2-D Vertex-centered vector grid structure. More...
#include <Core/Grid/VertexCenteredVectorGrid2.h>
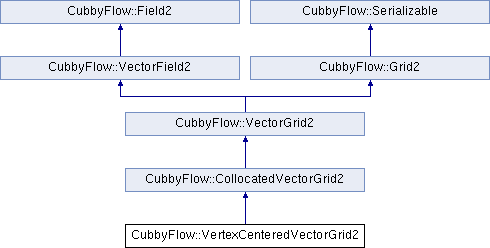
Classes | |
class | Builder |
A grid builder class that returns 2-D vertex-centered Vector grid. More... | |
Public Member Functions | |
VertexCenteredVectorGrid2 () | |
Constructs zero-sized grid. More... | |
VertexCenteredVectorGrid2 (size_t resolutionX, size_t resolutionY, double gridSpacingX=1.0, double gridSpacingY=1.0, double originX=0.0, double originY=0.0, double initialValueU=0.0, double initialValueV=0.0) | |
VertexCenteredVectorGrid2 (const Size2 &resolution, const Vector2D &gridSpacing=Vector2D(1.0, 1.0), const Vector2D &origin=Vector2D(), const Vector2D &initialValue=Vector2D()) | |
VertexCenteredVectorGrid2 (const VertexCenteredVectorGrid2 &other) | |
Copy constructor. More... | |
Size2 | GetDataSize () const override |
Returns the actual data point size. More... | |
Vector2D | GetDataOrigin () const override |
void | Swap (Grid2 *other) override |
Swaps the contents with the given other grid. More... | |
void | Set (const VertexCenteredVectorGrid2 &other) |
Sets the contents with the given other grid. More... | |
VertexCenteredVectorGrid2 & | operator= (const VertexCenteredVectorGrid2 &other) |
Sets the contents with the given other grid. More... | |
void | Fill (const Vector2D &value, ExecutionPolicy policy=ExecutionPolicy::Parallel) override |
Fills the grid with given value. More... | |
void | Fill (const std::function< Vector2D(const Vector2D &)> &func, ExecutionPolicy policy=ExecutionPolicy::Parallel) override |
Fills the grid with given function. More... | |
std::shared_ptr< VectorGrid2 > | Clone () const override |
Returns the copy of the grid instance. More... | |
![]() | |
CollocatedVectorGrid2 () | |
Constructs an empty grid. More... | |
virtual | ~CollocatedVectorGrid2 () |
Default destructor. More... | |
const Vector2D & | operator() (size_t i, size_t j) const |
Returns the grid data at given data point. More... | |
Vector2D & | operator() (size_t i, size_t j) |
Returns the grid data at given data point. More... | |
double | DivergenceAtDataPoint (size_t i, size_t j) const |
Returns divergence at data point location. More... | |
double | CurlAtDataPoint (size_t i, size_t j) const |
Returns curl at data point location. More... | |
VectorDataAccessor | GetDataAccessor () |
Returns the read-write data array accessor. More... | |
ConstVectorDataAccessor | GetConstDataAccessor () const |
Returns the read-only data array accessor. More... | |
DataPositionFunc | GetDataPosition () const |
Returns the function that maps data point to its position. More... | |
void | ForEachDataPointIndex (const std::function< void(size_t, size_t)> &func) const |
Invokes the given function func for each data point. More... | |
void | ParallelForEachDataPointIndex (const std::function< void(size_t, size_t)> &func) const |
Invokes the given function func for each data point in parallel. More... | |
Vector2D | Sample (const Vector2D &x) const override |
Returns sampled value at given position x . More... | |
double | Divergence (const Vector2D &x) const override |
Returns divergence at given position x . More... | |
double | Curl (const Vector2D &x) const override |
Returns curl at given position x . More... | |
std::function< Vector2D(const Vector2D &)> | Sampler () const override |
Returns the sampler function. More... | |
![]() | |
VectorGrid2 () | |
Constructs an empty grid. More... | |
virtual | ~VectorGrid2 () |
Default destructor. More... | |
void | Clear () |
Clears the contents of the grid. More... | |
void | Resize (size_t resolutionX, size_t resolutionY, double gridSpacingX=1.0, double gridSpacingY=1.0, double originX=0.0, double originY=0.0, double initialValueX=0.0, double initialValueY=0.0) |
Resizes the grid using given parameters. More... | |
void | Resize (const Size2 &resolution, const Vector2D &gridSpacing=Vector2D(1, 1), const Vector2D &origin=Vector2D(), const Vector2D &initialValue=Vector2D()) |
Resizes the grid using given parameters. More... | |
void | Resize (double gridSpacingX, double gridSpacingY, double originX, double originY) |
Resizes the grid using given parameters. More... | |
void | Resize (const Vector2D &gridSpacing, const Vector2D &origin) |
Resizes the grid using given parameters. More... | |
void | Serialize (std::vector< uint8_t > *buffer) const override |
Serializes the grid instance to the output buffer. More... | |
void | Deserialize (const std::vector< uint8_t > &buffer) override |
Deserializes the input buffer to the grid instance. More... | |
![]() | |
VectorField2 () | |
Default constructor. More... | |
virtual | ~VectorField2 () |
Default destructor. More... | |
![]() | |
Field2 () | |
virtual | ~Field2 () |
![]() | |
Grid2 () | |
Constructs an empty grid. More... | |
virtual | ~Grid2 () |
Default destructor. More... | |
virtual std::string | TypeName () const =0 |
Returns the type name of derived grid. More... | |
const Size2 & | Resolution () const |
Returns the grid resolution. More... | |
const Vector2D & | Origin () const |
Returns the grid origin. More... | |
const Vector2D & | GridSpacing () const |
Returns the grid spacing. More... | |
const BoundingBox2D & | BoundingBox () const |
Returns the bounding box of the grid. More... | |
DataPositionFunc | CellCenterPosition () const |
Returns the function that maps grid index to the cell-center position. More... | |
void | ForEachCellIndex (const std::function< void(size_t, size_t)> &func) const |
Invokes the given function func for each grid cell. More... | |
void | ParallelForEachCellIndex (const std::function< void(size_t, size_t)> &func) const |
Invokes the given function func for each grid cell in parallel. More... | |
bool | HasSameShape (const Grid2 &other) const |
Returns true if resolution, grid-spacing and origin are same. More... | |
Static Public Member Functions | |
static Builder | GetBuilder () |
Returns builder fox VertexCenteredVectorGrid2. More... | |
Additional Inherited Members | |
![]() | |
using | VectorDataAccessor = ArrayAccessor2< Vector2D > |
Read-write array accessor type. More... | |
using | ConstVectorDataAccessor = ConstArrayAccessor2< Vector2D > |
Read-only array accessor type. More... | |
![]() | |
using | DataPositionFunc = std::function< Vector2D(size_t, size_t)> |
Function type for mapping data index to actual position. More... | |
![]() | |
void | SwapCollocatedVectorGrid (CollocatedVectorGrid2 *other) |
Swaps the data storage and predefined samplers with given grid. More... | |
void | SetCollocatedVectorGrid (const CollocatedVectorGrid2 &other) |
Sets the data storage and predefined samplers with given grid. More... | |
void | GetData (std::vector< double > *data) const override |
Fetches the data into a continuous linear array. More... | |
void | SetData (const std::vector< double > &data) override |
Sets the data from a continuous linear array. More... | |
![]() | |
void | SetSizeParameters (const Size2 &resolution, const Vector2D &gridSpacing, const Vector2D &origin) |
Sets the size parameters including the resolution, grid spacing, and origin. More... | |
void | SwapGrid (Grid2 *other) |
Swaps the size parameters with given grid other . More... | |
void | SetGrid (const Grid2 &other) |
Sets the size parameters with given grid other . More... | |
Detailed Description
2-D Vertex-centered vector grid structure.
This class represents 2-D vertex-centered vector grid which extends CollocatedVectorGrid2. As its name suggests, the class defines the data point at the grid vertices (corners). Thus, A x B grid resolution will have (A+1) x (B+1) data points.
Constructor & Destructor Documentation
◆ VertexCenteredVectorGrid2() [1/4]
CubbyFlow::VertexCenteredVectorGrid2::VertexCenteredVectorGrid2 | ( | ) |
Constructs zero-sized grid.
◆ VertexCenteredVectorGrid2() [2/4]
CubbyFlow::VertexCenteredVectorGrid2::VertexCenteredVectorGrid2 | ( | size_t | resolutionX, |
size_t | resolutionY, | ||
double | gridSpacingX = 1.0 , |
||
double | gridSpacingY = 1.0 , |
||
double | originX = 0.0 , |
||
double | originY = 0.0 , |
||
double | initialValueU = 0.0 , |
||
double | initialValueV = 0.0 |
||
) |
Constructs a grid with given resolution, grid spacing, origin and initial value.
◆ VertexCenteredVectorGrid2() [3/4]
CubbyFlow::VertexCenteredVectorGrid2::VertexCenteredVectorGrid2 | ( | const Size2 & | resolution, |
const Vector2D & | gridSpacing = Vector2D(1.0, 1.0) , |
||
const Vector2D & | origin = Vector2D() , |
||
const Vector2D & | initialValue = Vector2D() |
||
) |
Constructs a grid with given resolution, grid spacing, origin and initial value.
◆ VertexCenteredVectorGrid2() [4/4]
CubbyFlow::VertexCenteredVectorGrid2::VertexCenteredVectorGrid2 | ( | const VertexCenteredVectorGrid2 & | other | ) |
Copy constructor.
Member Function Documentation
◆ Clone()
|
overridevirtual |
Returns the copy of the grid instance.
Implements CubbyFlow::VectorGrid2.
◆ Fill() [1/2]
|
overridevirtual |
Fills the grid with given value.
Implements CubbyFlow::VectorGrid2.
◆ Fill() [2/2]
|
overridevirtual |
Fills the grid with given function.
Implements CubbyFlow::VectorGrid2.
◆ GetBuilder()
|
static |
Returns builder fox VertexCenteredVectorGrid2.
◆ GetDataOrigin()
|
overridevirtual |
Returns data position for the grid point at (0, 0). Note that this is different from origin() since origin() returns the lower corner point of the bounding box.
Implements CubbyFlow::CollocatedVectorGrid2.
◆ GetDataSize()
|
overridevirtual |
Returns the actual data point size.
Implements CubbyFlow::CollocatedVectorGrid2.
◆ operator=()
VertexCenteredVectorGrid2& CubbyFlow::VertexCenteredVectorGrid2::operator= | ( | const VertexCenteredVectorGrid2 & | other | ) |
Sets the contents with the given other
grid.
◆ Set()
void CubbyFlow::VertexCenteredVectorGrid2::Set | ( | const VertexCenteredVectorGrid2 & | other | ) |
Sets the contents with the given other
grid.
◆ Swap()
|
overridevirtual |
Swaps the contents with the given other
grid.
This function swaps the contents of the grid instance with the given grid object other
only if other
has the same type with this grid.
Implements CubbyFlow::Grid2.
The documentation for this class was generated from the following file:
- Core/Grid/VertexCenteredVectorGrid2.h