3-D volumetric particle emitter. More...
#include <Core/Emitter/VolumeParticleEmitter3.h>
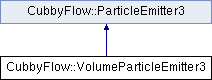
Classes | |
class | Builder |
Front-end to create VolumeParticleEmitter3 objects step by step. More... | |
Public Member Functions | |
VolumeParticleEmitter3 (const ImplicitSurface3Ptr &implicitSurface, const BoundingBox3D &bounds, double spacing, const Vector3D &initialVel=Vector3D(), size_t maxNumberOfParticles=std::numeric_limits< size_t >::max(), double jitter=0.0, bool isOneShot=true, bool allowOverlapping=false, uint32_t seed=0) | |
void | SetPointGenerator (const PointGenerator3Ptr &newPointsGen) |
Sets the point generator. More... | |
double | GetJitter () const |
Returns jitter amount. More... | |
void | SetJitter (double newJitter) |
Sets jitter amount between 0 and 1. More... | |
bool | GetIsOneShot () const |
Returns true if particles should be emitted just once. More... | |
void | SetIsOneShot (bool newValue) |
Sets the flag to true if particles are emitted just once. More... | |
bool | GetAllowOverlapping () const |
Returns true if particles can be overlapped. More... | |
void | SetAllowOverlapping (bool newValue) |
Sets the flag to true if particles can overlap each other. More... | |
size_t | GetMaxNumberOfParticles () const |
Returns max number of particles to be emitted. More... | |
void | SetMaxNumberOfParticles (size_t newMaxNumberOfParticles) |
Sets the max number of particles to be emitted. More... | |
double | GetSpacing () const |
Returns the spacing between particles. More... | |
void | SetSpacing (double newSpacing) |
Sets the spacing between particles. More... | |
Vector3D | GetInitialVelocity () const |
Sets the initial velocity of the particles. More... | |
void | SetInitialVelocity (const Vector3D &newInitialVel) |
Returns the initial velocity of the particles. More... | |
![]() | |
ParticleEmitter3 () | |
Default constructor. More... | |
virtual | ~ParticleEmitter3 () |
Destructor. More... | |
void | Update (double currentTimeInSeconds, double timeIntervalInSeconds) |
const ParticleSystemData3Ptr & | GetTarget () const |
Returns the target particle system to emit. More... | |
void | SetTarget (const ParticleSystemData3Ptr &particles) |
Sets the target particle system to emit. More... | |
void | SetOnBeginUpdateCallback (const OnBeginUpdateCallback &callback) |
Sets the callback function to be called when ParticleEmitter3::Update function is invoked. More... | |
Static Public Member Functions | |
static Builder | GetBuilder () |
Returns builder fox VolumeParticleEmitter3. More... | |
Additional Inherited Members | |
![]() | |
using | OnBeginUpdateCallback = std::function< void(ParticleEmitter3 *, double, double)> |
Callback function type for update calls. More... | |
![]() | |
virtual void | OnSetTarget (const ParticleSystemData3Ptr &particles) |
Called when ParticleEmitter3::SetTarget is executed. More... | |
Detailed Description
3-D volumetric particle emitter.
This class emits particles from volumetric geometry.
Constructor & Destructor Documentation
◆ VolumeParticleEmitter3()
CubbyFlow::VolumeParticleEmitter3::VolumeParticleEmitter3 | ( | const ImplicitSurface3Ptr & | implicitSurface, |
const BoundingBox3D & | bounds, | ||
double | spacing, | ||
const Vector3D & | initialVel = Vector3D() , |
||
size_t | maxNumberOfParticles = std::numeric_limits< size_t >::max() , |
||
double | jitter = 0.0 , |
||
bool | isOneShot = true , |
||
bool | allowOverlapping = false , |
||
uint32_t | seed = 0 |
||
) |
Constructs an emitter that spawns particles from given implicit surface which defines the volumetric geometry. Provided bounding box limits the particle generation region.
- Parameters
-
[in] implicitSurface The implicit surface. [in] bounds The max region. [in] spacing The spacing between particles. [in] initialVel The initial velocity. [in] maxNumberOfParticles The max number of particles to be emitted. [in] jitter The jitter amount between 0 and 1. [in] isOneShot Set true if particles are emitted just once. [in] allowOverlapping True if particles can be overlapped. [in] seed The random seed.
Member Function Documentation
◆ GetAllowOverlapping()
bool CubbyFlow::VolumeParticleEmitter3::GetAllowOverlapping | ( | ) | const |
Returns true if particles can be overlapped.
◆ GetBuilder()
|
static |
Returns builder fox VolumeParticleEmitter3.
◆ GetInitialVelocity()
Vector3D CubbyFlow::VolumeParticleEmitter3::GetInitialVelocity | ( | ) | const |
Sets the initial velocity of the particles.
◆ GetIsOneShot()
bool CubbyFlow::VolumeParticleEmitter3::GetIsOneShot | ( | ) | const |
Returns true if particles should be emitted just once.
◆ GetJitter()
double CubbyFlow::VolumeParticleEmitter3::GetJitter | ( | ) | const |
Returns jitter amount.
◆ GetMaxNumberOfParticles()
size_t CubbyFlow::VolumeParticleEmitter3::GetMaxNumberOfParticles | ( | ) | const |
Returns max number of particles to be emitted.
◆ GetSpacing()
double CubbyFlow::VolumeParticleEmitter3::GetSpacing | ( | ) | const |
Returns the spacing between particles.
◆ SetAllowOverlapping()
void CubbyFlow::VolumeParticleEmitter3::SetAllowOverlapping | ( | bool | newValue | ) |
Sets the flag to true if particles can overlap each other.
If true is set, the emitter will generate particles even if the new particles can find existing nearby particles within the particle spacing.
- Parameters
-
[in] newValue True if particles can be overlapped.
◆ SetInitialVelocity()
void CubbyFlow::VolumeParticleEmitter3::SetInitialVelocity | ( | const Vector3D & | newInitialVel | ) |
Returns the initial velocity of the particles.
◆ SetIsOneShot()
void CubbyFlow::VolumeParticleEmitter3::SetIsOneShot | ( | bool | newValue | ) |
Sets the flag to true if particles are emitted just once.
If true is set, the emitter will generate particles only once even after multiple emit calls. If false, it will keep generating particles from the volumetric geometry. Default value is true.
- Parameters
-
[in] newValue True if particles should be emitted just once.
◆ SetJitter()
void CubbyFlow::VolumeParticleEmitter3::SetJitter | ( | double | newJitter | ) |
Sets jitter amount between 0 and 1.
◆ SetMaxNumberOfParticles()
void CubbyFlow::VolumeParticleEmitter3::SetMaxNumberOfParticles | ( | size_t | newMaxNumberOfParticles | ) |
Sets the max number of particles to be emitted.
◆ SetPointGenerator()
void CubbyFlow::VolumeParticleEmitter3::SetPointGenerator | ( | const PointGenerator3Ptr & | newPointsGen | ) |
Sets the point generator.
This function sets the point generator that defines the pattern of the point distribution within the volume.
- Parameters
-
[in] newPointsGen The new points generator.
◆ SetSpacing()
void CubbyFlow::VolumeParticleEmitter3::SetSpacing | ( | double | newSpacing | ) |
Sets the spacing between particles.
The documentation for this class was generated from the following file:
- Core/Emitter/VolumeParticleEmitter3.h