()
|
protectedvirtual |
Returns the signed-distance representation of the fluid.
This function returns the signed-distance representation of the fluid. Positive sign area is considered to be atmosphere and won't be included for computing the dynamics. By default, this will return constant scalar field of -std::numeric_limits<double>::max(), meaning that the entire volume is occupied with fluid.
Reimplemented in CubbyFlow::LevelSetLiquidSolver3, and CubbyFlow::PICSolver3.
◆ GetGravity()
const Vector3D& CubbyFlow::GridFluidSolver3::GetGravity | ( | ) | const |
Returns the gravity vector of the system.
◆ GetGridOrigin()
Vector3D CubbyFlow::GridFluidSolver3::GetGridOrigin | ( | ) | const |
Returns the origin of the grid system data.
This function returns the resolution of the grid system data. This is equivalent to calling gridSystemData()->origin(), but provides a shortcut.
◆ GetGridSpacing()
Vector3D CubbyFlow::GridFluidSolver3::GetGridSpacing | ( | ) | const |
Returns the grid spacing of the grid system data.
This function returns the resolution of the grid system data. This is equivalent to calling gridSystemData()->gridSpacing(), but provides a shortcut.
◆ GetGridSystemData()
const GridSystemData3Ptr& CubbyFlow::GridFluidSolver3::GetGridSystemData | ( | ) | const |
Returns the grid system data.
This function returns the grid system data. The grid system data stores the core fluid flow fields such as velocity. By default, the data instance has velocity field only.
- See also
- GridSystemData3
◆ GetMaxCFL()
double CubbyFlow::GridFluidSolver3::GetMaxCFL | ( | ) | const |
Returns the max allowed CFL number.
◆ GetNumberOfSubTimeSteps()
|
overrideprotectedvirtual |
Returns the required sub-time-steps for given time interval.
This function returns the required sub-time-steps for given time interval based on the max allowed CFL number. If the time interval is too large so that it makes the CFL number greater than the max value, This function will return a number that is greater than 1.
- See also
- GridFluidSolver3::GetMaxCFL
Reimplemented from CubbyFlow::PhysicsAnimation.
◆ GetPressureSolver()
const GridPressureSolver3Ptr& CubbyFlow::GridFluidSolver3::GetPressureSolver | ( | ) | const |
Returns the pressure solver instance.
◆ GetResolution()
Size3 CubbyFlow::GridFluidSolver3::GetResolution | ( | ) | const |
Returns the resolution of the grid system data.
This function returns the resolution of the grid system data. This is equivalent to calling gridSystemData()->resolution(), but provides a shortcut.
◆ GetUseCompressedLinearSystem()
bool CubbyFlow::GridFluidSolver3::GetUseCompressedLinearSystem | ( | ) | const |
Returns true if the solver is using compressed linear system.
◆ GetVelocity()
const FaceCenteredGrid3Ptr& CubbyFlow::GridFluidSolver3::GetVelocity | ( | ) | const |
Returns the velocity field.
This function returns the velocity field from the grid system data. It is just a shortcut to the most commonly accessed data chunk.
◆ GetViscosityCoefficient()
double CubbyFlow::GridFluidSolver3::GetViscosityCoefficient | ( | ) | const |
Returns the viscosity coefficient.
◆ OnAdvanceTimeStep()
|
overrideprotectedvirtual |
Called when advancing a single time-step.
Implements CubbyFlow::PhysicsAnimation.
◆ OnBeginAdvanceTimeStep()
|
protectedvirtual |
Called at the beginning of a time-step.
Reimplemented in CubbyFlow::LevelSetLiquidSolver3, and CubbyFlow::PICSolver3.
◆ OnEndAdvanceTimeStep()
|
protectedvirtual |
Called at the end of a time-step.
Reimplemented in CubbyFlow::GridSmokeSolver3, and CubbyFlow::LevelSetLiquidSolver3.
◆ OnInitialize()
|
overrideprotectedvirtual |
Called when it needs to setup initial condition.
Reimplemented from CubbyFlow::PhysicsAnimation.
Reimplemented in CubbyFlow::PICSolver3.
◆ ResizeGrid()
void CubbyFlow::GridFluidSolver3::ResizeGrid | ( | const Size3 & | newSize, |
const Vector3D & | newGridSpacing, | ||
const Vector3D & | newGridOrigin | ||
) | const |
Resizes grid system data.
This function resizes grid system data. You can also resize the grid by calling resize function directly from GridFluidSolver3::gridSystemData(), but this function provides a shortcut for the same operation.
- Parameters
-
[in] newSize The new size. [in] newGridSpacing The new grid spacing. [in] newGridOrigin The new grid origin.
◆ SetAdvectionSolver()
void CubbyFlow::GridFluidSolver3::SetAdvectionSolver | ( | const AdvectionSolver3Ptr & | newSolver | ) |
Sets the advection solver.
◆ SetClosedDomainBoundaryFlag()
void CubbyFlow::GridFluidSolver3::SetClosedDomainBoundaryFlag | ( | int | flag | ) |
Sets the closed domain boundary flag.
◆ SetCollider()
void CubbyFlow::GridFluidSolver3::SetCollider | ( | const Collider3Ptr & | newCollider | ) |
Sets the collider.
◆ SetDiffusionSolver()
void CubbyFlow::GridFluidSolver3::SetDiffusionSolver | ( | const GridDiffusionSolver3Ptr & | newSolver | ) |
Sets the diffusion solver.
◆ SetEmitter()
void CubbyFlow::GridFluidSolver3::SetEmitter | ( | const GridEmitter3Ptr & | newEmitter | ) |
Sets the emitter.
◆ SetGravity()
void CubbyFlow::GridFluidSolver3::SetGravity | ( | const Vector3D & | newGravity | ) |
Sets the gravity of the system.
◆ SetMaxCFL()
void CubbyFlow::GridFluidSolver3::SetMaxCFL | ( | double | newCFL | ) |
Sets the max allowed CFL number.
◆ SetPressureSolver()
void CubbyFlow::GridFluidSolver3::SetPressureSolver | ( | const GridPressureSolver3Ptr & | newSolver | ) |
Sets the pressure solver.
◆ SetUseCompressedLinearSystem()
void CubbyFlow::GridFluidSolver3::SetUseCompressedLinearSystem | ( | bool | onoff | ) |
Sets whether the solver should use compressed linear system.
◆ SetViscosityCoefficient()
void CubbyFlow::GridFluidSolver3::SetViscosityCoefficient | ( | double | newValue | ) |
Sets the viscosity coefficient.
This function sets the viscosity coefficient. Non-positive input will be clamped to zero.
- Parameters
-
[in] newValue The new viscosity coefficient value.
The documentation for this class was generated from the following file:
- Core/Solver/Grid/GridFluidSolver3.h