Basic 2-D particle system solver. More...
#include <Core/Solver/Particle/ParticleSystemSolver2.h>
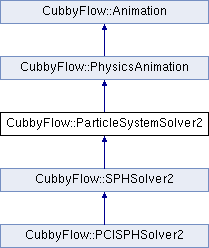
Classes | |
class | Builder |
Front-end to create ParticleSystemSolver2 objects step by step. More... | |
Public Member Functions | |
ParticleSystemSolver2 () | |
Constructs an empty solver. More... | |
ParticleSystemSolver2 (double radius, double mass) | |
Constructs a solver with particle parameters. More... | |
virtual | ~ParticleSystemSolver2 () |
Destructor. More... | |
double | GetDragCoefficient () const |
Returns the drag coefficient. More... | |
void | SetDragCoefficient (double newDragCoefficient) |
Sets the drag coefficient. More... | |
double | GetRestitutionCoefficient () const |
Gets the restitution coefficient. More... | |
void | SetRestitutionCoefficient (double newRestitutionCoefficient) |
Sets the restitution coefficient. More... | |
const Vector2D & | GetGravity () const |
Returns the gravity. More... | |
void | SetGravity (const Vector2D &newGravity) |
Sets the gravity. More... | |
const ParticleSystemData2Ptr & | GetParticleSystemData () const |
Returns the particle system data. More... | |
const Collider2Ptr & | GetCollider () const |
Returns the collider. More... | |
void | SetCollider (const Collider2Ptr &newCollider) |
Sets the collider. More... | |
const ParticleEmitter2Ptr & | GetEmitter () const |
Returns the emitter. More... | |
void | SetEmitter (const ParticleEmitter2Ptr &newEmitter) |
Sets the emitter. More... | |
const VectorField2Ptr & | GetWind () const |
Returns the wind field. More... | |
void | SetWind (const VectorField2Ptr &newWind) |
Sets the wind. More... | |
![]() | |
PhysicsAnimation () | |
Default constructor. More... | |
virtual | ~PhysicsAnimation () |
Destructor. More... | |
bool | GetIsUsingFixedSubTimeSteps () const |
Returns true if fixed sub-timestepping is used. More... | |
void | SetIsUsingFixedSubTimeSteps (bool isUsing) |
Sets true if fixed sub-timestepping is used. More... | |
unsigned int | GetNumberOfFixedSubTimeSteps () const |
Returns the number of fixed sub-timesteps. More... | |
void | SetNumberOfFixedSubTimeSteps (unsigned int numberOfSteps) |
Sets the number of fixed sub-timesteps. More... | |
void | AdvanceSingleFrame () |
Advances a single frame. More... | |
Frame | GetCurrentFrame () const |
Returns current frame. More... | |
void | SetCurrentFrame (const Frame &frame) |
Sets current frame cursor (but do not invoke update()). More... | |
double | GetCurrentTimeInSeconds () const |
Returns current time in seconds. More... | |
![]() | |
Animation () | |
virtual | ~Animation () |
void | Update (const Frame &frame) |
Updates animation state for given frame . More... | |
Static Public Member Functions | |
static Builder | GetBuilder () |
Returns builder fox ParticleSystemSolver2. More... | |
Protected Member Functions | |
void | OnInitialize () override |
Initializes the simulator. More... | |
void | OnAdvanceTimeStep (double timeStepInSeconds) override |
Called to advance a single time-step. More... | |
virtual void | AccumulateForces (double timeStepInSeconds) |
Accumulates forces applied to the particles. More... | |
virtual void | OnBeginAdvanceTimeStep (double timeStepInSeconds) |
Called when a time-step is about to begin. More... | |
virtual void | OnEndAdvanceTimeStep (double timeStepInSeconds) |
Called after a time-step is completed. More... | |
void | ResolveCollision () |
Resolves any collisions occurred by the particles. More... | |
void | ResolveCollision (ArrayAccessor1< Vector2D > newPositions, ArrayAccessor1< Vector2D > newVelocities) |
void | SetParticleSystemData (const ParticleSystemData2Ptr &newParticles) |
Assign a new particle system data. More... | |
![]() | |
virtual unsigned int | GetNumberOfSubTimeSteps (double timeIntervalInSeconds) const |
Returns the required number of sub-timesteps for given time interval. More... | |
Detailed Description
Basic 2-D particle system solver.
This class implements basic particle system solver. It includes gravity, air drag, and collision. But it does not compute particle-to-particle interaction. Thus, this solver is suitable for performing simple spray-like simulations with low computational cost. This class can be further extend to add more sophisticated simulations, such as SPH, to handle particle-to-particle intersection.
- See also
- SphSolver2
Constructor & Destructor Documentation
◆ ParticleSystemSolver2() [1/2]
CubbyFlow::ParticleSystemSolver2::ParticleSystemSolver2 | ( | ) |
Constructs an empty solver.
◆ ParticleSystemSolver2() [2/2]
CubbyFlow::ParticleSystemSolver2::ParticleSystemSolver2 | ( | double | radius, |
double | mass | ||
) |
Constructs a solver with particle parameters.
◆ ~ParticleSystemSolver2()
|
virtual |
Destructor.
Member Function Documentation
◆ AccumulateForces()
|
protectedvirtual |
Accumulates forces applied to the particles.
Reimplemented in CubbyFlow::SPHSolver2.
◆ GetBuilder()
|
static |
Returns builder fox ParticleSystemSolver2.
◆ GetCollider()
const Collider2Ptr& CubbyFlow::ParticleSystemSolver2::GetCollider | ( | ) | const |
Returns the collider.
◆ GetDragCoefficient()
double CubbyFlow::ParticleSystemSolver2::GetDragCoefficient | ( | ) | const |
Returns the drag coefficient.
◆ GetEmitter()
const ParticleEmitter2Ptr& CubbyFlow::ParticleSystemSolver2::GetEmitter | ( | ) | const |
Returns the emitter.
◆ GetGravity()
const Vector2D& CubbyFlow::ParticleSystemSolver2::GetGravity | ( | ) | const |
Returns the gravity.
◆ GetParticleSystemData()
const ParticleSystemData2Ptr& CubbyFlow::ParticleSystemSolver2::GetParticleSystemData | ( | ) | const |
Returns the particle system data.
This function returns the particle system data. The data is created when this solver is constructed and also owned by the solver.
- Returns
- The particle system data.
◆ GetRestitutionCoefficient()
double CubbyFlow::ParticleSystemSolver2::GetRestitutionCoefficient | ( | ) | const |
Gets the restitution coefficient.
◆ GetWind()
const VectorField2Ptr& CubbyFlow::ParticleSystemSolver2::GetWind | ( | ) | const |
Returns the wind field.
◆ OnAdvanceTimeStep()
|
overrideprotectedvirtual |
Called to advance a single time-step.
Implements CubbyFlow::PhysicsAnimation.
◆ OnBeginAdvanceTimeStep()
|
protectedvirtual |
Called when a time-step is about to begin.
Reimplemented in CubbyFlow::SPHSolver2, and CubbyFlow::PCISPHSolver2.
◆ OnEndAdvanceTimeStep()
|
protectedvirtual |
Called after a time-step is completed.
Reimplemented in CubbyFlow::SPHSolver2.
◆ OnInitialize()
|
overrideprotectedvirtual |
Initializes the simulator.
Reimplemented from CubbyFlow::PhysicsAnimation.
◆ ResolveCollision() [1/2]
|
protected |
Resolves any collisions occurred by the particles.
◆ ResolveCollision() [2/2]
|
protected |
Resolves any collisions occurred by the particles where the particle state is given by the position and velocity arrays.
◆ SetCollider()
void CubbyFlow::ParticleSystemSolver2::SetCollider | ( | const Collider2Ptr & | newCollider | ) |
Sets the collider.
◆ SetDragCoefficient()
void CubbyFlow::ParticleSystemSolver2::SetDragCoefficient | ( | double | newDragCoefficient | ) |
Sets the drag coefficient.
The drag coefficient controls the amount of air-drag. The coefficient should be a positive number and 0 means no drag force.
- Parameters
-
[in] newDragCoefficient The new drag coefficient.
◆ SetEmitter()
void CubbyFlow::ParticleSystemSolver2::SetEmitter | ( | const ParticleEmitter2Ptr & | newEmitter | ) |
Sets the emitter.
◆ SetGravity()
void CubbyFlow::ParticleSystemSolver2::SetGravity | ( | const Vector2D & | newGravity | ) |
Sets the gravity.
◆ SetParticleSystemData()
|
protected |
Assign a new particle system data.
◆ SetRestitutionCoefficient()
void CubbyFlow::ParticleSystemSolver2::SetRestitutionCoefficient | ( | double | newRestitutionCoefficient | ) |
Sets the restitution coefficient.
The restitution coefficient controls the bounciness of a particle when it hits a collider surface. The range of the coefficient should be 0 to 1 – 0 means no bounce back and 1 means perfect reflection.
- Parameters
-
[in] newRestitutionCoefficient The new restitution coefficient.
◆ SetWind()
void CubbyFlow::ParticleSystemSolver2::SetWind | ( | const VectorField2Ptr & | newWind | ) |
Sets the wind.
Wind can be applied to the particle system by setting a vector field to the solver.
- Parameters
-
[in] newWind The new wind.
The documentation for this class was generated from the following file:
- Core/Solver/Particle/ParticleSystemSolver2.h