Parallel version of hash grid-based 2-D point searcher. More...
#include <Core/Searcher/PointParallelHashGridSearcher2.h>
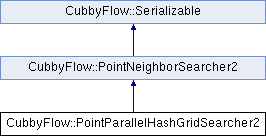
Classes | |
class | Builder |
Front-end to create PointParallelHashGridSearcher2 objects step by step. More... | |
Public Member Functions | |
PointParallelHashGridSearcher2 (const Size2 &resolution, double gridSpacing) | |
Constructs hash grid with given resolution and grid spacing. More... | |
PointParallelHashGridSearcher2 (size_t resolutionX, size_t resolutionY, double gridSpacing) | |
Constructs hash grid with given resolution and grid spacing. More... | |
PointParallelHashGridSearcher2 (const PointParallelHashGridSearcher2 &other) | |
Copy constructor. More... | |
void | Build (const ConstArrayAccessor1< Vector2D > &points) override |
Builds internal acceleration structure for given points list. More... | |
void | ForEachNearbyPoint (const Vector2D &origin, double radius, const ForEachNearbyPointFunc &callback) const override |
bool | HasNearbyPoint (const Vector2D &origin, double radius) const override |
const std::vector< size_t > & | Keys () const |
Returns the hash key list. More... | |
const std::vector< size_t > & | StartIndexTable () const |
Returns the start index table. More... | |
const std::vector< size_t > & | EndIndexTable () const |
Returns the end index table. More... | |
const std::vector< size_t > & | SortedIndices () const |
Returns the sorted indices of the points. More... | |
size_t | GetHashKeyFromBucketIndex (const Point2I &bucketIndex) const |
Point2I | GetBucketIndex (const Vector2D &position) const |
PointNeighborSearcher2Ptr | Clone () const override |
Creates a new instance of the object with same properties than original. More... | |
PointParallelHashGridSearcher2 & | operator= (const PointParallelHashGridSearcher2 &other) |
Assignment operator. More... | |
void | Set (const PointParallelHashGridSearcher2 &other) |
Copy from the other instance. More... | |
void | Serialize (std::vector< uint8_t > *buffer) const override |
Serializes the neighbor searcher into the buffer. More... | |
void | Deserialize (const std::vector< uint8_t > &buffer) override |
Deserializes the neighbor searcher from the buffer. More... | |
![]() | |
PointNeighborSearcher2 () | |
Default constructor. More... | |
virtual | ~PointNeighborSearcher2 () |
Destructor. More... | |
virtual std::string | TypeName () const =0 |
Returns the type name of the derived class. More... | |
Static Public Member Functions | |
static Builder | GetBuilder () |
Returns builder fox PointParallelHashGridSearcher2. More... | |
Additional Inherited Members | |
![]() | |
using | ForEachNearbyPointFunc = std::function< void(size_t, const Vector2D &)> |
Detailed Description
Parallel version of hash grid-based 2-D point searcher.
This class implements parallel version of 2-D point searcher by using hash grid for its internal acceleration data structure. Each point is recorded to its corresponding bucket where the hashing function is 2-D grid mapping.
Constructor & Destructor Documentation
◆ PointParallelHashGridSearcher2() [1/3]
CubbyFlow::PointParallelHashGridSearcher2::PointParallelHashGridSearcher2 | ( | const Size2 & | resolution, |
double | gridSpacing | ||
) |
Constructs hash grid with given resolution and grid spacing.
This constructor takes hash grid resolution and its grid spacing as its input parameters. The grid spacing must be 2x or greater than search radius.
- Parameters
-
[in] resolution The resolution. [in] gridSpacing The grid spacing.
◆ PointParallelHashGridSearcher2() [2/3]
CubbyFlow::PointParallelHashGridSearcher2::PointParallelHashGridSearcher2 | ( | size_t | resolutionX, |
size_t | resolutionY, | ||
double | gridSpacing | ||
) |
Constructs hash grid with given resolution and grid spacing.
This constructor takes hash grid resolution and its grid spacing as its input parameters. The grid spacing must be 2x or greater than search radius.
- Parameters
-
[in] resolutionX The resolution x. [in] resolutionY The resolution y. [in] gridSpacing The grid spacing.
◆ PointParallelHashGridSearcher2() [3/3]
CubbyFlow::PointParallelHashGridSearcher2::PointParallelHashGridSearcher2 | ( | const PointParallelHashGridSearcher2 & | other | ) |
Copy constructor.
Member Function Documentation
◆ Build()
|
overridevirtual |
Builds internal acceleration structure for given points list.
This function builds the hash grid for given points in parallel.
- Parameters
-
[in] points The points to be added.
Implements CubbyFlow::PointNeighborSearcher2.
◆ Clone()
|
overridevirtual |
Creates a new instance of the object with same properties than original.
- Returns
- Copy of this object.
Implements CubbyFlow::PointNeighborSearcher2.
◆ Deserialize()
|
overridevirtual |
Deserializes the neighbor searcher from the buffer.
Implements CubbyFlow::Serializable.
◆ EndIndexTable()
const std::vector<size_t>& CubbyFlow::PointParallelHashGridSearcher2::EndIndexTable | ( | ) | const |
Returns the end index table.
The end index table maps the hash grid bucket index to starting index of the sorted point list. Assume the hash key list looks like:
Then startIndexTable and endIndexTable should be like:
So that endIndexTable[i] - startIndexTable[i] is the number points in i-th table bucket.
- Returns
- The end index table.
◆ ForEachNearbyPoint()
|
overridevirtual |
Invokes the callback function for each nearby point around the origin within given radius.
- Parameters
-
[in] origin The origin position. [in] radius The search radius. [in] callback The callback function.
Implements CubbyFlow::PointNeighborSearcher2.
◆ GetBucketIndex()
Point2I CubbyFlow::PointParallelHashGridSearcher2::GetBucketIndex | ( | const Vector2D & | position | ) | const |
Gets the bucket index from a point.
- Parameters
-
[in] position The position of the point.
- Returns
- The bucket index.
◆ GetBuilder()
|
static |
Returns builder fox PointParallelHashGridSearcher2.
◆ GetHashKeyFromBucketIndex()
size_t CubbyFlow::PointParallelHashGridSearcher2::GetHashKeyFromBucketIndex | ( | const Point2I & | bucketIndex | ) | const |
Returns the hash value for given 2-D bucket index.
- Parameters
-
[in] bucketIndex The bucket index.
- Returns
- The hash key from bucket index.
◆ HasNearbyPoint()
|
overridevirtual |
Returns true if there are any nearby points for given origin within radius.
- Parameters
-
[in] origin The origin. [in] radius The radius.
- Returns
- True if has nearby point, false otherwise.
Implements CubbyFlow::PointNeighborSearcher2.
◆ Keys()
const std::vector<size_t>& CubbyFlow::PointParallelHashGridSearcher2::Keys | ( | ) | const |
Returns the hash key list.
The hash key list maps sorted point index i to its hash key value. The sorting order is based on the key value itself.
- Returns
- The hash key list.
◆ operator=()
PointParallelHashGridSearcher2& CubbyFlow::PointParallelHashGridSearcher2::operator= | ( | const PointParallelHashGridSearcher2 & | other | ) |
Assignment operator.
◆ Serialize()
|
overridevirtual |
Serializes the neighbor searcher into the buffer.
Implements CubbyFlow::Serializable.
◆ Set()
void CubbyFlow::PointParallelHashGridSearcher2::Set | ( | const PointParallelHashGridSearcher2 & | other | ) |
Copy from the other instance.
◆ SortedIndices()
const std::vector<size_t>& CubbyFlow::PointParallelHashGridSearcher2::SortedIndices | ( | ) | const |
Returns the sorted indices of the points.
When the hash grid is built, it sorts the points in hash key order. But rather than sorting the original points, this class keeps the shuffled indices of the points. The list this function returns maps sorted index i to original index j.
- Returns
- The sorted indices of the points.
◆ StartIndexTable()
const std::vector<size_t>& CubbyFlow::PointParallelHashGridSearcher2::StartIndexTable | ( | ) | const |
Returns the start index table.
The start index table maps the hash grid bucket index to starting index of the sorted point list. Assume the hash key list looks like:
Then startIndexTable and endIndexTable should be like:
So that endIndexTable[i] - startIndexTable[i] is the number points in i-th table bucket.
- Returns
- The start index table.
The documentation for this class was generated from the following file:
- Core/Searcher/PointParallelHashGridSearcher2.h