Compressed Sparse Row (CSR) matrix class. More...
#include <Core/Matrix/MatrixCSR.h>
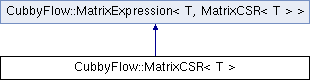
Classes | |
struct | Element |
Public Types | |
using | NonZeroContainerType = std::vector< T > |
using | NonZeroIterator = typename NonZeroContainerType::iterator |
using | ConstNonZeroIterator = typename NonZeroContainerType::const_iterator |
using | IndexContainerType = std::vector< size_t > |
using | IndexIterator = IndexContainerType::iterator |
using | ConstIndexIterator = IndexContainerType::const_iterator |
Public Member Functions | |
MatrixCSR () | |
Constructs an empty matrix. More... | |
MatrixCSR (const std::initializer_list< std::initializer_list< T >> &list, T epsilon=std::numeric_limits< T >::epsilon()) | |
Compresses given initializer list list into a sparse matrix. More... | |
template<typename E > | |
MatrixCSR (const MatrixExpression< T, E > &other, T epsilon=std::numeric_limits< T >::epsilon()) | |
Compresses input (dense) matrix expression into a sparse matrix. More... | |
MatrixCSR (const MatrixCSR &other) | |
Copy constructor. More... | |
MatrixCSR (MatrixCSR &&other) noexcept | |
Move constructor. More... | |
void | Clear () |
Clears the matrix and make it zero-dimensional. More... | |
void | Set (const T &s) |
Sets whole matrix with input scalar. More... | |
void | Set (const MatrixCSR &other) |
Copy from given sparse matrix. More... | |
void | Reserve (size_t rows, size_t cols, size_t numNonZeros) |
Reserves memory space of this matrix. More... | |
void | Compress (const std::initializer_list< std::initializer_list< T >> &list, T epsilon=std::numeric_limits< T >::epsilon()) |
Compresses given initializer list list into a sparse matrix. More... | |
template<typename E > | |
void | Compress (const MatrixExpression< T, E > &other, T epsilon=std::numeric_limits< T >::epsilon()) |
Compresses input (dense) matrix expression into a sparse matrix. More... | |
void | AddElement (size_t i, size_t j, const T &value) |
Adds non-zero element to (i, j). More... | |
void | AddElement (const Element &element) |
Adds non-zero element. More... | |
void | AddRow (const NonZeroContainerType &nonZeros, const IndexContainerType &columnIndices) |
void | SetElement (size_t i, size_t j, const T &value) |
Sets non-zero element to (i, j). More... | |
void | SetElement (const Element &element) |
Sets non-zero element. More... | |
bool | IsEqual (const MatrixCSR &other) const |
bool | IsSimilar (const MatrixCSR &other, double tol=std::numeric_limits< double >::epsilon()) const |
Returns true if this matrix is similar to the input matrix within the given tolerance. More... | |
bool | IsSquare () const |
Returns true if this matrix is a square matrix. More... | |
Size2 | size () const |
Returns the size of this matrix. More... | |
size_t | Rows () const |
Returns number of rows of this matrix. More... | |
size_t | Cols () const |
Returns number of columns of this matrix. More... | |
size_t | NumberOfNonZeros () const |
Returns the number of non-zero elements. More... | |
const T & | NonZero (size_t i) const |
Returns i-th non-zero element. More... | |
T & | NonZero (size_t i) |
Returns i-th non-zero element. More... | |
const size_t & | RowPointer (size_t i) const |
Returns i-th row pointer. More... | |
const size_t & | ColumnIndex (size_t i) const |
Returns i-th column index. More... | |
T * | NonZeroData () |
Returns pointer of the non-zero elements data. More... | |
const T * | NonZeroData () const |
Returns constant pointer of the non-zero elements data. More... | |
const size_t * | RowPointersData () const |
Returns constant pointer of the row pointers data. More... | |
const size_t * | ColumnIndicesData () const |
Returns constant pointer of the column indices data. More... | |
NonZeroIterator | NonZeroBegin () |
Returns the begin iterator of the non-zero elements. More... | |
ConstNonZeroIterator | NonZeroBegin () const |
Returns the begin const iterator of the non-zero elements. More... | |
NonZeroIterator | NonZeroEnd () |
Returns the end iterator of the non-zero elements. More... | |
ConstNonZeroIterator | NonZeroEnd () const |
Returns the end const iterator of the non-zero elements. More... | |
IndexIterator | RowPointersBegin () |
Returns the begin iterator of the row pointers. More... | |
ConstIndexIterator | RowPointersBegin () const |
Returns the begin const iterator of the row pointers. More... | |
IndexIterator | RowPointersEnd () |
Returns the end iterator of the row pointers. More... | |
ConstIndexIterator | RowPointersEnd () const |
Returns the end const iterator of the row pointers. More... | |
IndexIterator | ColumnIndicesBegin () |
Returns the begin iterator of the column indices. More... | |
ConstIndexIterator | ColumnIndicesBegin () const |
Returns the begin const iterator of the column indices. More... | |
IndexIterator | ColumnIndicesEnd () |
Returns the end iterator of the column indices. More... | |
ConstIndexIterator | ColumnIndicesEnd () const |
Returns the end const iterator of the column indices. More... | |
MatrixCSR | Add (const T &s) const |
Returns this matrix + input scalar. More... | |
MatrixCSR | Add (const MatrixCSR &m) const |
Returns this matrix + input matrix (element-wise). More... | |
MatrixCSR | Sub (const T &s) const |
Returns this matrix - input scalar. More... | |
MatrixCSR | Sub (const MatrixCSR &m) const |
Returns this matrix - input matrix (element-wise). More... | |
MatrixCSR | Mul (const T &s) const |
Returns this matrix * input scalar. More... | |
template<typename VE > | |
MatrixCSRVectorMul< T, VE > | Mul (const VectorExpression< T, VE > &v) const |
Returns this matrix * input vector. More... | |
template<typename ME > | |
MatrixCSRMatrixMul< T, ME > | Mul (const MatrixExpression< T, ME > &m) const |
Returns this matrix * input matrix. More... | |
MatrixCSR | Div (const T &s) const |
Returns this matrix / input scalar. More... | |
MatrixCSR | RAdd (const T &s) const |
Returns input scalar + this matrix. More... | |
MatrixCSR | RAdd (const MatrixCSR &m) const |
Returns input matrix + this matrix (element-wise). More... | |
MatrixCSR | RSub (const T &s) const |
Returns input scalar - this matrix. More... | |
MatrixCSR | RSub (const MatrixCSR &m) const |
Returns input matrix - this matrix (element-wise). More... | |
MatrixCSR | RMul (const T &s) const |
Returns input scalar * this matrix. More... | |
MatrixCSR | RDiv (const T &s) const |
Returns input matrix / this scalar. More... | |
void | IAdd (const T &s) |
Adds input scalar to this matrix. More... | |
void | IAdd (const MatrixCSR &m) |
Adds input matrix to this matrix (element-wise). More... | |
void | ISub (const T &s) |
Subtracts input scalar from this matrix. More... | |
void | ISub (const MatrixCSR &m) |
Subtracts input matrix from this matrix (element-wise). More... | |
void | IMul (const T &s) |
Multiplies input scalar to this matrix. More... | |
template<typename ME > | |
void | IMul (const MatrixExpression< T, ME > &m) |
Multiplies input matrix to this matrix. More... | |
void | IDiv (const T &s) |
Divides this matrix with input scalar. More... | |
T | Sum () const |
Returns sum of all elements. More... | |
T | Avg () const |
Returns average of all elements. More... | |
T | Min () const |
Returns minimum among all elements. More... | |
T | Max () const |
Returns maximum among all elements. More... | |
T | AbsMin () const |
Returns absolute minimum among all elements. More... | |
T | AbsMax () const |
Returns absolute maximum among all elements. More... | |
T | Trace () const |
template<typename U > | |
MatrixCSR< U > | CastTo () const |
Type-casts to different value-typed matrix. More... | |
template<typename E > | |
MatrixCSR & | operator= (const E &m) |
Compresses input (dense) matrix expression into a sparse matrix. More... | |
MatrixCSR & | operator= (const MatrixCSR &other) |
Copies to this matrix. More... | |
MatrixCSR & | operator= (MatrixCSR &&other) noexcept |
Moves to this matrix. More... | |
MatrixCSR & | operator+= (const T &s) |
Addition assignment with input scalar. More... | |
MatrixCSR & | operator+= (const MatrixCSR &m) |
Addition assignment with input matrix (element-wise). More... | |
MatrixCSR & | operator-= (const T &s) |
Subtraction assignment with input scalar. More... | |
MatrixCSR & | operator-= (const MatrixCSR &m) |
Subtraction assignment with input matrix (element-wise). More... | |
MatrixCSR & | operator*= (const T &s) |
Multiplication assignment with input scalar. More... | |
template<typename ME > | |
MatrixCSR & | operator*= (const MatrixExpression< T, ME > &m) |
Multiplication assignment with input matrix. More... | |
MatrixCSR & | operator/= (const T &s) |
Division assignment with input scalar. More... | |
T | operator() (size_t i, size_t j) const |
Returns (i,j) element. More... | |
bool | operator== (const MatrixCSR &m) const |
Returns true if is equal to m. More... | |
bool | operator!= (const MatrixCSR &m) const |
Returns true if is not equal to m. More... | |
template<typename E > | |
MatrixCSR< T > & | operator= (const E &m) |
template<typename ME > | |
MatrixCSR< T > & | operator*= (const MatrixExpression< T, ME > &m) |
template<typename Op > | |
MatrixCSR< T > | BinaryOp (const MatrixCSR &m, Op op) const |
![]() | |
Size2 | size () const |
Size of the matrix. More... | |
size_t | Rows () const |
Number of rows. More... | |
size_t | Cols () const |
Number of columns. More... | |
const MatrixCSR< T > & | operator() () const |
Returns actual implementation (the subclass). More... | |
Static Public Member Functions | |
static MatrixCSR< T > | MakeIdentity (size_t m) |
Makes a m x m matrix with all diagonal elements to 1, and other elements to 0. More... | |
Detailed Description
template<typename T>
class CubbyFlow::MatrixCSR< T >
Compressed Sparse Row (CSR) matrix class.
This class defines Compressed Sparse Row (CSR) matrix using arrays of non-zero elements, row pointers, and column indices.
- Template Parameters
-
T Type of the element.
Member Typedef Documentation
◆ ConstIndexIterator
using CubbyFlow::MatrixCSR< T >::ConstIndexIterator = IndexContainerType::const_iterator |
◆ ConstNonZeroIterator
using CubbyFlow::MatrixCSR< T >::ConstNonZeroIterator = typename NonZeroContainerType::const_iterator |
◆ IndexContainerType
using CubbyFlow::MatrixCSR< T >::IndexContainerType = std::vector<size_t> |
◆ IndexIterator
using CubbyFlow::MatrixCSR< T >::IndexIterator = IndexContainerType::iterator |
◆ NonZeroContainerType
using CubbyFlow::MatrixCSR< T >::NonZeroContainerType = std::vector<T> |
◆ NonZeroIterator
using CubbyFlow::MatrixCSR< T >::NonZeroIterator = typename NonZeroContainerType::iterator |
Constructor & Destructor Documentation
◆ MatrixCSR() [1/5]
CubbyFlow::MatrixCSR< T >::MatrixCSR | ( | ) |
Constructs an empty matrix.
◆ MatrixCSR() [2/5]
CubbyFlow::MatrixCSR< T >::MatrixCSR | ( | const std::initializer_list< std::initializer_list< T >> & | list, |
T | epsilon = std::numeric_limits<T>::epsilon() |
||
) |
Compresses given initializer list list
into a sparse matrix.
This constructor will build a matrix with given initializer list list
such as
Note the initializer has 4x3 structure which will create 4x3 matrix. During the process, zero elements (less than epsilon
) will not be stored.
- Parameters
-
list Initializer list that should be copy to the new matrix.
◆ MatrixCSR() [3/5]
CubbyFlow::MatrixCSR< T >::MatrixCSR | ( | const MatrixExpression< T, E > & | other, |
T | epsilon = std::numeric_limits<T>::epsilon() |
||
) |
Compresses input (dense) matrix expression into a sparse matrix.
This function sets this sparse matrix with dense input matrix. During the process, zero elements (less than epsilon
) will not be stored.
◆ MatrixCSR() [4/5]
CubbyFlow::MatrixCSR< T >::MatrixCSR | ( | const MatrixCSR< T > & | other | ) |
Copy constructor.
◆ MatrixCSR() [5/5]
|
noexcept |
Move constructor.
Member Function Documentation
◆ AbsMax()
T CubbyFlow::MatrixCSR< T >::AbsMax | ( | ) | const |
Returns absolute maximum among all elements.
◆ AbsMin()
T CubbyFlow::MatrixCSR< T >::AbsMin | ( | ) | const |
Returns absolute minimum among all elements.
◆ Add() [1/2]
MatrixCSR< T > CubbyFlow::MatrixCSR< T >::Add | ( | const T & | s | ) | const |
Returns this matrix + input scalar.
◆ Add() [2/2]
MatrixCSR< T > CubbyFlow::MatrixCSR< T >::Add | ( | const MatrixCSR< T > & | m | ) | const |
Returns this matrix + input matrix (element-wise).
◆ AddElement() [1/2]
void CubbyFlow::MatrixCSR< T >::AddElement | ( | size_t | i, |
size_t | j, | ||
const T & | value | ||
) |
Adds non-zero element to (i, j).
◆ AddElement() [2/2]
void CubbyFlow::MatrixCSR< T >::AddElement | ( | const Element & | element | ) |
Adds non-zero element.
◆ AddRow()
void CubbyFlow::MatrixCSR< T >::AddRow | ( | const NonZeroContainerType & | nonZeros, |
const IndexContainerType & | columnIndices | ||
) |
◆ Avg()
T CubbyFlow::MatrixCSR< T >::Avg | ( | ) | const |
Returns average of all elements.
◆ BinaryOp()
MatrixCSR<T> CubbyFlow::MatrixCSR< T >::BinaryOp | ( | const MatrixCSR< T > & | m, |
Op | op | ||
) | const |
◆ CastTo()
MatrixCSR< U > CubbyFlow::MatrixCSR< T >::CastTo | ( | ) | const |
Type-casts to different value-typed matrix.
◆ Clear()
void CubbyFlow::MatrixCSR< T >::Clear | ( | ) |
Clears the matrix and make it zero-dimensional.
◆ Cols()
size_t CubbyFlow::MatrixCSR< T >::Cols | ( | ) | const |
Returns number of columns of this matrix.
◆ ColumnIndex()
const size_t & CubbyFlow::MatrixCSR< T >::ColumnIndex | ( | size_t | i | ) | const |
Returns i-th column index.
◆ ColumnIndicesBegin() [1/2]
MatrixCSR< T >::IndexIterator CubbyFlow::MatrixCSR< T >::ColumnIndicesBegin | ( | ) |
Returns the begin iterator of the column indices.
◆ ColumnIndicesBegin() [2/2]
MatrixCSR< T >::ConstIndexIterator CubbyFlow::MatrixCSR< T >::ColumnIndicesBegin | ( | ) | const |
Returns the begin const iterator of the column indices.
◆ ColumnIndicesData()
const size_t * CubbyFlow::MatrixCSR< T >::ColumnIndicesData | ( | ) | const |
Returns constant pointer of the column indices data.
◆ ColumnIndicesEnd() [1/2]
MatrixCSR< T >::IndexIterator CubbyFlow::MatrixCSR< T >::ColumnIndicesEnd | ( | ) |
Returns the end iterator of the column indices.
◆ ColumnIndicesEnd() [2/2]
MatrixCSR< T >::ConstIndexIterator CubbyFlow::MatrixCSR< T >::ColumnIndicesEnd | ( | ) | const |
Returns the end const iterator of the column indices.
◆ Compress() [1/2]
void CubbyFlow::MatrixCSR< T >::Compress | ( | const std::initializer_list< std::initializer_list< T >> & | list, |
T | epsilon = std::numeric_limits<T>::epsilon() |
||
) |
Compresses given initializer list list
into a sparse matrix.
This function will fill the matrix with given initializer list list
such as
Note the initializer has 4x3 structure which will resize to 4x3 matrix. During the process, zero elements (less than epsilon
) will not be stored.
- Parameters
-
list Initializer list that should be copy to the new matrix.
◆ Compress() [2/2]
void CubbyFlow::MatrixCSR< T >::Compress | ( | const MatrixExpression< T, E > & | other, |
T | epsilon = std::numeric_limits<T>::epsilon() |
||
) |
Compresses input (dense) matrix expression into a sparse matrix.
This function sets this sparse matrix with dense input matrix. During the process, zero elements (less than epsilon
) will not be stored.
◆ Div()
MatrixCSR< T > CubbyFlow::MatrixCSR< T >::Div | ( | const T & | s | ) | const |
Returns this matrix / input scalar.
◆ IAdd() [1/2]
void CubbyFlow::MatrixCSR< T >::IAdd | ( | const T & | s | ) |
Adds input scalar to this matrix.
◆ IAdd() [2/2]
void CubbyFlow::MatrixCSR< T >::IAdd | ( | const MatrixCSR< T > & | m | ) |
Adds input matrix to this matrix (element-wise).
◆ IDiv()
void CubbyFlow::MatrixCSR< T >::IDiv | ( | const T & | s | ) |
Divides this matrix with input scalar.
◆ IMul() [1/2]
void CubbyFlow::MatrixCSR< T >::IMul | ( | const T & | s | ) |
Multiplies input scalar to this matrix.
◆ IMul() [2/2]
void CubbyFlow::MatrixCSR< T >::IMul | ( | const MatrixExpression< T, ME > & | m | ) |
Multiplies input matrix to this matrix.
◆ IsEqual()
bool CubbyFlow::MatrixCSR< T >::IsEqual | ( | const MatrixCSR< T > & | other | ) | const |
◆ IsSimilar()
bool CubbyFlow::MatrixCSR< T >::IsSimilar | ( | const MatrixCSR< T > & | other, |
double | tol = std::numeric_limits<double>::epsilon() |
||
) | const |
Returns true if this matrix is similar to the input matrix within the given tolerance.
◆ IsSquare()
bool CubbyFlow::MatrixCSR< T >::IsSquare | ( | ) | const |
Returns true if this matrix is a square matrix.
◆ ISub() [1/2]
void CubbyFlow::MatrixCSR< T >::ISub | ( | const T & | s | ) |
Subtracts input scalar from this matrix.
◆ ISub() [2/2]
void CubbyFlow::MatrixCSR< T >::ISub | ( | const MatrixCSR< T > & | m | ) |
Subtracts input matrix from this matrix (element-wise).
◆ MakeIdentity()
|
static |
Makes a m x m matrix with all diagonal elements to 1, and other elements to 0.
◆ Max()
T CubbyFlow::MatrixCSR< T >::Max | ( | ) | const |
Returns maximum among all elements.
◆ Min()
T CubbyFlow::MatrixCSR< T >::Min | ( | ) | const |
Returns minimum among all elements.
◆ Mul() [1/3]
MatrixCSR< T > CubbyFlow::MatrixCSR< T >::Mul | ( | const T & | s | ) | const |
Returns this matrix * input scalar.
◆ Mul() [2/3]
MatrixCSRVectorMul< T, VE > CubbyFlow::MatrixCSR< T >::Mul | ( | const VectorExpression< T, VE > & | v | ) | const |
Returns this matrix * input vector.
◆ Mul() [3/3]
MatrixCSRMatrixMul< T, ME > CubbyFlow::MatrixCSR< T >::Mul | ( | const MatrixExpression< T, ME > & | m | ) | const |
Returns this matrix * input matrix.
◆ NonZero() [1/2]
const T & CubbyFlow::MatrixCSR< T >::NonZero | ( | size_t | i | ) | const |
Returns i-th non-zero element.
◆ NonZero() [2/2]
T & CubbyFlow::MatrixCSR< T >::NonZero | ( | size_t | i | ) |
Returns i-th non-zero element.
◆ NonZeroBegin() [1/2]
MatrixCSR< T >::NonZeroIterator CubbyFlow::MatrixCSR< T >::NonZeroBegin | ( | ) |
Returns the begin iterator of the non-zero elements.
◆ NonZeroBegin() [2/2]
MatrixCSR< T >::ConstNonZeroIterator CubbyFlow::MatrixCSR< T >::NonZeroBegin | ( | ) | const |
Returns the begin const iterator of the non-zero elements.
◆ NonZeroData() [1/2]
T * CubbyFlow::MatrixCSR< T >::NonZeroData | ( | ) |
Returns pointer of the non-zero elements data.
◆ NonZeroData() [2/2]
const T * CubbyFlow::MatrixCSR< T >::NonZeroData | ( | ) | const |
Returns constant pointer of the non-zero elements data.
◆ NonZeroEnd() [1/2]
MatrixCSR< T >::NonZeroIterator CubbyFlow::MatrixCSR< T >::NonZeroEnd | ( | ) |
Returns the end iterator of the non-zero elements.
◆ NonZeroEnd() [2/2]
MatrixCSR< T >::ConstNonZeroIterator CubbyFlow::MatrixCSR< T >::NonZeroEnd | ( | ) | const |
Returns the end const iterator of the non-zero elements.
◆ NumberOfNonZeros()
size_t CubbyFlow::MatrixCSR< T >::NumberOfNonZeros | ( | ) | const |
Returns the number of non-zero elements.
◆ operator!=()
bool CubbyFlow::MatrixCSR< T >::operator!= | ( | const MatrixCSR< T > & | m | ) | const |
Returns true if is not equal to m.
◆ operator()()
T CubbyFlow::MatrixCSR< T >::operator() | ( | size_t | i, |
size_t | j | ||
) | const |
Returns (i,j) element.
◆ operator*=() [1/3]
MatrixCSR< T > & CubbyFlow::MatrixCSR< T >::operator*= | ( | const T & | s | ) |
Multiplication assignment with input scalar.
◆ operator*=() [2/3]
MatrixCSR& CubbyFlow::MatrixCSR< T >::operator*= | ( | const MatrixExpression< T, ME > & | m | ) |
Multiplication assignment with input matrix.
◆ operator*=() [3/3]
MatrixCSR<T>& CubbyFlow::MatrixCSR< T >::operator*= | ( | const MatrixExpression< T, ME > & | m | ) |
◆ operator+=() [1/2]
MatrixCSR< T > & CubbyFlow::MatrixCSR< T >::operator+= | ( | const T & | s | ) |
Addition assignment with input scalar.
◆ operator+=() [2/2]
MatrixCSR< T > & CubbyFlow::MatrixCSR< T >::operator+= | ( | const MatrixCSR< T > & | m | ) |
Addition assignment with input matrix (element-wise).
◆ operator-=() [1/2]
MatrixCSR< T > & CubbyFlow::MatrixCSR< T >::operator-= | ( | const T & | s | ) |
Subtraction assignment with input scalar.
◆ operator-=() [2/2]
MatrixCSR< T > & CubbyFlow::MatrixCSR< T >::operator-= | ( | const MatrixCSR< T > & | m | ) |
Subtraction assignment with input matrix (element-wise).
◆ operator/=()
MatrixCSR< T > & CubbyFlow::MatrixCSR< T >::operator/= | ( | const T & | s | ) |
Division assignment with input scalar.
◆ operator=() [1/4]
MatrixCSR& CubbyFlow::MatrixCSR< T >::operator= | ( | const E & | m | ) |
Compresses input (dense) matrix expression into a sparse matrix.
This function sets this sparse matrix with dense input matrix. During the process, zero elements (less than epsilon
) will not be stored.
◆ operator=() [2/4]
MatrixCSR< T > & CubbyFlow::MatrixCSR< T >::operator= | ( | const MatrixCSR< T > & | other | ) |
Copies to this matrix.
◆ operator=() [3/4]
|
noexcept |
Moves to this matrix.
◆ operator=() [4/4]
MatrixCSR<T>& CubbyFlow::MatrixCSR< T >::operator= | ( | const E & | m | ) |
◆ operator==()
bool CubbyFlow::MatrixCSR< T >::operator== | ( | const MatrixCSR< T > & | m | ) | const |
Returns true if is equal to m.
◆ RAdd() [1/2]
MatrixCSR< T > CubbyFlow::MatrixCSR< T >::RAdd | ( | const T & | s | ) | const |
Returns input scalar + this matrix.
◆ RAdd() [2/2]
MatrixCSR< T > CubbyFlow::MatrixCSR< T >::RAdd | ( | const MatrixCSR< T > & | m | ) | const |
Returns input matrix + this matrix (element-wise).
◆ RDiv()
MatrixCSR< T > CubbyFlow::MatrixCSR< T >::RDiv | ( | const T & | s | ) | const |
Returns input matrix / this scalar.
◆ Reserve()
void CubbyFlow::MatrixCSR< T >::Reserve | ( | size_t | rows, |
size_t | cols, | ||
size_t | numNonZeros | ||
) |
Reserves memory space of this matrix.
◆ RMul()
MatrixCSR< T > CubbyFlow::MatrixCSR< T >::RMul | ( | const T & | s | ) | const |
Returns input scalar * this matrix.
◆ RowPointer()
const size_t & CubbyFlow::MatrixCSR< T >::RowPointer | ( | size_t | i | ) | const |
Returns i-th row pointer.
◆ RowPointersBegin() [1/2]
MatrixCSR< T >::IndexIterator CubbyFlow::MatrixCSR< T >::RowPointersBegin | ( | ) |
Returns the begin iterator of the row pointers.
◆ RowPointersBegin() [2/2]
MatrixCSR< T >::ConstIndexIterator CubbyFlow::MatrixCSR< T >::RowPointersBegin | ( | ) | const |
Returns the begin const iterator of the row pointers.
◆ RowPointersData()
const size_t * CubbyFlow::MatrixCSR< T >::RowPointersData | ( | ) | const |
Returns constant pointer of the row pointers data.
◆ RowPointersEnd() [1/2]
MatrixCSR< T >::IndexIterator CubbyFlow::MatrixCSR< T >::RowPointersEnd | ( | ) |
Returns the end iterator of the row pointers.
◆ RowPointersEnd() [2/2]
MatrixCSR< T >::ConstIndexIterator CubbyFlow::MatrixCSR< T >::RowPointersEnd | ( | ) | const |
Returns the end const iterator of the row pointers.
◆ Rows()
size_t CubbyFlow::MatrixCSR< T >::Rows | ( | ) | const |
Returns number of rows of this matrix.
◆ RSub() [1/2]
MatrixCSR< T > CubbyFlow::MatrixCSR< T >::RSub | ( | const T & | s | ) | const |
Returns input scalar - this matrix.
◆ RSub() [2/2]
MatrixCSR< T > CubbyFlow::MatrixCSR< T >::RSub | ( | const MatrixCSR< T > & | m | ) | const |
Returns input matrix - this matrix (element-wise).
◆ Set() [1/2]
void CubbyFlow::MatrixCSR< T >::Set | ( | const T & | s | ) |
Sets whole matrix with input scalar.
◆ Set() [2/2]
void CubbyFlow::MatrixCSR< T >::Set | ( | const MatrixCSR< T > & | other | ) |
Copy from given sparse matrix.
◆ SetElement() [1/2]
void CubbyFlow::MatrixCSR< T >::SetElement | ( | size_t | i, |
size_t | j, | ||
const T & | value | ||
) |
Sets non-zero element to (i, j).
◆ SetElement() [2/2]
void CubbyFlow::MatrixCSR< T >::SetElement | ( | const Element & | element | ) |
Sets non-zero element.
◆ size()
Size2 CubbyFlow::MatrixCSR< T >::size | ( | ) | const |
Returns the size of this matrix.
◆ Sub() [1/2]
MatrixCSR< T > CubbyFlow::MatrixCSR< T >::Sub | ( | const T & | s | ) | const |
Returns this matrix - input scalar.
◆ Sub() [2/2]
MatrixCSR< T > CubbyFlow::MatrixCSR< T >::Sub | ( | const MatrixCSR< T > & | m | ) | const |
Returns this matrix - input matrix (element-wise).
◆ Sum()
T CubbyFlow::MatrixCSR< T >::Sum | ( | ) | const |
Returns sum of all elements.
◆ Trace()
T CubbyFlow::MatrixCSR< T >::Trace | ( | ) | const |
Returns sum of all diagonal elements.
- Warning
- Should be a square matrix.
The documentation for this class was generated from the following files:
- Core/Matrix/MatrixCSR.h
- Core/Matrix/MatrixCSR-Impl.h