Abstract base class for generic collider object. More...
#include <Core/Collider/Collider2.h>
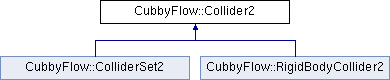
Classes | |
struct | ColliderQueryResult |
Internal query result structure. More... | |
Public Types | |
using | OnBeginUpdateCallback = std::function< void(Collider2 *, double, double)> |
Callback function type for update calls. More... | |
Public Member Functions | |
Collider2 () | |
Default constructor. More... | |
virtual | ~Collider2 () |
Default destructor. More... | |
virtual Vector2D | VelocityAt (const Vector2D &point) const =0 |
Returns the velocity of the collider at given point . More... | |
void | ResolveCollision (double radius, double restitutionCoefficient, Vector2D *position, Vector2D *velocity) |
double | GetFrictionCoefficient () const |
Returns friction coefficient. More... | |
void | SetFrictionCoefficient (double newFrictionCoeffient) |
Sets the friction coefficient. More... | |
const Surface2Ptr & | GetSurface () const |
Returns the surface instance. More... | |
void | Update (double currentTimeInSeconds, double timeIntervalInSeconds) |
Updates the collider state. More... | |
void | SetOnBeginUpdateCallback (const OnBeginUpdateCallback &callback) |
Sets the callback function to be called when Collider2::update function is invoked. More... | |
Protected Member Functions | |
void | SetSurface (const Surface2Ptr &newSurface) |
Assigns the surface instance from the subclass. More... | |
void | GetClosestPoint (const Surface2Ptr &surface, const Vector2D &queryPoint, ColliderQueryResult *result) const |
Outputs closest point's information. More... | |
bool | IsPenetrating (const ColliderQueryResult &colliderPoint, const Vector2D &position, double radius) |
Returns true if given point is in the opposite side of the surface. More... | |
Detailed Description
Abstract base class for generic collider object.
This class contains basic interfaces for colliders. Most of the functionalities are implemented within this class, except the member function Collider2::VelocityAt. This class also let the subclasses to provide a Surface2 instance to define collider surface using Collider2::SetSurface function.
Member Typedef Documentation
◆ OnBeginUpdateCallback
using CubbyFlow::Collider2::OnBeginUpdateCallback = std::function<void(Collider2*, double, double)> |
Callback function type for update calls.
This type of callback function will take the collider pointer, current time, and time interval in seconds.
Constructor & Destructor Documentation
◆ Collider2()
CubbyFlow::Collider2::Collider2 | ( | ) |
Default constructor.
◆ ~Collider2()
|
virtual |
Default destructor.
Member Function Documentation
◆ GetClosestPoint()
|
protected |
Outputs closest point's information.
◆ GetFrictionCoefficient()
double CubbyFlow::Collider2::GetFrictionCoefficient | ( | ) | const |
Returns friction coefficient.
◆ GetSurface()
const Surface2Ptr& CubbyFlow::Collider2::GetSurface | ( | ) | const |
Returns the surface instance.
◆ IsPenetrating()
|
protected |
Returns true if given point is in the opposite side of the surface.
◆ ResolveCollision()
void CubbyFlow::Collider2::ResolveCollision | ( | double | radius, |
double | restitutionCoefficient, | ||
Vector2D * | position, | ||
Vector2D * | velocity | ||
) |
Resolves collision for given point.
- Parameters
-
radius Radius of the colliding point. restitutionCoefficient Defines the restitution effect. position Input and output position of the point. velocity Input and output velocity of the point.
◆ SetFrictionCoefficient()
void CubbyFlow::Collider2::SetFrictionCoefficient | ( | double | newFrictionCoeffient | ) |
Sets the friction coefficient.
This function assigns the friction coefficient to the collider. Any negative inputs will be clamped to zero.
◆ SetOnBeginUpdateCallback()
void CubbyFlow::Collider2::SetOnBeginUpdateCallback | ( | const OnBeginUpdateCallback & | callback | ) |
Sets the callback function to be called when Collider2::update function is invoked.
The callback function takes current simulation time in seconds unit. Use this callback to track any motion or state changes related to this collider.
- Parameters
-
[in] callback The callback function.
◆ SetSurface()
|
protected |
Assigns the surface instance from the subclass.
◆ Update()
void CubbyFlow::Collider2::Update | ( | double | currentTimeInSeconds, |
double | timeIntervalInSeconds | ||
) |
Updates the collider state.
◆ VelocityAt()
Returns the velocity of the collider at given point
.
Implemented in CubbyFlow::RigidBodyCollider2, and CubbyFlow::ColliderSet2.
The documentation for this class was generated from the following file:
- Core/Collider/Collider2.h