3-D PCISPH solver. More...
#include <Core/Solver/Particle/PCISPH/PCISPHSolver3.h>
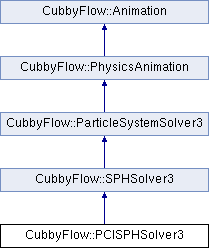
Classes | |
class | Builder |
Front-end to create PCISPHSolver3 objects step by step. More... | |
Public Member Functions | |
PCISPHSolver3 () | |
Constructs a solver with empty particle set. More... | |
PCISPHSolver3 (double targetDensity, double targetSpacing, double relativeKernelRadius) | |
Constructs a solver with target density, spacing, and relative kernel radius. More... | |
virtual | ~PCISPHSolver3 () |
double | GetMaxDensityErrorRatio () const |
Returns max allowed density error ratio. More... | |
void | SetMaxDensityErrorRatio (double ratio) |
Sets max allowed density error ratio. More... | |
unsigned int | GetMaxNumberOfIterations () const |
Returns max number of iterations. More... | |
void | SetMaxNumberOfIterations (unsigned int n) |
Sets max number of PCISPH iterations. More... | |
![]() | |
SPHSolver3 () | |
Constructs a solver with empty particle set. More... | |
SPHSolver3 (double targetDensity, double targetSpacing, double relativeKernelRadius) | |
virtual | ~SPHSolver3 () |
double | GetEosExponent () const |
Returns the exponent part of the equation-of-state. More... | |
void | SetEosExponent (double newEosExponent) |
Sets the exponent part of the equation-of-state. More... | |
double | GetNegativePressureScale () const |
Returns the negative pressure scale. More... | |
void | SetNegativePressureScale (double newNegativePressureScale) |
Sets the negative pressure scale. More... | |
double | GetViscosityCoefficient () const |
Returns the viscosity coefficient. More... | |
void | SetViscosityCoefficient (double newViscosityCoefficient) |
Sets the viscosity coefficient. More... | |
double | GetPseudoViscosityCoefficient () const |
Returns the pseudo viscosity coefficient. More... | |
void | SetPseudoViscosityCoefficient (double newPseudoViscosityCoefficient) |
Sets the pseudo viscosity coefficient. More... | |
double | GetSpeedOfSound () const |
Returns the speed of sound. More... | |
void | SetSpeedOfSound (double newSpeedOfSound) |
Sets the speed of sound. More... | |
double | GetTimeStepLimitScale () const |
Multiplier that scales the max allowed time-step. More... | |
void | SetTimeStepLimitScale (double newScale) |
Sets the multiplier that scales the max allowed time-step. More... | |
SPHSystemData3Ptr | GetSPHSystemData () const |
Returns the SPH system data. More... | |
![]() | |
ParticleSystemSolver3 () | |
Constructs an empty solver. More... | |
ParticleSystemSolver3 (double radius, double mass) | |
Constructs a solver with particle parameters. More... | |
virtual | ~ParticleSystemSolver3 () |
Destructor. More... | |
double | GetDragCoefficient () const |
Returns the drag coefficient. More... | |
void | SetDragCoefficient (double newDragCoefficient) |
Sets the drag coefficient. More... | |
double | GetRestitutionCoefficient () const |
Gets the restitution coefficient. More... | |
void | SetRestitutionCoefficient (double newRestitutionCoefficient) |
Sets the restitution coefficient. More... | |
const Vector3D & | GetGravity () const |
Returns the gravity. More... | |
void | SetGravity (const Vector3D &newGravity) |
Sets the gravity. More... | |
const ParticleSystemData3Ptr & | GetParticleSystemData () const |
Returns the particle system data. More... | |
const Collider3Ptr & | GetCollider () const |
Returns the collider. More... | |
void | SetCollider (const Collider3Ptr &newCollider) |
Sets the collider. More... | |
const ParticleEmitter3Ptr & | GetEmitter () const |
Returns the emitter. More... | |
void | SetEmitter (const ParticleEmitter3Ptr &newEmitter) |
Sets the emitter. More... | |
const VectorField3Ptr & | GetWind () const |
Returns the wind field. More... | |
void | SetWind (const VectorField3Ptr &newWind) |
Sets the wind. More... | |
![]() | |
PhysicsAnimation () | |
Default constructor. More... | |
virtual | ~PhysicsAnimation () |
Destructor. More... | |
bool | GetIsUsingFixedSubTimeSteps () const |
Returns true if fixed sub-timestepping is used. More... | |
void | SetIsUsingFixedSubTimeSteps (bool isUsing) |
Sets true if fixed sub-timestepping is used. More... | |
unsigned int | GetNumberOfFixedSubTimeSteps () const |
Returns the number of fixed sub-timesteps. More... | |
void | SetNumberOfFixedSubTimeSteps (unsigned int numberOfSteps) |
Sets the number of fixed sub-timesteps. More... | |
void | AdvanceSingleFrame () |
Advances a single frame. More... | |
Frame | GetCurrentFrame () const |
Returns current frame. More... | |
void | SetCurrentFrame (const Frame &frame) |
Sets current frame cursor (but do not invoke update()). More... | |
double | GetCurrentTimeInSeconds () const |
Returns current time in seconds. More... | |
![]() | |
Animation () | |
virtual | ~Animation () |
void | Update (const Frame &frame) |
Updates animation state for given frame . More... | |
Static Public Member Functions | |
static Builder | GetBuilder () |
Returns builder fox PCISPHSolver3. More... | |
![]() | |
static Builder | GetBuilder () |
Returns builder fox SPHSolver3. More... | |
![]() | |
static Builder | GetBuilder () |
Returns builder fox ParticleSystemSolver3. More... | |
Protected Member Functions | |
void | AccumulatePressureForce (double timeIntervalInSeconds) override |
Accumulates the pressure force to the forces array in the particle system. More... | |
void | OnBeginAdvanceTimeStep (double timeStepInSeconds) override |
Performs pre-processing step before the simulation. More... | |
![]() | |
unsigned int | GetNumberOfSubTimeSteps (double timeIntervalInSeconds) const override |
Returns the number of sub-time-steps. More... | |
void | AccumulateForces (double timeStepInSeconds) override |
Accumulates the force to the forces array in the particle system. More... | |
void | OnBeginAdvanceTimeStep (double timeStepInSeconds) override |
Performs pre-processing step before the simulation. More... | |
void | OnEndAdvanceTimeStep (double timeStepInSeconds) override |
Performs post-processing step before the simulation. More... | |
virtual void | AccumulateNonPressureForces (double timeStepInSeconds) |
void | ComputePressure () |
Computes the pressure. More... | |
void | AccumulatePressureForce (const ConstArrayAccessor1< Vector3D > &positions, const ConstArrayAccessor1< double > &densities, const ConstArrayAccessor1< double > &pressures, ArrayAccessor1< Vector3D > pressureForces) |
Accumulates the pressure force to the given pressureForces array. More... | |
void | AccumulateViscosityForce () |
void | ComputePseudoViscosity (double timeStepInSeconds) |
Computes pseudo viscosity. More... | |
![]() | |
void | OnInitialize () override |
Initializes the simulator. More... | |
void | OnAdvanceTimeStep (double timeStepInSeconds) override |
Called to advance a single time-step. More... | |
void | ResolveCollision () |
Resolves any collisions occurred by the particles. More... | |
void | ResolveCollision (ArrayAccessor1< Vector3D > newPositions, ArrayAccessor1< Vector3D > newVelocities) |
void | SetParticleSystemData (const ParticleSystemData3Ptr &newParticles) |
Assign a new particle system data. More... | |
Detailed Description
3-D PCISPH solver.
This class implements 3-D predictive-corrective SPH solver. The main pressure solver is based on Solenthaler and Pajarola's 2009 SIGGRAPH paper.
- See also
- Solenthaler and Pajarola, Predictive-corrective incompressible SPH, ACM transactions on graphics (TOG). Vol. 28. No. 3. ACM, 2009.
Constructor & Destructor Documentation
◆ PCISPHSolver3() [1/2]
CubbyFlow::PCISPHSolver3::PCISPHSolver3 | ( | ) |
Constructs a solver with empty particle set.
◆ PCISPHSolver3() [2/2]
CubbyFlow::PCISPHSolver3::PCISPHSolver3 | ( | double | targetDensity, |
double | targetSpacing, | ||
double | relativeKernelRadius | ||
) |
Constructs a solver with target density, spacing, and relative kernel radius.
◆ ~PCISPHSolver3()
|
virtual |
Member Function Documentation
◆ AccumulatePressureForce()
|
overrideprotectedvirtual |
Accumulates the pressure force to the forces array in the particle system.
Reimplemented from CubbyFlow::SPHSolver3.
◆ GetBuilder()
|
static |
Returns builder fox PCISPHSolver3.
◆ GetMaxDensityErrorRatio()
double CubbyFlow::PCISPHSolver3::GetMaxDensityErrorRatio | ( | ) | const |
Returns max allowed density error ratio.
◆ GetMaxNumberOfIterations()
unsigned int CubbyFlow::PCISPHSolver3::GetMaxNumberOfIterations | ( | ) | const |
Returns max number of iterations.
◆ OnBeginAdvanceTimeStep()
|
overrideprotectedvirtual |
Performs pre-processing step before the simulation.
Reimplemented from CubbyFlow::ParticleSystemSolver3.
◆ SetMaxDensityErrorRatio()
void CubbyFlow::PCISPHSolver3::SetMaxDensityErrorRatio | ( | double | ratio | ) |
Sets max allowed density error ratio.
This function sets the max allowed density error ratio during the PCISPH iteration. Default is 0.01 (1%). The input value should be positive.
◆ SetMaxNumberOfIterations()
void CubbyFlow::PCISPHSolver3::SetMaxNumberOfIterations | ( | unsigned int | n | ) |
Sets max number of PCISPH iterations.
This function sets the max number of PCISPH iterations. Default is 5.
The documentation for this class was generated from the following file:
- Core/Solver/Particle/PCISPH/PCISPHSolver3.h