Abstract base class for 2-D cartesian grid structure. More...
#include <Core/Grid/Grid2.h>
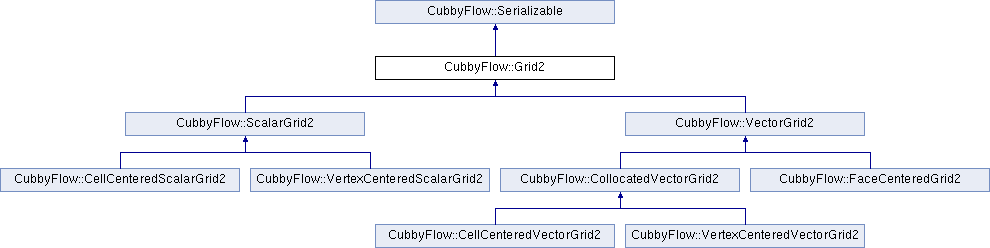
Public Types | |
using | DataPositionFunc = std::function< Vector2D(size_t, size_t)> |
Function type for mapping data index to actual position. More... | |
Public Member Functions | |
Grid2 () | |
Constructs an empty grid. More... | |
virtual | ~Grid2 () |
Default destructor. More... | |
virtual std::string | TypeName () const =0 |
Returns the type name of derived grid. More... | |
const Size2 & | Resolution () const |
Returns the grid resolution. More... | |
const Vector2D & | Origin () const |
Returns the grid origin. More... | |
const Vector2D & | GridSpacing () const |
Returns the grid spacing. More... | |
const BoundingBox2D & | BoundingBox () const |
Returns the bounding box of the grid. More... | |
DataPositionFunc | CellCenterPosition () const |
Returns the function that maps grid index to the cell-center position. More... | |
void | ForEachCellIndex (const std::function< void(size_t, size_t)> &func) const |
Invokes the given function func for each grid cell. More... | |
void | ParallelForEachCellIndex (const std::function< void(size_t, size_t)> &func) const |
Invokes the given function func for each grid cell in parallel. More... | |
bool | HasSameShape (const Grid2 &other) const |
Returns true if resolution, grid-spacing and origin are same. More... | |
virtual void | Swap (Grid2 *other)=0 |
Swaps the data with other grid. More... | |
![]() | |
virtual void | Serialize (std::vector< uint8_t > *buffer) const =0 |
Serializes this instance into the flat buffer. More... | |
virtual void | Deserialize (const std::vector< uint8_t > &buffer)=0 |
Deserializes this instance from the flat buffer. More... | |
Protected Member Functions | |
void | SetSizeParameters (const Size2 &resolution, const Vector2D &gridSpacing, const Vector2D &origin) |
Sets the size parameters including the resolution, grid spacing, and origin. More... | |
void | SwapGrid (Grid2 *other) |
Swaps the size parameters with given grid other . More... | |
void | SetGrid (const Grid2 &other) |
Sets the size parameters with given grid other . More... | |
virtual void | GetData (std::vector< double > *data) const =0 |
Fetches the data into a continuous linear array. More... | |
virtual void | SetData (const std::vector< double > &data)=0 |
Sets the data from a continuous linear array. More... | |
Detailed Description
Abstract base class for 2-D cartesian grid structure.
This class represents 2-D cartesian grid structure. This class is an abstract base class and does not store any data. The class only stores the shape of the grid. The grid structure is axis-aligned and can have different grid spacing per axis.
Member Typedef Documentation
◆ DataPositionFunc
using CubbyFlow::Grid2::DataPositionFunc = std::function<Vector2D(size_t, size_t)> |
Function type for mapping data index to actual position.
Constructor & Destructor Documentation
◆ Grid2()
CubbyFlow::Grid2::Grid2 | ( | ) |
Constructs an empty grid.
◆ ~Grid2()
|
virtual |
Default destructor.
Member Function Documentation
◆ BoundingBox()
const BoundingBox2D& CubbyFlow::Grid2::BoundingBox | ( | ) | const |
Returns the bounding box of the grid.
◆ CellCenterPosition()
DataPositionFunc CubbyFlow::Grid2::CellCenterPosition | ( | ) | const |
Returns the function that maps grid index to the cell-center position.
◆ ForEachCellIndex()
void CubbyFlow::Grid2::ForEachCellIndex | ( | const std::function< void(size_t, size_t)> & | func | ) | const |
Invokes the given function func
for each grid cell.
This function invokes the given function object func
for each grid cell in serial manner. The input parameters are i and j indices of a grid cell. The order of execution is i-first, j-last.
◆ GetData()
|
protectedpure virtual |
Fetches the data into a continuous linear array.
Implemented in CubbyFlow::FaceCenteredGrid2, CubbyFlow::ScalarGrid2, and CubbyFlow::CollocatedVectorGrid2.
◆ GridSpacing()
const Vector2D& CubbyFlow::Grid2::GridSpacing | ( | ) | const |
Returns the grid spacing.
◆ HasSameShape()
bool CubbyFlow::Grid2::HasSameShape | ( | const Grid2 & | other | ) | const |
Returns true if resolution, grid-spacing and origin are same.
◆ Origin()
const Vector2D& CubbyFlow::Grid2::Origin | ( | ) | const |
Returns the grid origin.
◆ ParallelForEachCellIndex()
void CubbyFlow::Grid2::ParallelForEachCellIndex | ( | const std::function< void(size_t, size_t)> & | func | ) | const |
Invokes the given function func
for each grid cell in parallel.
This function invokes the given function object func
for each grid cell in parallel manner. The input parameters are i and j indices of a grid cell. The order of execution can be arbitrary since it's multi-threaded.
◆ Resolution()
const Size2& CubbyFlow::Grid2::Resolution | ( | ) | const |
Returns the grid resolution.
◆ SetData()
|
protectedpure virtual |
Sets the data from a continuous linear array.
Implemented in CubbyFlow::FaceCenteredGrid2, CubbyFlow::ScalarGrid2, and CubbyFlow::CollocatedVectorGrid2.
◆ SetGrid()
|
protected |
Sets the size parameters with given grid other
.
◆ SetSizeParameters()
|
protected |
Sets the size parameters including the resolution, grid spacing, and origin.
◆ Swap()
|
pure virtual |
Swaps the data with other grid.
Implemented in CubbyFlow::CellCenteredVectorGrid2, CubbyFlow::VertexCenteredScalarGrid2, CubbyFlow::CellCenteredScalarGrid2, CubbyFlow::VertexCenteredVectorGrid2, and CubbyFlow::FaceCenteredGrid2.
◆ SwapGrid()
|
protected |
Swaps the size parameters with given grid other
.
◆ TypeName()
|
pure virtual |
Returns the type name of derived grid.
The documentation for this class was generated from the following file:
- Core/Grid/Grid2.h