General purpose dynamically-sizedN-D vector class. More...
#include <Core/Vector/VectorN.h>
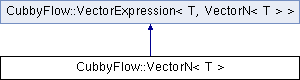
Public Types | |
using | ContainerType = std::vector< T > |
Public Member Functions | |
VectorN () | |
Constructs empty vector. More... | |
VectorN (size_t n, const T &val=0) | |
Constructs default vector (val, val, ... , val). More... | |
template<typename U > | |
VectorN (const std::initializer_list< U > &list) | |
Constructs vector with given initializer list. More... | |
template<typename E > | |
VectorN (const VectorExpression< T, E > &other) | |
Constructs vector with expression template. More... | |
VectorN (const VectorN &other) | |
Copy constructor. More... | |
VectorN (VectorN &&other) noexcept | |
Move constructor. More... | |
void | Resize (size_t n, const T &val=0) |
Resizes to n dimensional vector with initial value val . More... | |
void | Clear () |
Clears the vector and make it zero-dimensional. More... | |
void | Set (const T &s) |
Sets all elements to s . More... | |
template<typename U > | |
void | Set (const std::initializer_list< U > &list) |
Sets all elements with given initializer list. More... | |
template<typename E > | |
void | Set (const VectorExpression< T, E > &other) |
Sets vector with expression template. More... | |
void | Append (const T &val) |
Adds an element. More... | |
void | Swap (VectorN &other) |
Swaps the content of the vector with other vector. More... | |
void | SetZero () |
Sets all elements to zero. More... | |
void | Normalize () |
Normalizes this vector. More... | |
size_t | size () const |
Returns the size of the vector. More... | |
T * | data () |
Returns the raw pointer to the vector data. More... | |
const T * | data () const |
Returns the const raw pointer to the vector data. More... | |
ContainerType::iterator | begin () |
Returns the begin iterator of the vector. More... | |
ContainerType::const_iterator | begin () const |
Returns the begin const iterator of the vector. More... | |
ContainerType::iterator | end () |
Returns the end iterator of the vector. More... | |
ContainerType::const_iterator | end () const |
Returns the end const iterator of the vector. More... | |
ArrayAccessor1< T > | Accessor () |
Returns the array accessor. More... | |
ConstArrayAccessor1< T > | ConstAccessor () const |
Returns the const array accessor. More... | |
T | At (size_t i) const |
Returns const reference to the i -th element of the vector. More... | |
T & | At (size_t i) |
Returns reference to the i -th element of the vector. More... | |
T | Sum () const |
Returns the sum of all the elements. More... | |
T | Avg () const |
Returns the average of all the elements. More... | |
T | Min () const |
Returns the minimum element. More... | |
T | Max () const |
Returns the maximum element. More... | |
T | AbsMin () const |
Returns the absolute minimum element. More... | |
T | AbsMax () const |
Returns the absolute maximum element. More... | |
size_t | DominantAxis () const |
Returns the index of the dominant axis. More... | |
size_t | SubdominantAxis () const |
Returns the index of the subdominant axis. More... | |
VectorScalarDiv< T, VectorN > | Normalized () const |
Returns normalized vector. More... | |
T | Length () const |
Returns the length of the vector. More... | |
T | LengthSquared () const |
Returns the squared length of the vector. More... | |
template<typename E > | |
T | DistanceTo (const E &other) const |
Returns the distance to the other vector. More... | |
template<typename E > | |
T | DistanceSquaredTo (const E &other) const |
Returns the squared distance to the other vector. More... | |
template<typename U > | |
VectorTypeCast< U, VectorN< T >, T > | CastTo () const |
Returns a vector with different value type. More... | |
template<typename E > | |
bool | IsEqual (const E &other) const |
Returns true if other is the same as this vector. More... | |
template<typename E > | |
bool | IsSimilar (const E &other, T epsilon=std::numeric_limits< T >::epsilon()) const |
Returns true if other is similar to this vector. More... | |
template<typename E > | |
VectorAdd< T, VectorN, E > | Add (const E &v) const |
Computes this + v. More... | |
VectorScalarAdd< T, VectorN > | Add (const T &s) const |
Computes this + (s, s, ... , s). More... | |
template<typename E > | |
VectorSub< T, VectorN, E > | Sub (const E &v) const |
Computes this - v. More... | |
VectorScalarSub< T, VectorN > | Sub (const T &s) const |
Computes this - (s, s, ... , s). More... | |
template<typename E > | |
VectorMul< T, VectorN, E > | Mul (const E &v) const |
Computes this * v. More... | |
VectorScalarMul< T, VectorN > | Mul (const T &s) const |
Computes this * (s, s, ... , s). More... | |
template<typename E > | |
VectorDiv< T, VectorN, E > | Div (const E &v) const |
Computes this / v. More... | |
VectorScalarDiv< T, VectorN > | Div (const T &s) const |
Computes this / (s, s, ... , s). More... | |
template<typename E > | |
T | Dot (const E &v) const |
Computes dot product. More... | |
VectorScalarRSub< T, VectorN > | RSub (const T &s) const |
Computes (s, s, ... , s) - this. More... | |
template<typename E > | |
VectorSub< T, VectorN, E > | RSub (const E &v) const |
Computes v - this. More... | |
VectorScalarRDiv< T, VectorN > | RDiv (const T &s) const |
Computes (s, s, ... , s) / this. More... | |
template<typename E > | |
VectorDiv< T, VectorN, E > | RDiv (const E &v) const |
Computes v / this. More... | |
void | IAdd (const T &s) |
Computes this += (s, s, ... , s). More... | |
template<typename E > | |
void | IAdd (const E &v) |
Computes this += v. More... | |
void | ISub (const T &s) |
Computes this -= (s, s, ... , s). More... | |
template<typename E > | |
void | ISub (const E &v) |
Computes this -= v. More... | |
void | IMul (const T &s) |
Computes this *= (s, s, ... , s). More... | |
template<typename E > | |
void | IMul (const E &v) |
Computes this *= v. More... | |
void | IDiv (const T &s) |
Computes this /= (s, s, ... , s). More... | |
template<typename E > | |
void | IDiv (const E &v) |
Computes this /= v. More... | |
template<typename Callback > | |
void | ForEach (Callback func) const |
Iterates the vector and invoke given func for each element. More... | |
template<typename Callback > | |
void | ForEachIndex (Callback func) const |
Iterates the vector and invoke given func for each index. More... | |
template<typename Callback > | |
void | ParallelForEach (Callback func) |
Iterates the vector and invoke given func for each element in parallel using multi-threading. More... | |
template<typename Callback > | |
void | ParallelForEachIndex (Callback func) const |
Iterates the vector and invoke given func for each index in parallel using multi-threading. More... | |
T | operator[] (size_t i) const |
Returns the i -th element. More... | |
T & | operator[] (size_t i) |
Returns the reference to the i -th element. More... | |
template<typename U > | |
VectorN & | operator= (const std::initializer_list< U > &list) |
Sets vector with given initializer list. More... | |
template<typename E > | |
VectorN & | operator= (const VectorExpression< T, E > &other) |
Sets vector with expression template. More... | |
VectorN & | operator= (const VectorN &other) |
Copy assignment. More... | |
VectorN & | operator= (VectorN &&other) noexcept |
Move assignment. More... | |
VectorN & | operator+= (const T &s) |
Computes this += (s, s, ... , s) More... | |
template<typename E > | |
VectorN & | operator+= (const E &v) |
Computes this += v. More... | |
VectorN & | operator-= (const T &s) |
Computes this -= (s, s, ... , s) More... | |
template<typename E > | |
VectorN & | operator-= (const E &v) |
Computes this -= v. More... | |
VectorN & | operator*= (const T &s) |
Computes this *= (s, s, ... , s) More... | |
template<typename E > | |
VectorN & | operator*= (const E &v) |
Computes this *= v. More... | |
VectorN & | operator/= (const T &s) |
Computes this /= (s, s, ... , s) More... | |
template<typename E > | |
VectorN & | operator/= (const E &v) |
Computes this /= v. More... | |
template<typename E > | |
bool | operator== (const E &v) const |
Returns true if other is the same as this vector. More... | |
template<typename E > | |
bool | operator!= (const E &v) const |
Returns true if other is the not same as this vector. More... | |
template<typename E > | |
VectorAdd< T, VectorN< T >, E > | Add (const E &v) const |
template<typename E > | |
VectorSub< T, VectorN< T >, E > | Sub (const E &v) const |
template<typename E > | |
VectorMul< T, VectorN< T >, E > | Mul (const E &v) const |
template<typename E > | |
VectorDiv< T, VectorN< T >, E > | Div (const E &v) const |
template<typename E > | |
VectorSub< T, VectorN< T >, E > | RSub (const E &v) const |
template<typename E > | |
VectorDiv< T, VectorN< T >, E > | RDiv (const E &v) const |
template<typename U > | |
VectorN< T > & | operator= (const std::initializer_list< U > &list) |
template<typename E > | |
VectorN< T > & | operator= (const VectorExpression< T, E > &other) |
template<typename E > | |
VectorN< T > & | operator+= (const E &v) |
template<typename E > | |
VectorN< T > & | operator-= (const E &v) |
template<typename E > | |
VectorN< T > & | operator*= (const E &v) |
template<typename E > | |
VectorN< T > & | operator/= (const E &v) |
![]() | |
size_t | size () const |
Size of the vector. More... | |
const VectorN< T > & | operator() () const |
Returns actual implementation (the subclass). More... | |
Detailed Description
template<typename T>
class CubbyFlow::VectorN< T >
General purpose dynamically-sizedN-D vector class.
This class defines N-D vector data where its size can be defined dynamically.
- Template Parameters
-
T Type of the element.
Member Typedef Documentation
◆ ContainerType
using CubbyFlow::VectorN< T >::ContainerType = std::vector<T> |
Constructor & Destructor Documentation
◆ VectorN() [1/6]
CubbyFlow::VectorN< T >::VectorN | ( | ) |
Constructs empty vector.
◆ VectorN() [2/6]
CubbyFlow::VectorN< T >::VectorN | ( | size_t | n, |
const T & | val = 0 |
||
) |
Constructs default vector (val, val, ... , val).
◆ VectorN() [3/6]
CubbyFlow::VectorN< T >::VectorN | ( | const std::initializer_list< U > & | list | ) |
Constructs vector with given initializer list.
◆ VectorN() [4/6]
CubbyFlow::VectorN< T >::VectorN | ( | const VectorExpression< T, E > & | other | ) |
Constructs vector with expression template.
◆ VectorN() [5/6]
CubbyFlow::VectorN< T >::VectorN | ( | const VectorN< T > & | other | ) |
Copy constructor.
◆ VectorN() [6/6]
|
noexcept |
Move constructor.
Member Function Documentation
◆ AbsMax()
T CubbyFlow::VectorN< T >::AbsMax | ( | ) | const |
Returns the absolute maximum element.
◆ AbsMin()
T CubbyFlow::VectorN< T >::AbsMin | ( | ) | const |
Returns the absolute minimum element.
◆ Accessor()
ArrayAccessor1< T > CubbyFlow::VectorN< T >::Accessor | ( | ) |
Returns the array accessor.
◆ Add() [1/3]
VectorAdd<T, VectorN, E> CubbyFlow::VectorN< T >::Add | ( | const E & | v | ) | const |
Computes this + v.
◆ Add() [2/3]
VectorScalarAdd< T, VectorN< T > > CubbyFlow::VectorN< T >::Add | ( | const T & | s | ) | const |
Computes this + (s, s, ... , s).
◆ Add() [3/3]
VectorAdd<T, VectorN<T>, E> CubbyFlow::VectorN< T >::Add | ( | const E & | v | ) | const |
◆ Append()
void CubbyFlow::VectorN< T >::Append | ( | const T & | val | ) |
Adds an element.
◆ At() [1/2]
T CubbyFlow::VectorN< T >::At | ( | size_t | i | ) | const |
Returns const reference to the i
-th element of the vector.
◆ At() [2/2]
T & CubbyFlow::VectorN< T >::At | ( | size_t | i | ) |
Returns reference to the i
-th element of the vector.
◆ Avg()
T CubbyFlow::VectorN< T >::Avg | ( | ) | const |
Returns the average of all the elements.
◆ begin() [1/2]
VectorN< T >::ContainerType::iterator CubbyFlow::VectorN< T >::begin | ( | ) |
Returns the begin iterator of the vector.
◆ begin() [2/2]
VectorN< T >::ContainerType::const_iterator CubbyFlow::VectorN< T >::begin | ( | ) | const |
Returns the begin const iterator of the vector.
◆ CastTo()
VectorTypeCast< U, VectorN< T >, T > CubbyFlow::VectorN< T >::CastTo | ( | ) | const |
Returns a vector with different value type.
◆ Clear()
void CubbyFlow::VectorN< T >::Clear | ( | ) |
Clears the vector and make it zero-dimensional.
◆ ConstAccessor()
ConstArrayAccessor1< T > CubbyFlow::VectorN< T >::ConstAccessor | ( | ) | const |
Returns the const array accessor.
◆ data() [1/2]
T * CubbyFlow::VectorN< T >::data | ( | ) |
Returns the raw pointer to the vector data.
◆ data() [2/2]
const T * CubbyFlow::VectorN< T >::data | ( | ) | const |
Returns the const raw pointer to the vector data.
◆ DistanceSquaredTo()
T CubbyFlow::VectorN< T >::DistanceSquaredTo | ( | const E & | other | ) | const |
Returns the squared distance to the other vector.
◆ DistanceTo()
T CubbyFlow::VectorN< T >::DistanceTo | ( | const E & | other | ) | const |
Returns the distance to the other vector.
◆ Div() [1/3]
VectorDiv<T, VectorN, E> CubbyFlow::VectorN< T >::Div | ( | const E & | v | ) | const |
Computes this / v.
◆ Div() [2/3]
VectorScalarDiv< T, VectorN< T > > CubbyFlow::VectorN< T >::Div | ( | const T & | s | ) | const |
Computes this / (s, s, ... , s).
◆ Div() [3/3]
VectorDiv<T, VectorN<T>, E> CubbyFlow::VectorN< T >::Div | ( | const E & | v | ) | const |
◆ DominantAxis()
size_t CubbyFlow::VectorN< T >::DominantAxis | ( | ) | const |
Returns the index of the dominant axis.
◆ Dot()
Computes dot product.
◆ end() [1/2]
VectorN< T >::ContainerType::iterator CubbyFlow::VectorN< T >::end | ( | ) |
Returns the end iterator of the vector.
◆ end() [2/2]
VectorN< T >::ContainerType::const_iterator CubbyFlow::VectorN< T >::end | ( | ) | const |
Returns the end const iterator of the vector.
◆ ForEach()
void CubbyFlow::VectorN< T >::ForEach | ( | Callback | func | ) | const |
Iterates the vector and invoke given func
for each element.
This function iterates the vector elements and invoke the callback function func
. The callback function takes array's element as its input. The order of execution will be 0 to N-1 where N is the size of the vector. Below is the sample usage:
◆ ForEachIndex()
void CubbyFlow::VectorN< T >::ForEachIndex | ( | Callback | func | ) | const |
Iterates the vector and invoke given func
for each index.
This function iterates the vector elements and invoke the callback function func
. The callback function takes one parameter which is the index of the vector. The order of execution will be 0 to N-1 where N is the size of the array. Below is the sample usage:
◆ IAdd() [1/2]
void CubbyFlow::VectorN< T >::IAdd | ( | const T & | s | ) |
Computes this += (s, s, ... , s).
◆ IAdd() [2/2]
Computes this += v.
◆ IDiv() [1/2]
void CubbyFlow::VectorN< T >::IDiv | ( | const T & | s | ) |
Computes this /= (s, s, ... , s).
◆ IDiv() [2/2]
Computes this /= v.
◆ IMul() [1/2]
void CubbyFlow::VectorN< T >::IMul | ( | const T & | s | ) |
Computes this *= (s, s, ... , s).
◆ IMul() [2/2]
Computes this *= v.
◆ IsEqual()
bool CubbyFlow::VectorN< T >::IsEqual | ( | const E & | other | ) | const |
Returns true if other
is the same as this vector.
◆ IsSimilar()
bool CubbyFlow::VectorN< T >::IsSimilar | ( | const E & | other, |
T | epsilon = std::numeric_limits<T>::epsilon() |
||
) | const |
Returns true if other
is similar to this vector.
◆ ISub() [1/2]
void CubbyFlow::VectorN< T >::ISub | ( | const T & | s | ) |
Computes this -= (s, s, ... , s).
◆ ISub() [2/2]
Computes this -= v.
◆ Length()
T CubbyFlow::VectorN< T >::Length | ( | ) | const |
Returns the length of the vector.
◆ LengthSquared()
T CubbyFlow::VectorN< T >::LengthSquared | ( | ) | const |
Returns the squared length of the vector.
◆ Max()
T CubbyFlow::VectorN< T >::Max | ( | ) | const |
Returns the maximum element.
◆ Min()
T CubbyFlow::VectorN< T >::Min | ( | ) | const |
Returns the minimum element.
◆ Mul() [1/3]
VectorMul<T, VectorN, E> CubbyFlow::VectorN< T >::Mul | ( | const E & | v | ) | const |
Computes this * v.
◆ Mul() [2/3]
VectorScalarMul< T, VectorN< T > > CubbyFlow::VectorN< T >::Mul | ( | const T & | s | ) | const |
Computes this * (s, s, ... , s).
◆ Mul() [3/3]
VectorMul<T, VectorN<T>, E> CubbyFlow::VectorN< T >::Mul | ( | const E & | v | ) | const |
◆ Normalize()
void CubbyFlow::VectorN< T >::Normalize | ( | ) |
Normalizes this vector.
◆ Normalized()
VectorScalarDiv< T, VectorN< T > > CubbyFlow::VectorN< T >::Normalized | ( | ) | const |
Returns normalized vector.
◆ operator!=()
bool CubbyFlow::VectorN< T >::operator!= | ( | const E & | v | ) | const |
Returns true if other
is the not same as this vector.
◆ operator*=() [1/3]
VectorN< T > & CubbyFlow::VectorN< T >::operator*= | ( | const T & | s | ) |
Computes this *= (s, s, ... , s)
◆ operator*=() [2/3]
VectorN& CubbyFlow::VectorN< T >::operator*= | ( | const E & | v | ) |
Computes this *= v.
◆ operator*=() [3/3]
VectorN<T>& CubbyFlow::VectorN< T >::operator*= | ( | const E & | v | ) |
◆ operator+=() [1/3]
VectorN< T > & CubbyFlow::VectorN< T >::operator+= | ( | const T & | s | ) |
Computes this += (s, s, ... , s)
◆ operator+=() [2/3]
VectorN& CubbyFlow::VectorN< T >::operator+= | ( | const E & | v | ) |
Computes this += v.
◆ operator+=() [3/3]
VectorN<T>& CubbyFlow::VectorN< T >::operator+= | ( | const E & | v | ) |
◆ operator-=() [1/3]
VectorN< T > & CubbyFlow::VectorN< T >::operator-= | ( | const T & | s | ) |
Computes this -= (s, s, ... , s)
◆ operator-=() [2/3]
VectorN& CubbyFlow::VectorN< T >::operator-= | ( | const E & | v | ) |
Computes this -= v.
◆ operator-=() [3/3]
VectorN<T>& CubbyFlow::VectorN< T >::operator-= | ( | const E & | v | ) |
◆ operator/=() [1/3]
VectorN< T > & CubbyFlow::VectorN< T >::operator/= | ( | const T & | s | ) |
Computes this /= (s, s, ... , s)
◆ operator/=() [2/3]
VectorN& CubbyFlow::VectorN< T >::operator/= | ( | const E & | v | ) |
Computes this /= v.
◆ operator/=() [3/3]
VectorN<T>& CubbyFlow::VectorN< T >::operator/= | ( | const E & | v | ) |
◆ operator=() [1/6]
VectorN& CubbyFlow::VectorN< T >::operator= | ( | const std::initializer_list< U > & | list | ) |
Sets vector with given initializer list.
◆ operator=() [2/6]
VectorN& CubbyFlow::VectorN< T >::operator= | ( | const VectorExpression< T, E > & | other | ) |
Sets vector with expression template.
◆ operator=() [3/6]
VectorN< T > & CubbyFlow::VectorN< T >::operator= | ( | const VectorN< T > & | other | ) |
Copy assignment.
◆ operator=() [4/6]
|
noexcept |
Move assignment.
◆ operator=() [5/6]
VectorN<T>& CubbyFlow::VectorN< T >::operator= | ( | const std::initializer_list< U > & | list | ) |
◆ operator=() [6/6]
VectorN<T>& CubbyFlow::VectorN< T >::operator= | ( | const VectorExpression< T, E > & | other | ) |
◆ operator==()
bool CubbyFlow::VectorN< T >::operator== | ( | const E & | v | ) | const |
Returns true if other
is the same as this vector.
◆ operator[]() [1/2]
T CubbyFlow::VectorN< T >::operator[] | ( | size_t | i | ) | const |
Returns the i
-th element.
◆ operator[]() [2/2]
T & CubbyFlow::VectorN< T >::operator[] | ( | size_t | i | ) |
Returns the reference to the i
-th element.
◆ ParallelForEach()
void CubbyFlow::VectorN< T >::ParallelForEach | ( | Callback | func | ) |
Iterates the vector and invoke given func
for each element in parallel using multi-threading.
This function iterates the vector elements and invoke the callback function func
in parallel using multi-threading. The callback function takes vector's element as its input. The order of execution will be non-deterministic since it runs in parallel. Below is the sample usage:
The parameter type of the callback function doesn't have to be T&, but const T& or T can be used as well.
◆ ParallelForEachIndex()
void CubbyFlow::VectorN< T >::ParallelForEachIndex | ( | Callback | func | ) | const |
Iterates the vector and invoke given func
for each index in parallel using multi-threading.
This function iterates the vector elements and invoke the callback function func
in parallel using multi-threading. The callback function takes one parameter which is the index of the vector. The order of execution will be non-deterministic since it runs in parallel. Below is the sample usage:
◆ RDiv() [1/3]
VectorScalarRDiv< T, VectorN< T > > CubbyFlow::VectorN< T >::RDiv | ( | const T & | s | ) | const |
Computes (s, s, ... , s) / this.
◆ RDiv() [2/3]
VectorDiv<T, VectorN, E> CubbyFlow::VectorN< T >::RDiv | ( | const E & | v | ) | const |
Computes v / this.
◆ RDiv() [3/3]
VectorDiv<T, VectorN<T>, E> CubbyFlow::VectorN< T >::RDiv | ( | const E & | v | ) | const |
◆ Resize()
void CubbyFlow::VectorN< T >::Resize | ( | size_t | n, |
const T & | val = 0 |
||
) |
Resizes to n
dimensional vector with initial value val
.
◆ RSub() [1/3]
VectorScalarRSub< T, VectorN< T > > CubbyFlow::VectorN< T >::RSub | ( | const T & | s | ) | const |
Computes (s, s, ... , s) - this.
◆ RSub() [2/3]
VectorSub<T, VectorN, E> CubbyFlow::VectorN< T >::RSub | ( | const E & | v | ) | const |
Computes v - this.
◆ RSub() [3/3]
VectorSub<T, VectorN<T>, E> CubbyFlow::VectorN< T >::RSub | ( | const E & | v | ) | const |
◆ Set() [1/3]
void CubbyFlow::VectorN< T >::Set | ( | const T & | s | ) |
Sets all elements to s
.
◆ Set() [2/3]
void CubbyFlow::VectorN< T >::Set | ( | const std::initializer_list< U > & | list | ) |
Sets all elements with given initializer list.
◆ Set() [3/3]
void CubbyFlow::VectorN< T >::Set | ( | const VectorExpression< T, E > & | other | ) |
Sets vector with expression template.
◆ SetZero()
void CubbyFlow::VectorN< T >::SetZero | ( | ) |
Sets all elements to zero.
◆ size()
size_t CubbyFlow::VectorN< T >::size | ( | ) | const |
Returns the size of the vector.
◆ Sub() [1/3]
VectorSub<T, VectorN, E> CubbyFlow::VectorN< T >::Sub | ( | const E & | v | ) | const |
Computes this - v.
◆ Sub() [2/3]
VectorScalarSub< T, VectorN< T > > CubbyFlow::VectorN< T >::Sub | ( | const T & | s | ) | const |
Computes this - (s, s, ... , s).
◆ Sub() [3/3]
VectorSub<T, VectorN<T>, E> CubbyFlow::VectorN< T >::Sub | ( | const E & | v | ) | const |
◆ SubdominantAxis()
size_t CubbyFlow::VectorN< T >::SubdominantAxis | ( | ) | const |
Returns the index of the subdominant axis.
◆ Sum()
T CubbyFlow::VectorN< T >::Sum | ( | ) | const |
Returns the sum of all the elements.
◆ Swap()
void CubbyFlow::VectorN< T >::Swap | ( | VectorN< T > & | other | ) |
Swaps the content of the vector with other
vector.
The documentation for this class was generated from the following files:
- Core/Vector/VectorN.h
- Core/Vector/VectorN-Impl.h