2-D grid-based backward Euler diffusion solver. More...
#include <Core/Solver/Grid/GridBackwardEulerDiffusionSolver2.h>
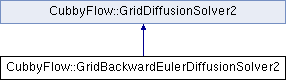
Public Types | |
enum | BoundaryType { BoundaryType::Dirichlet, BoundaryType::Neumann } |
Public Member Functions | |
GridBackwardEulerDiffusionSolver2 (BoundaryType boundaryType=BoundaryType::Neumann) | |
Constructs the solver with given boundary type. More... | |
void | Solve (const ScalarGrid2 &source, double diffusionCoefficient, double timeIntervalInSeconds, ScalarGrid2 *dest, const ScalarField2 &boundarySDF=ConstantScalarField2(std::numeric_limits< double >::max()), const ScalarField2 &fluidSDF=ConstantScalarField2(-std::numeric_limits< double >::max())) override |
void | Solve (const CollocatedVectorGrid2 &source, double diffusionCoefficient, double timeIntervalInSeconds, CollocatedVectorGrid2 *dest, const ScalarField2 &boundarySDF=ConstantScalarField2(std::numeric_limits< double >::max()), const ScalarField2 &fluidSDF=ConstantScalarField2(-std::numeric_limits< double >::max())) override |
void | Solve (const FaceCenteredGrid2 &source, double diffusionCoefficient, double timeIntervalInSeconds, FaceCenteredGrid2 *dest, const ScalarField2 &boundarySDF=ConstantScalarField2(std::numeric_limits< double >::max()), const ScalarField2 &fluidSDF=ConstantScalarField2(-std::numeric_limits< double >::max())) override |
void | SetLinearSystemSolver (const FDMLinearSystemSolver2Ptr &solver) |
Sets the linear system solver for this diffusion solver. More... | |
![]() | |
GridDiffusionSolver2 () | |
Default constructor. More... | |
virtual | ~GridDiffusionSolver2 () |
Default destructor. More... | |
Detailed Description
2-D grid-based backward Euler diffusion solver.
This class implements 2-D grid-based forward Euler diffusion solver using second-order central differencing spatially. Since the method is following the implicit time-integration (i.e. backward Euler), larger time interval or diffusion coefficient can be used without breaking the result. Note, higher values for those parameters will still impact the accuracy of the result. To solve the backward Euler method, a linear system solver is used and incomplete Cholesky conjugate gradient method is used by default.
Member Enumeration Documentation
◆ BoundaryType
Constructor & Destructor Documentation
◆ GridBackwardEulerDiffusionSolver2()
|
explicit |
Constructs the solver with given boundary type.
Member Function Documentation
◆ SetLinearSystemSolver()
void CubbyFlow::GridBackwardEulerDiffusionSolver2::SetLinearSystemSolver | ( | const FDMLinearSystemSolver2Ptr & | solver | ) |
Sets the linear system solver for this diffusion solver.
◆ Solve() [1/3]
|
overridevirtual |
Solves diffusion equation for a scalar field.
- Parameters
-
source Input scalar field. diffusionCoefficient Amount of diffusion. timeIntervalInSeconds Small time-interval that diffusion occur. dest Output scalar field. boundarySDF Shape of the solid boundary that is empty by default. fluidSDF Shape of the fluid boundary that is full by default.
Implements CubbyFlow::GridDiffusionSolver2.
◆ Solve() [2/3]
|
overridevirtual |
Solves diffusion equation for a collocated vector field.
- Parameters
-
source Input collocated vector field. diffusionCoefficient Amount of diffusion. timeIntervalInSeconds Small time-interval that diffusion occur. dest Output collocated vector field. boundarySDF Shape of the solid boundary that is empty by default. fluidSDF Shape of the fluid boundary that is full by default.
Implements CubbyFlow::GridDiffusionSolver2.
◆ Solve() [3/3]
|
overridevirtual |
Solves diffusion equation for a face-centered vector field.
- Parameters
-
source Input face-centered vector field. diffusionCoefficient Amount of diffusion. timeIntervalInSeconds Small time-interval that diffusion occur. dest Output face-centered vector field.
boundarySDF Shape of the solid boundary that is empty by default. fluidSDF Shape of the fluid boundary that is full by default.
Implements CubbyFlow::GridDiffusionSolver2.
The documentation for this class was generated from the following file:
- Core/Solver/Grid/GridBackwardEulerDiffusionSolver2.h