Static-sized M x N matrix class. More...
#include <Core/Matrix/Matrix.h>
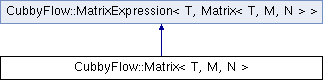
Public Types | |
using | ContainerType = std::array< T, M *N > |
using | Iterator = typename ContainerType::iterator |
using | ConstIterator = typename ContainerType::const_iterator |
Public Member Functions | |
Matrix () | |
template<typename... Params> | |
Matrix (Params... params) | |
Constructs matrix instance with parameters. More... | |
Matrix (const std::initializer_list< std::initializer_list< T >> &list) | |
Constructs a matrix with given initializer list list . More... | |
template<typename E > | |
Matrix (const MatrixExpression< T, E > &other) | |
Constructs a matrix with expression template. More... | |
Matrix (const Matrix &other) | |
Copy constructor. More... | |
void | Resize (size_t m, size_t n, const T &s=T(0)) |
Resizes to m x n matrix with initial value s . More... | |
void | Set (const T &s) |
Sets whole matrix with input scalar. More... | |
void | Set (const std::initializer_list< std::initializer_list< T >> &list) |
Sets a matrix with given initializer list list . More... | |
template<typename E > | |
void | Set (const MatrixExpression< T, E > &other) |
Copies from input matrix expression. More... | |
void | SetDiagonal (const T &s) |
Sets diagonal elements with input scalar. More... | |
void | SetOffDiagonal (const T &s) |
Sets off-diagonal elements with input scalar. More... | |
template<typename E > | |
void | SetRow (size_t i, const VectorExpression< T, E > &row) |
Sets i-th row with input vector. More... | |
template<typename E > | |
void | SetColumn (size_t j, const VectorExpression< T, E > &col) |
Sets j-th column with input vector. More... | |
template<typename E > | |
bool | IsEqual (const MatrixExpression< T, E > &other) const |
template<typename E > | |
bool | IsSimilar (const MatrixExpression< T, E > &other, double tol=std::numeric_limits< double >::epsilon()) const |
constexpr bool | IsSquare () const |
Returns true if this matrix is a square matrix. More... | |
constexpr Size2 | size () const |
Returns the size of this matrix. More... | |
constexpr size_t | Rows () const |
Returns number of rows of this matrix. More... | |
constexpr size_t | Cols () const |
Returns number of columns of this matrix. More... | |
T * | data () |
Returns data pointer of this matrix. More... | |
const T * | data () const |
Returns constant pointer of this matrix. More... | |
Iterator | begin () |
Returns the begin iterator of the matrix. More... | |
ConstIterator | begin () const |
Returns the begin const iterator of the matrix. More... | |
Iterator | end () |
Returns the end iterator of the matrix. More... | |
ConstIterator | end () const |
Returns the end const iterator of the matrix. More... | |
MatrixScalarAdd< T, Matrix > | Add (const T &s) const |
Returns this matrix + input scalar. More... | |
template<typename E > | |
MatrixAdd< T, Matrix, E > | Add (const E &m) const |
Returns this matrix + input matrix (element-wise). More... | |
MatrixScalarSub< T, Matrix > | Sub (const T &s) const |
Returns this matrix - input scalar. More... | |
template<typename E > | |
MatrixSub< T, Matrix, E > | Sub (const E &m) const |
Returns this matrix - input matrix (element-wise). More... | |
MatrixScalarMul< T, Matrix > | Mul (const T &s) const |
Returns this matrix * input scalar. More... | |
template<typename VE > | |
MatrixVectorMul< T, Matrix, VE > | Mul (const VectorExpression< T, VE > &v) const |
Returns this matrix * input vector. More... | |
template<size_t L> | |
MatrixMul< T, Matrix, Matrix< T, N, L > > | Mul (const Matrix< T, N, L > &m) const |
Returns this matrix * input matrix. More... | |
MatrixScalarDiv< T, Matrix > | Div (const T &s) const |
Returns this matrix / input scalar. More... | |
MatrixScalarAdd< T, Matrix > | RAdd (const T &s) const |
Returns input scalar + this matrix. More... | |
template<typename E > | |
MatrixAdd< T, Matrix, E > | RAdd (const E &m) const |
Returns input matrix + this matrix (element-wise). More... | |
MatrixScalarRSub< T, Matrix > | RSub (const T &s) const |
Returns input scalar - this matrix. More... | |
template<typename E > | |
MatrixSub< T, Matrix, E > | RSub (const E &m) const |
Returns input matrix - this matrix (element-wise). More... | |
MatrixScalarMul< T, Matrix > | RMul (const T &s) const |
Returns input scalar * this matrix. More... | |
template<size_t L> | |
MatrixMul< T, Matrix< T, N, L >, Matrix > | RMul (const Matrix< T, N, L > &m) const |
Returns input matrix * this matrix. More... | |
MatrixScalarRDiv< T, Matrix > | RDiv (const T &s) const |
Returns input matrix / this scalar. More... | |
void | IAdd (const T &s) |
Adds input scalar to this matrix. More... | |
template<typename E > | |
void | IAdd (const E &m) |
Adds input matrix to this matrix (element-wise). More... | |
void | ISub (const T &s) |
Subtracts input scalar from this matrix. More... | |
template<typename E > | |
void | ISub (const E &m) |
Subtracts input matrix from this matrix (element-wise). More... | |
void | IMul (const T &s) |
Multiplies input scalar to this matrix. More... | |
template<typename E > | |
void | IMul (const E &m) |
Multiplies input matrix to this matrix. More... | |
void | IDiv (const T &s) |
Divides this matrix with input scalar. More... | |
void | Transpose () |
Transposes this matrix. More... | |
void | Invert () |
Inverts this matrix. More... | |
T | Sum () const |
Returns sum of all elements. More... | |
T | Avg () const |
Returns average of all elements. More... | |
T | Min () const |
Returns minimum among all elements. More... | |
T | Max () const |
Returns maximum among all elements. More... | |
T | AbsMin () const |
Returns absolute minimum among all elements. More... | |
T | AbsMax () const |
Returns absolute maximum among all elements. More... | |
T | Trace () const |
T | Determinant () const |
Returns determinant of this matrix. More... | |
MatrixDiagonal< T, Matrix > | Diagonal () const |
Returns diagonal part of this matrix. More... | |
MatrixDiagonal< T, Matrix > | OffDiagonal () const |
Returns off-diagonal part of this matrix. More... | |
MatrixTriangular< T, Matrix > | StrictLowerTri () const |
Returns strictly lower triangle part of this matrix. More... | |
MatrixTriangular< T, Matrix > | StrictUpperTri () const |
Returns strictly upper triangle part of this matrix. More... | |
MatrixTriangular< T, Matrix > | LowerTri () const |
Returns lower triangle part of this matrix (including the diagonal). More... | |
MatrixTriangular< T, Matrix > | UpperTri () const |
Returns upper triangle part of this matrix (including the diagonal). More... | |
Matrix< T, N, M > | Transposed () const |
Returns transposed matrix. More... | |
Matrix | Inverse () const |
Returns inverse matrix. More... | |
template<typename U > | |
MatrixTypeCast< U, Matrix, T > | CastTo () const |
template<typename E > | |
Matrix & | operator= (const E &m) |
Assigns input matrix. More... | |
Matrix & | operator= (const Matrix &other) |
Copies to this matrix. More... | |
Matrix & | operator+= (const T &s) |
Addition assignment with input scalar. More... | |
template<typename E > | |
Matrix & | operator+= (const E &m) |
Addition assignment with input matrix (element-wise). More... | |
Matrix & | operator-= (const T &s) |
Subtraction assignment with input scalar. More... | |
template<typename E > | |
Matrix & | operator-= (const E &m) |
Subtraction assignment with input matrix (element-wise). More... | |
Matrix & | operator*= (const T &s) |
Multiplication assignment with input scalar. More... | |
template<typename E > | |
Matrix & | operator*= (const E &m) |
Multiplication assignment with input matrix. More... | |
Matrix & | operator/= (const T &s) |
Division assignment with input scalar. More... | |
T & | operator[] (size_t i) |
Returns reference of i-th element. More... | |
const T & | operator[] (size_t i) const |
Returns constant reference of i-th element. More... | |
T & | operator() (size_t i, size_t j) |
Returns reference of (i,j) element. More... | |
const T & | operator() (size_t i, size_t j) const |
Returns constant reference of (i,j) element. More... | |
template<typename E > | |
bool | operator== (const MatrixExpression< T, E > &m) const |
Returns true if is equal to m. More... | |
template<typename E > | |
bool | operator!= (const MatrixExpression< T, E > &m) const |
Returns true if is not equal to m. More... | |
template<typename Callback > | |
void | ForEach (Callback func) const |
Iterates the matrix and invoke given func for each index. More... | |
template<typename Callback > | |
void | ForEachIndex (Callback func) const |
Iterates the matrix and invoke given func for each index. More... | |
template<typename E > | |
MatrixAdd< T, Matrix< T, M, N >, E > | Add (const E &m) const |
template<typename E > | |
MatrixSub< T, Matrix< T, M, N >, E > | Sub (const E &m) const |
template<typename VE> | |
MatrixVectorMul< T, Matrix< T, M, N >, VE > | Mul (const VectorExpression< T, VE > &v) const |
template<size_t L> | |
MatrixMul< T, Matrix< T, M, N >, Matrix< T, N, L > > | Mul (const Matrix< T, N, L > &m) const |
template<typename E > | |
MatrixAdd< T, Matrix< T, M, N >, E > | RAdd (const E &m) const |
template<typename E > | |
MatrixSub< T, Matrix< T, M, N >, E > | RSub (const E &m) const |
template<size_t L> | |
MatrixMul< T, Matrix< T, N, L >, Matrix< T, M, N > > | RMul (const Matrix< T, N, L > &m) const |
template<typename U > | |
MatrixTypeCast< U, Matrix< T, M, N >, T > | CastTo () const |
template<typename E > | |
Matrix< T, M, N > & | operator= (const E &m) |
template<typename E > | |
Matrix< T, M, N > & | operator+= (const E &m) |
template<typename E > | |
Matrix< T, M, N > & | operator-= (const E &m) |
template<typename E > | |
Matrix< T, M, N > & | operator*= (const E &m) |
![]() | |
Size2 | size () const |
Size of the matrix. More... | |
size_t | Rows () const |
Number of rows. More... | |
size_t | Cols () const |
Number of columns. More... | |
const Matrix< T, M, N > & | operator() () const |
Returns actual implementation (the subclass). More... | |
Static Public Member Functions | |
static MatrixConstant< T > | MakeZero () |
Makes a M x N matrix with zeros. More... | |
static MatrixIdentity< T > | MakeIdentity () |
Makes a M x N matrix with all diagonal elements to 1, and other elements to 0. More... | |
Detailed Description
template<typename T, size_t M, size_t N>
class CubbyFlow::Matrix< T, M, N >
Static-sized M x N matrix class.
This class defines M x N row-major matrix data where its size is determined statically at compile time.
- Template Parameters
-
T - Real number type. M - Number of rows. N - Number of columns.
Member Typedef Documentation
◆ ConstIterator
using CubbyFlow::Matrix< T, M, N >::ConstIterator = typename ContainerType::const_iterator |
◆ ContainerType
using CubbyFlow::Matrix< T, M, N >::ContainerType = std::array<T, M * N> |
◆ Iterator
using CubbyFlow::Matrix< T, M, N >::Iterator = typename ContainerType::iterator |
Constructor & Destructor Documentation
◆ Matrix() [1/5]
CubbyFlow::Matrix< T, M, N >::Matrix | ( | ) |
Default constructor.
- Warning
- This constructor will create zero matrix.
◆ Matrix() [2/5]
|
explicit |
Constructs matrix instance with parameters.
◆ Matrix() [3/5]
CubbyFlow::Matrix< T, M, N >::Matrix | ( | const std::initializer_list< std::initializer_list< T >> & | list | ) |
Constructs a matrix with given initializer list list
.
This constructor will build a matrix with given initializer list list
such as
- Parameters
-
list Initializer list that should be copy to the new matrix.
◆ Matrix() [4/5]
CubbyFlow::Matrix< T, M, N >::Matrix | ( | const MatrixExpression< T, E > & | other | ) |
Constructs a matrix with expression template.
◆ Matrix() [5/5]
CubbyFlow::Matrix< T, M, N >::Matrix | ( | const Matrix< T, M, N > & | other | ) |
Copy constructor.
Member Function Documentation
◆ AbsMax()
T CubbyFlow::Matrix< T, M, N >::AbsMax | ( | ) | const |
Returns absolute maximum among all elements.
◆ AbsMin()
T CubbyFlow::Matrix< T, M, N >::AbsMin | ( | ) | const |
Returns absolute minimum among all elements.
◆ Add() [1/3]
MatrixScalarAdd< T, Matrix< T, M, N > > CubbyFlow::Matrix< T, M, N >::Add | ( | const T & | s | ) | const |
Returns this matrix + input scalar.
◆ Add() [2/3]
MatrixAdd<T, Matrix, E> CubbyFlow::Matrix< T, M, N >::Add | ( | const E & | m | ) | const |
Returns this matrix + input matrix (element-wise).
◆ Add() [3/3]
MatrixAdd<T, Matrix<T, M, N>, E> CubbyFlow::Matrix< T, M, N >::Add | ( | const E & | m | ) | const |
◆ Avg()
T CubbyFlow::Matrix< T, M, N >::Avg | ( | ) | const |
Returns average of all elements.
◆ begin() [1/2]
Matrix< T, M, N >::Iterator CubbyFlow::Matrix< T, M, N >::begin | ( | ) |
Returns the begin iterator of the matrix.
◆ begin() [2/2]
Matrix< T, M, N >::ConstIterator CubbyFlow::Matrix< T, M, N >::begin | ( | ) | const |
Returns the begin const iterator of the matrix.
◆ CastTo() [1/2]
MatrixTypeCast<U, Matrix, T> CubbyFlow::Matrix< T, M, N >::CastTo | ( | ) | const |
◆ CastTo() [2/2]
MatrixTypeCast<U, Matrix<T, M, N>, T> CubbyFlow::Matrix< T, M, N >::CastTo | ( | ) | const |
◆ Cols()
constexpr size_t CubbyFlow::Matrix< T, M, N >::Cols | ( | ) | const |
Returns number of columns of this matrix.
◆ data() [1/2]
T * CubbyFlow::Matrix< T, M, N >::data | ( | ) |
Returns data pointer of this matrix.
◆ data() [2/2]
const T * CubbyFlow::Matrix< T, M, N >::data | ( | ) | const |
Returns constant pointer of this matrix.
◆ Determinant()
T CubbyFlow::Matrix< T, M, N >::Determinant | ( | ) | const |
Returns determinant of this matrix.
◆ Diagonal()
MatrixDiagonal< T, Matrix< T, M, N > > CubbyFlow::Matrix< T, M, N >::Diagonal | ( | ) | const |
Returns diagonal part of this matrix.
◆ Div()
MatrixScalarDiv< T, Matrix< T, M, N > > CubbyFlow::Matrix< T, M, N >::Div | ( | const T & | s | ) | const |
Returns this matrix / input scalar.
◆ end() [1/2]
Matrix< T, M, N >::Iterator CubbyFlow::Matrix< T, M, N >::end | ( | ) |
Returns the end iterator of the matrix.
◆ end() [2/2]
Matrix< T, M, N >::ConstIterator CubbyFlow::Matrix< T, M, N >::end | ( | ) | const |
Returns the end const iterator of the matrix.
◆ ForEach()
void CubbyFlow::Matrix< T, M, N >::ForEach | ( | Callback | func | ) | const |
Iterates the matrix and invoke given func
for each index.
This function iterates the matrix elements and invoke the callback function func
. The callback function takes matrix's element as its input. The order of execution will be the same as the nested for-loop below:
Below is the sample usage:
◆ ForEachIndex()
void CubbyFlow::Matrix< T, M, N >::ForEachIndex | ( | Callback | func | ) | const |
Iterates the matrix and invoke given func
for each index.
This function iterates the matrix elements and invoke the callback function func
. The callback function takes two parameters which are the (i, j) indices of the matrix. The order of execution will be the same as the nested for-loop below:
Below is the sample usage:
◆ IAdd() [1/2]
void CubbyFlow::Matrix< T, M, N >::IAdd | ( | const T & | s | ) |
Adds input scalar to this matrix.
◆ IAdd() [2/2]
void CubbyFlow::Matrix< T, M, N >::IAdd | ( | const E & | m | ) |
Adds input matrix to this matrix (element-wise).
◆ IDiv()
void CubbyFlow::Matrix< T, M, N >::IDiv | ( | const T & | s | ) |
Divides this matrix with input scalar.
◆ IMul() [1/2]
void CubbyFlow::Matrix< T, M, N >::IMul | ( | const T & | s | ) |
Multiplies input scalar to this matrix.
◆ IMul() [2/2]
void CubbyFlow::Matrix< T, M, N >::IMul | ( | const E & | m | ) |
Multiplies input matrix to this matrix.
◆ Inverse()
Matrix< T, M, N > CubbyFlow::Matrix< T, M, N >::Inverse | ( | ) | const |
Returns inverse matrix.
◆ Invert()
void CubbyFlow::Matrix< T, M, N >::Invert | ( | ) |
Inverts this matrix.
This function computes the inverse using Gaussian elimination method.
◆ IsEqual()
bool CubbyFlow::Matrix< T, M, N >::IsEqual | ( | const MatrixExpression< T, E > & | other | ) | const |
◆ IsSimilar()
bool CubbyFlow::Matrix< T, M, N >::IsSimilar | ( | const MatrixExpression< T, E > & | other, |
double | tol = std::numeric_limits<double>::epsilon() |
||
) | const |
Returns true if this matrix is similar to the input matrix within the given tolerance.
◆ IsSquare()
constexpr bool CubbyFlow::Matrix< T, M, N >::IsSquare | ( | ) | const |
Returns true if this matrix is a square matrix.
◆ ISub() [1/2]
void CubbyFlow::Matrix< T, M, N >::ISub | ( | const T & | s | ) |
Subtracts input scalar from this matrix.
◆ ISub() [2/2]
void CubbyFlow::Matrix< T, M, N >::ISub | ( | const E & | m | ) |
Subtracts input matrix from this matrix (element-wise).
◆ LowerTri()
MatrixTriangular< T, Matrix< T, M, N > > CubbyFlow::Matrix< T, M, N >::LowerTri | ( | ) | const |
Returns lower triangle part of this matrix (including the diagonal).
◆ MakeIdentity()
|
static |
Makes a M x N matrix with all diagonal elements to 1, and other elements to 0.
◆ MakeZero()
|
static |
Makes a M x N matrix with zeros.
◆ Max()
T CubbyFlow::Matrix< T, M, N >::Max | ( | ) | const |
Returns maximum among all elements.
◆ Min()
T CubbyFlow::Matrix< T, M, N >::Min | ( | ) | const |
Returns minimum among all elements.
◆ Mul() [1/5]
MatrixScalarMul< T, Matrix< T, M, N > > CubbyFlow::Matrix< T, M, N >::Mul | ( | const T & | s | ) | const |
Returns this matrix * input scalar.
◆ Mul() [2/5]
MatrixVectorMul<T, Matrix, VE> CubbyFlow::Matrix< T, M, N >::Mul | ( | const VectorExpression< T, VE > & | v | ) | const |
Returns this matrix * input vector.
◆ Mul() [3/5]
MatrixMul<T, Matrix, Matrix<T, N, L> > CubbyFlow::Matrix< T, M, N >::Mul | ( | const Matrix< T, N, L > & | m | ) | const |
Returns this matrix * input matrix.
◆ Mul() [4/5]
MatrixVectorMul<T, Matrix<T, M, N>, VE> CubbyFlow::Matrix< T, M, N >::Mul | ( | const VectorExpression< T, VE > & | v | ) | const |
◆ Mul() [5/5]
MatrixMul<T, Matrix<T, M, N>, Matrix<T, N, L> > CubbyFlow::Matrix< T, M, N >::Mul | ( | const Matrix< T, N, L > & | m | ) | const |
◆ OffDiagonal()
MatrixDiagonal< T, Matrix< T, M, N > > CubbyFlow::Matrix< T, M, N >::OffDiagonal | ( | ) | const |
Returns off-diagonal part of this matrix.
◆ operator!=()
bool CubbyFlow::Matrix< T, M, N >::operator!= | ( | const MatrixExpression< T, E > & | m | ) | const |
Returns true if is not equal to m.
◆ operator()() [1/2]
T & CubbyFlow::Matrix< T, M, N >::operator() | ( | size_t | i, |
size_t | j | ||
) |
Returns reference of (i,j) element.
◆ operator()() [2/2]
const T & CubbyFlow::Matrix< T, M, N >::operator() | ( | size_t | i, |
size_t | j | ||
) | const |
Returns constant reference of (i,j) element.
◆ operator*=() [1/3]
Matrix< T, M, N > & CubbyFlow::Matrix< T, M, N >::operator*= | ( | const T & | s | ) |
Multiplication assignment with input scalar.
◆ operator*=() [2/3]
Matrix& CubbyFlow::Matrix< T, M, N >::operator*= | ( | const E & | m | ) |
Multiplication assignment with input matrix.
◆ operator*=() [3/3]
Matrix<T, M, N>& CubbyFlow::Matrix< T, M, N >::operator*= | ( | const E & | m | ) |
◆ operator+=() [1/3]
Matrix< T, M, N > & CubbyFlow::Matrix< T, M, N >::operator+= | ( | const T & | s | ) |
Addition assignment with input scalar.
◆ operator+=() [2/3]
Matrix& CubbyFlow::Matrix< T, M, N >::operator+= | ( | const E & | m | ) |
Addition assignment with input matrix (element-wise).
◆ operator+=() [3/3]
Matrix<T, M, N>& CubbyFlow::Matrix< T, M, N >::operator+= | ( | const E & | m | ) |
◆ operator-=() [1/3]
Matrix< T, M, N > & CubbyFlow::Matrix< T, M, N >::operator-= | ( | const T & | s | ) |
Subtraction assignment with input scalar.
◆ operator-=() [2/3]
Matrix& CubbyFlow::Matrix< T, M, N >::operator-= | ( | const E & | m | ) |
Subtraction assignment with input matrix (element-wise).
◆ operator-=() [3/3]
Matrix<T, M, N>& CubbyFlow::Matrix< T, M, N >::operator-= | ( | const E & | m | ) |
◆ operator/=()
Matrix< T, M, N > & CubbyFlow::Matrix< T, M, N >::operator/= | ( | const T & | s | ) |
Division assignment with input scalar.
◆ operator=() [1/3]
Matrix& CubbyFlow::Matrix< T, M, N >::operator= | ( | const E & | m | ) |
Assigns input matrix.
◆ operator=() [2/3]
Matrix< T, M, N > & CubbyFlow::Matrix< T, M, N >::operator= | ( | const Matrix< T, M, N > & | other | ) |
Copies to this matrix.
◆ operator=() [3/3]
Matrix<T, M, N>& CubbyFlow::Matrix< T, M, N >::operator= | ( | const E & | m | ) |
◆ operator==()
bool CubbyFlow::Matrix< T, M, N >::operator== | ( | const MatrixExpression< T, E > & | m | ) | const |
Returns true if is equal to m.
◆ operator[]() [1/2]
T & CubbyFlow::Matrix< T, M, N >::operator[] | ( | size_t | i | ) |
Returns reference of i-th element.
◆ operator[]() [2/2]
const T & CubbyFlow::Matrix< T, M, N >::operator[] | ( | size_t | i | ) | const |
Returns constant reference of i-th element.
◆ RAdd() [1/3]
MatrixScalarAdd< T, Matrix< T, M, N > > CubbyFlow::Matrix< T, M, N >::RAdd | ( | const T & | s | ) | const |
Returns input scalar + this matrix.
◆ RAdd() [2/3]
MatrixAdd<T, Matrix, E> CubbyFlow::Matrix< T, M, N >::RAdd | ( | const E & | m | ) | const |
Returns input matrix + this matrix (element-wise).
◆ RAdd() [3/3]
MatrixAdd<T, Matrix<T, M, N>, E> CubbyFlow::Matrix< T, M, N >::RAdd | ( | const E & | m | ) | const |
◆ RDiv()
MatrixScalarRDiv< T, Matrix< T, M, N > > CubbyFlow::Matrix< T, M, N >::RDiv | ( | const T & | s | ) | const |
Returns input matrix / this scalar.
◆ Resize()
void CubbyFlow::Matrix< T, M, N >::Resize | ( | size_t | m, |
size_t | n, | ||
const T & | s = T(0) |
||
) |
Resizes to m x n matrix with initial value s
.
◆ RMul() [1/3]
MatrixScalarMul< T, Matrix< T, M, N > > CubbyFlow::Matrix< T, M, N >::RMul | ( | const T & | s | ) | const |
Returns input scalar * this matrix.
◆ RMul() [2/3]
MatrixMul<T, Matrix<T, N, L>, Matrix> CubbyFlow::Matrix< T, M, N >::RMul | ( | const Matrix< T, N, L > & | m | ) | const |
Returns input matrix * this matrix.
◆ RMul() [3/3]
MatrixMul<T, Matrix<T, N, L>, Matrix<T, M, N> > CubbyFlow::Matrix< T, M, N >::RMul | ( | const Matrix< T, N, L > & | m | ) | const |
◆ Rows()
constexpr size_t CubbyFlow::Matrix< T, M, N >::Rows | ( | ) | const |
Returns number of rows of this matrix.
◆ RSub() [1/3]
MatrixScalarRSub< T, Matrix< T, M, N > > CubbyFlow::Matrix< T, M, N >::RSub | ( | const T & | s | ) | const |
Returns input scalar - this matrix.
◆ RSub() [2/3]
MatrixSub<T, Matrix, E> CubbyFlow::Matrix< T, M, N >::RSub | ( | const E & | m | ) | const |
Returns input matrix - this matrix (element-wise).
◆ RSub() [3/3]
MatrixSub<T, Matrix<T, M, N>, E> CubbyFlow::Matrix< T, M, N >::RSub | ( | const E & | m | ) | const |
◆ Set() [1/3]
void CubbyFlow::Matrix< T, M, N >::Set | ( | const T & | s | ) |
Sets whole matrix with input scalar.
◆ Set() [2/3]
void CubbyFlow::Matrix< T, M, N >::Set | ( | const std::initializer_list< std::initializer_list< T >> & | list | ) |
Sets a matrix with given initializer list list
.
This function will fill the matrix with given initializer list list
such as
- Parameters
-
list Initializer list that should be copy to the new matrix.
◆ Set() [3/3]
void CubbyFlow::Matrix< T, M, N >::Set | ( | const MatrixExpression< T, E > & | other | ) |
Copies from input matrix expression.
◆ SetColumn()
void CubbyFlow::Matrix< T, M, N >::SetColumn | ( | size_t | j, |
const VectorExpression< T, E > & | col | ||
) |
Sets j-th column with input vector.
◆ SetDiagonal()
void CubbyFlow::Matrix< T, M, N >::SetDiagonal | ( | const T & | s | ) |
Sets diagonal elements with input scalar.
◆ SetOffDiagonal()
void CubbyFlow::Matrix< T, M, N >::SetOffDiagonal | ( | const T & | s | ) |
Sets off-diagonal elements with input scalar.
◆ SetRow()
void CubbyFlow::Matrix< T, M, N >::SetRow | ( | size_t | i, |
const VectorExpression< T, E > & | row | ||
) |
Sets i-th row with input vector.
◆ size()
constexpr Size2 CubbyFlow::Matrix< T, M, N >::size | ( | ) | const |
Returns the size of this matrix.
◆ StrictLowerTri()
MatrixTriangular< T, Matrix< T, M, N > > CubbyFlow::Matrix< T, M, N >::StrictLowerTri | ( | ) | const |
Returns strictly lower triangle part of this matrix.
◆ StrictUpperTri()
MatrixTriangular< T, Matrix< T, M, N > > CubbyFlow::Matrix< T, M, N >::StrictUpperTri | ( | ) | const |
Returns strictly upper triangle part of this matrix.
◆ Sub() [1/3]
MatrixScalarSub< T, Matrix< T, M, N > > CubbyFlow::Matrix< T, M, N >::Sub | ( | const T & | s | ) | const |
Returns this matrix - input scalar.
◆ Sub() [2/3]
MatrixSub<T, Matrix, E> CubbyFlow::Matrix< T, M, N >::Sub | ( | const E & | m | ) | const |
Returns this matrix - input matrix (element-wise).
◆ Sub() [3/3]
MatrixSub<T, Matrix<T, M, N>, E> CubbyFlow::Matrix< T, M, N >::Sub | ( | const E & | m | ) | const |
◆ Sum()
T CubbyFlow::Matrix< T, M, N >::Sum | ( | ) | const |
Returns sum of all elements.
◆ Trace()
T CubbyFlow::Matrix< T, M, N >::Trace | ( | ) | const |
Returns sum of all diagonal elements.
- Warning
- Should be a square matrix.
◆ Transpose()
void CubbyFlow::Matrix< T, M, N >::Transpose | ( | ) |
Transposes this matrix.
◆ Transposed()
Matrix< T, N, M > CubbyFlow::Matrix< T, M, N >::Transposed | ( | ) | const |
Returns transposed matrix.
◆ UpperTri()
MatrixTriangular< T, Matrix< T, M, N > > CubbyFlow::Matrix< T, M, N >::UpperTri | ( | ) | const |
Returns upper triangle part of this matrix (including the diagonal).
The documentation for this class was generated from the following files:
- Core/Matrix/Matrix.h
- Core/Matrix/Matrix-Impl.h