3-D SPH particle system data. More...
#include <Core/SPH/SPHSystemData3.h>
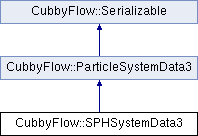
Public Member Functions | |
SPHSystemData3 () | |
Constructs empty SPH system. More... | |
SPHSystemData3 (size_t numberOfParticles) | |
Constructs SPH system data with given number of particles. More... | |
SPHSystemData3 (const SPHSystemData3 &other) | |
Copy constructor. More... | |
virtual | ~SPHSystemData3 () |
Destructor. More... | |
void | SetRadius (double newRadius) override |
Sets the radius. More... | |
void | SetMass (double newMass) override |
Sets the mass of a particle. More... | |
ConstArrayAccessor1< double > | GetDensities () const |
Returns the density array accessor (immutable). More... | |
ArrayAccessor1< double > | GetDensities () |
Returns the density array accessor (mutable). More... | |
ConstArrayAccessor1< double > | GetPressures () const |
Returns the pressure array accessor (immutable). More... | |
ArrayAccessor1< double > | GetPressures () |
Returns the pressure array accessor (mutable). More... | |
void | UpdateDensities () |
Updates the density array with the latest particle positions. More... | |
void | SetTargetDensity (double targetDensity) |
Sets the target density of this particle system. More... | |
double | GetTargetDensity () const |
Returns the target density of this particle system. More... | |
void | SetTargetSpacing (double spacing) |
Sets the target particle spacing in meters. More... | |
double | GetTargetSpacing () const |
Returns the target particle spacing in meters. More... | |
void | SetRelativeKernelRadius (double relativeRadius) |
Sets the relative kernel radius. More... | |
double | GetRelativeKernelRadius () const |
Returns the relative kernel radius. More... | |
void | SetKernelRadius (double kernelRadius) |
Sets the absolute kernel radius. More... | |
double | GetKernelRadius () const |
Returns the kernel radius in meters unit. More... | |
double | SumOfKernelNearby (const Vector3D &position) const |
Returns sum of kernel function evaluation for each nearby particle. More... | |
double | Interpolate (const Vector3D &origin, const ConstArrayAccessor1< double > &values) const |
Returns interpolated value at given origin point. More... | |
Vector3D | Interpolate (const Vector3D &origin, const ConstArrayAccessor1< Vector3D > &values) const |
Returns interpolated vector value at given origin point. More... | |
Vector3D | GradientAt (size_t i, const ConstArrayAccessor1< double > &values) const |
Returns the gradient of the given values at i-th particle. More... | |
double | LaplacianAt (size_t i, const ConstArrayAccessor1< double > &values) const |
Returns the Laplacian of the given values at i-th particle. More... | |
Vector3D | LaplacianAt (size_t i, const ConstArrayAccessor1< Vector3D > &values) const |
Returns the Laplacian of the given values at i-th particle. More... | |
void | BuildNeighborSearcher () |
Builds neighbor searcher with kernel radius. More... | |
void | BuildNeighborLists () |
Builds neighbor lists with kernel radius. More... | |
void | Serialize (std::vector< uint8_t > *buffer) const override |
Serializes this SPH system data to the buffer. More... | |
void | Deserialize (const std::vector< uint8_t > &buffer) override |
Deserializes this SPH system data from the buffer. More... | |
void | Set (const SPHSystemData3 &other) |
Copies from other SPH system data. More... | |
SPHSystemData3 & | operator= (const SPHSystemData3 &other) |
Copies from other SPH system data. More... | |
![]() | |
ParticleSystemData3 () | |
Default constructor. More... | |
ParticleSystemData3 (size_t numberOfParticles) | |
Constructs particle system data with given number of particles. More... | |
ParticleSystemData3 (const ParticleSystemData3 &other) | |
Copy constructor. More... | |
virtual | ~ParticleSystemData3 () |
Destructor. More... | |
void | Resize (size_t newNumberOfParticles) |
Resizes the number of particles of the container. More... | |
size_t | GetNumberOfParticles () const |
Returns the number of particles. More... | |
size_t | AddScalarData (double initialVal=0.0) |
Adds a scalar data layer and returns its index. More... | |
size_t | AddVectorData (const Vector3D &initialVal=Vector3D()) |
Adds a vector data layer and returns its index. More... | |
double | GetRadius () const |
Returns the radius of the particles. More... | |
double | GetMass () const |
Returns the mass of the particles. More... | |
ConstArrayAccessor1< Vector3D > | GetPositions () const |
Returns the position array (immutable). More... | |
ArrayAccessor1< Vector3D > | GetPositions () |
Returns the position array (mutable). More... | |
ConstArrayAccessor1< Vector3D > | GetVelocities () const |
Returns the velocity array (immutable). More... | |
ArrayAccessor1< Vector3D > | GetVelocities () |
Returns the velocity array (mutable). More... | |
ConstArrayAccessor1< Vector3D > | GetForces () const |
Returns the force array (immutable). More... | |
ArrayAccessor1< Vector3D > | GetForces () |
Returns the force array (mutable). More... | |
ConstArrayAccessor1< double > | ScalarDataAt (size_t idx) const |
Returns custom scalar data layer at given index (immutable). More... | |
ArrayAccessor1< double > | ScalarDataAt (size_t idx) |
Returns custom scalar data layer at given index (mutable). More... | |
ConstArrayAccessor1< Vector3D > | VectorDataAt (size_t idx) const |
Returns custom vector data layer at given index (immutable). More... | |
ArrayAccessor1< Vector3D > | VectorDataAt (size_t idx) |
Returns custom vector data layer at given index (mutable). More... | |
void | AddParticle (const Vector3D &newPosition, const Vector3D &newVelocity=Vector3D(), const Vector3D &newForce=Vector3D()) |
Adds a particle to the data structure. More... | |
void | AddParticles (const ConstArrayAccessor1< Vector3D > &newPositions, const ConstArrayAccessor1< Vector3D > &newVelocities=ConstArrayAccessor1< Vector3D >(), const ConstArrayAccessor1< Vector3D > &newForces=ConstArrayAccessor1< Vector3D >()) |
Adds particles to the data structure. More... | |
const PointNeighborSearcher3Ptr & | GetNeighborSearcher () const |
Returns neighbor searcher. More... | |
void | SetNeighborSearcher (const PointNeighborSearcher3Ptr &newNeighborSearcher) |
Sets neighbor searcher. More... | |
const std::vector< std::vector< size_t > > & | GetNeighborLists () const |
Returns neighbor lists. More... | |
void | BuildNeighborSearcher (double maxSearchRadius) |
Builds neighbor searcher with given search radius. More... | |
void | BuildNeighborLists (double maxSearchRadius) |
Builds neighbor lists with given search radius. More... | |
void | Set (const ParticleSystemData3 &other) |
Copies from other particle system data. More... | |
ParticleSystemData3 & | operator= (const ParticleSystemData3 &other) |
Copies from other particle system data. More... | |
Additional Inherited Members | |
![]() | |
using | ScalarData = Array1< double > |
Scalar data chunk. More... | |
using | VectorData = Array1< Vector3D > |
Vector data chunk. More... | |
![]() | |
void | SerializeParticleSystemData (flatbuffers::FlatBufferBuilder *builder, flatbuffers::Offset< fbs::ParticleSystemData3 > *fbsParticleSystemData) const |
void | DeserializeParticleSystemData (const fbs::ParticleSystemData3 *fbsParticleSystemData) |
Detailed Description
3-D SPH particle system data.
This class extends ParticleSystemData3 to specialize the data model for SPH. It includes density and pressure array as a default particle attribute, and it also contains SPH utilities such as interpolation operator.
Constructor & Destructor Documentation
◆ SPHSystemData3() [1/3]
CubbyFlow::SPHSystemData3::SPHSystemData3 | ( | ) |
Constructs empty SPH system.
◆ SPHSystemData3() [2/3]
|
explicit |
Constructs SPH system data with given number of particles.
◆ SPHSystemData3() [3/3]
CubbyFlow::SPHSystemData3::SPHSystemData3 | ( | const SPHSystemData3 & | other | ) |
Copy constructor.
◆ ~SPHSystemData3()
|
virtual |
Destructor.
Member Function Documentation
◆ BuildNeighborLists()
void CubbyFlow::SPHSystemData3::BuildNeighborLists | ( | ) |
Builds neighbor lists with kernel radius.
◆ BuildNeighborSearcher()
void CubbyFlow::SPHSystemData3::BuildNeighborSearcher | ( | ) |
Builds neighbor searcher with kernel radius.
◆ Deserialize()
|
overridevirtual |
Deserializes this SPH system data from the buffer.
Reimplemented from CubbyFlow::ParticleSystemData3.
◆ GetDensities() [1/2]
ConstArrayAccessor1<double> CubbyFlow::SPHSystemData3::GetDensities | ( | ) | const |
Returns the density array accessor (immutable).
◆ GetDensities() [2/2]
ArrayAccessor1<double> CubbyFlow::SPHSystemData3::GetDensities | ( | ) |
Returns the density array accessor (mutable).
◆ GetKernelRadius()
double CubbyFlow::SPHSystemData3::GetKernelRadius | ( | ) | const |
Returns the kernel radius in meters unit.
◆ GetPressures() [1/2]
ConstArrayAccessor1<double> CubbyFlow::SPHSystemData3::GetPressures | ( | ) | const |
Returns the pressure array accessor (immutable).
◆ GetPressures() [2/2]
ArrayAccessor1<double> CubbyFlow::SPHSystemData3::GetPressures | ( | ) |
Returns the pressure array accessor (mutable).
◆ GetRelativeKernelRadius()
double CubbyFlow::SPHSystemData3::GetRelativeKernelRadius | ( | ) | const |
Returns the relative kernel radius.
Returns the relative kernel radius compared to the target particle spacing (i.e. kernel radius / target spacing).
◆ GetTargetDensity()
double CubbyFlow::SPHSystemData3::GetTargetDensity | ( | ) | const |
Returns the target density of this particle system.
◆ GetTargetSpacing()
double CubbyFlow::SPHSystemData3::GetTargetSpacing | ( | ) | const |
Returns the target particle spacing in meters.
◆ GradientAt()
Vector3D CubbyFlow::SPHSystemData3::GradientAt | ( | size_t | i, |
const ConstArrayAccessor1< double > & | values | ||
) | const |
Returns the gradient of the given values at i-th particle.
◆ Interpolate() [1/2]
double CubbyFlow::SPHSystemData3::Interpolate | ( | const Vector3D & | origin, |
const ConstArrayAccessor1< double > & | values | ||
) | const |
Returns interpolated value at given origin point.
Returns interpolated scalar data from the given position using standard SPH weighted average. The data array should match the particle layout. For example, density or pressure arrays can be used.
◆ Interpolate() [2/2]
Vector3D CubbyFlow::SPHSystemData3::Interpolate | ( | const Vector3D & | origin, |
const ConstArrayAccessor1< Vector3D > & | values | ||
) | const |
Returns interpolated vector value at given origin point.
Returns interpolated vector data from the given position using standard SPH weighted average. The data array should match the particle layout. For example, velocity or acceleration arrays can be used.
◆ LaplacianAt() [1/2]
double CubbyFlow::SPHSystemData3::LaplacianAt | ( | size_t | i, |
const ConstArrayAccessor1< double > & | values | ||
) | const |
Returns the Laplacian of the given values at i-th particle.
◆ LaplacianAt() [2/2]
Vector3D CubbyFlow::SPHSystemData3::LaplacianAt | ( | size_t | i, |
const ConstArrayAccessor1< Vector3D > & | values | ||
) | const |
Returns the Laplacian of the given values at i-th particle.
◆ operator=()
SPHSystemData3& CubbyFlow::SPHSystemData3::operator= | ( | const SPHSystemData3 & | other | ) |
Copies from other SPH system data.
◆ Serialize()
|
overridevirtual |
Serializes this SPH system data to the buffer.
Reimplemented from CubbyFlow::ParticleSystemData3.
◆ Set()
void CubbyFlow::SPHSystemData3::Set | ( | const SPHSystemData3 & | other | ) |
Copies from other SPH system data.
◆ SetKernelRadius()
void CubbyFlow::SPHSystemData3::SetKernelRadius | ( | double | kernelRadius | ) |
Sets the absolute kernel radius.
Sets the absolute kernel radius compared to the target particle spacing (i.e. relative kernel radius * target spacing). Once this function is called, hash grid and density should be updated using UpdateHashGrid() and UpdateDensities).
◆ SetMass()
|
overridevirtual |
Sets the mass of a particle.
Setting the mass of a particle will change the target density.
- Parameters
-
[in] newMass The new mass.
Reimplemented from CubbyFlow::ParticleSystemData3.
◆ SetRadius()
|
overridevirtual |
Sets the radius.
Sets the radius of the particle system. The radius will be interpreted as target spacing.
Reimplemented from CubbyFlow::ParticleSystemData3.
◆ SetRelativeKernelRadius()
void CubbyFlow::SPHSystemData3::SetRelativeKernelRadius | ( | double | relativeRadius | ) |
Sets the relative kernel radius.
Sets the relative kernel radius compared to the target particle spacing (i.e. kernel radius / target spacing). Once this function is called, hash grid and density should be updated using UpdateHashGrid() and UpdateDensities).
◆ SetTargetDensity()
void CubbyFlow::SPHSystemData3::SetTargetDensity | ( | double | targetDensity | ) |
Sets the target density of this particle system.
◆ SetTargetSpacing()
void CubbyFlow::SPHSystemData3::SetTargetSpacing | ( | double | spacing | ) |
Sets the target particle spacing in meters.
Once this function is called, hash grid and density should be updated using UpdateHashGrid() and UpdateDensities).
◆ SumOfKernelNearby()
double CubbyFlow::SPHSystemData3::SumOfKernelNearby | ( | const Vector3D & | position | ) | const |
Returns sum of kernel function evaluation for each nearby particle.
◆ UpdateDensities()
void CubbyFlow::SPHSystemData3::UpdateDensities | ( | ) |
Updates the density array with the latest particle positions.
The documentation for this class was generated from the following file:
- Core/SPH/SPHSystemData3.h