2-D finite difference-type linear system solver using Gauss-Seidel method. More...
#include <Core/Solver/FDM/FDMGaussSeidelSolver2.h>
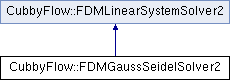
Public Member Functions | |
FDMGaussSeidelSolver2 (unsigned int maxNumberOfIterations, unsigned int residualCheckInterval, double tolerance, double sorFactor=1.0, bool useRedBlackOrdering=false) | |
Constructs the solver with given parameters. More... | |
bool | Solve (FDMLinearSystem2 *system) override |
Solves the given linear system. More... | |
bool | SolveCompressed (FDMCompressedLinearSystem2 *system) override |
Solves the given compressed linear system. More... | |
unsigned int | GetMaxNumberOfIterations () const |
Returns the max number of Gauss-Seidel iterations. More... | |
unsigned int | GetLastNumberOfIterations () const |
Returns the last number of Gauss-Seidel iterations the solver made. More... | |
double | GetTolerance () const |
Returns the max residual tolerance for the Gauss-Seidel method. More... | |
double | GetLastResidual () const |
Returns the last residual after the Gauss-Seidel iterations. More... | |
double | GetSORFactor () const |
Returns the SOR (Successive Over Relaxation) factor. More... | |
bool | GetUseRedBlackOrdering () const |
Returns true if red-black ordering is enabled. More... | |
![]() | |
virtual | ~FDMLinearSystemSolver2 ()=default |
Static Public Member Functions | |
static void | Relax (const FDMMatrix2 &A, const FDMVector2 &b, double sorFactor, FDMVector2 *x) |
Performs single natural Gauss-Seidel relaxation step. More... | |
static void | Relax (const MatrixCSRD &A, const VectorND &b, double sorFactor, VectorND *x) |
Performs single natural Gauss-Seidel relaxation step for compressed sys. More... | |
static void | RelaxRedBlack (const FDMMatrix2 &A, const FDMVector2 &b, double sorFactor, FDMVector2 *x) |
Performs single Red-Black Gauss-Seidel relaxation step. More... | |
Detailed Description
2-D finite difference-type linear system solver using Gauss-Seidel method.
Constructor & Destructor Documentation
◆ FDMGaussSeidelSolver2()
CubbyFlow::FDMGaussSeidelSolver2::FDMGaussSeidelSolver2 | ( | unsigned int | maxNumberOfIterations, |
unsigned int | residualCheckInterval, | ||
double | tolerance, | ||
double | sorFactor = 1.0 , |
||
bool | useRedBlackOrdering = false |
||
) |
Constructs the solver with given parameters.
Member Function Documentation
◆ GetLastNumberOfIterations()
unsigned int CubbyFlow::FDMGaussSeidelSolver2::GetLastNumberOfIterations | ( | ) | const |
Returns the last number of Gauss-Seidel iterations the solver made.
◆ GetLastResidual()
double CubbyFlow::FDMGaussSeidelSolver2::GetLastResidual | ( | ) | const |
Returns the last residual after the Gauss-Seidel iterations.
◆ GetMaxNumberOfIterations()
unsigned int CubbyFlow::FDMGaussSeidelSolver2::GetMaxNumberOfIterations | ( | ) | const |
Returns the max number of Gauss-Seidel iterations.
◆ GetSORFactor()
double CubbyFlow::FDMGaussSeidelSolver2::GetSORFactor | ( | ) | const |
Returns the SOR (Successive Over Relaxation) factor.
◆ GetTolerance()
double CubbyFlow::FDMGaussSeidelSolver2::GetTolerance | ( | ) | const |
Returns the max residual tolerance for the Gauss-Seidel method.
◆ GetUseRedBlackOrdering()
bool CubbyFlow::FDMGaussSeidelSolver2::GetUseRedBlackOrdering | ( | ) | const |
Returns true if red-black ordering is enabled.
◆ Relax() [1/2]
|
static |
Performs single natural Gauss-Seidel relaxation step.
◆ Relax() [2/2]
|
static |
Performs single natural Gauss-Seidel relaxation step for compressed sys.
◆ RelaxRedBlack()
|
static |
Performs single Red-Black Gauss-Seidel relaxation step.
◆ Solve()
|
overridevirtual |
Solves the given linear system.
Implements CubbyFlow::FDMLinearSystemSolver2.
◆ SolveCompressed()
|
overridevirtual |
Solves the given compressed linear system.
Reimplemented from CubbyFlow::FDMLinearSystemSolver2.
The documentation for this class was generated from the following file:
- Core/Solver/FDM/FDMGaussSeidelSolver2.h